Automating User Interaction: Simulating Typing in Automation Testing and Web Scraping
By Kainat Chaudhary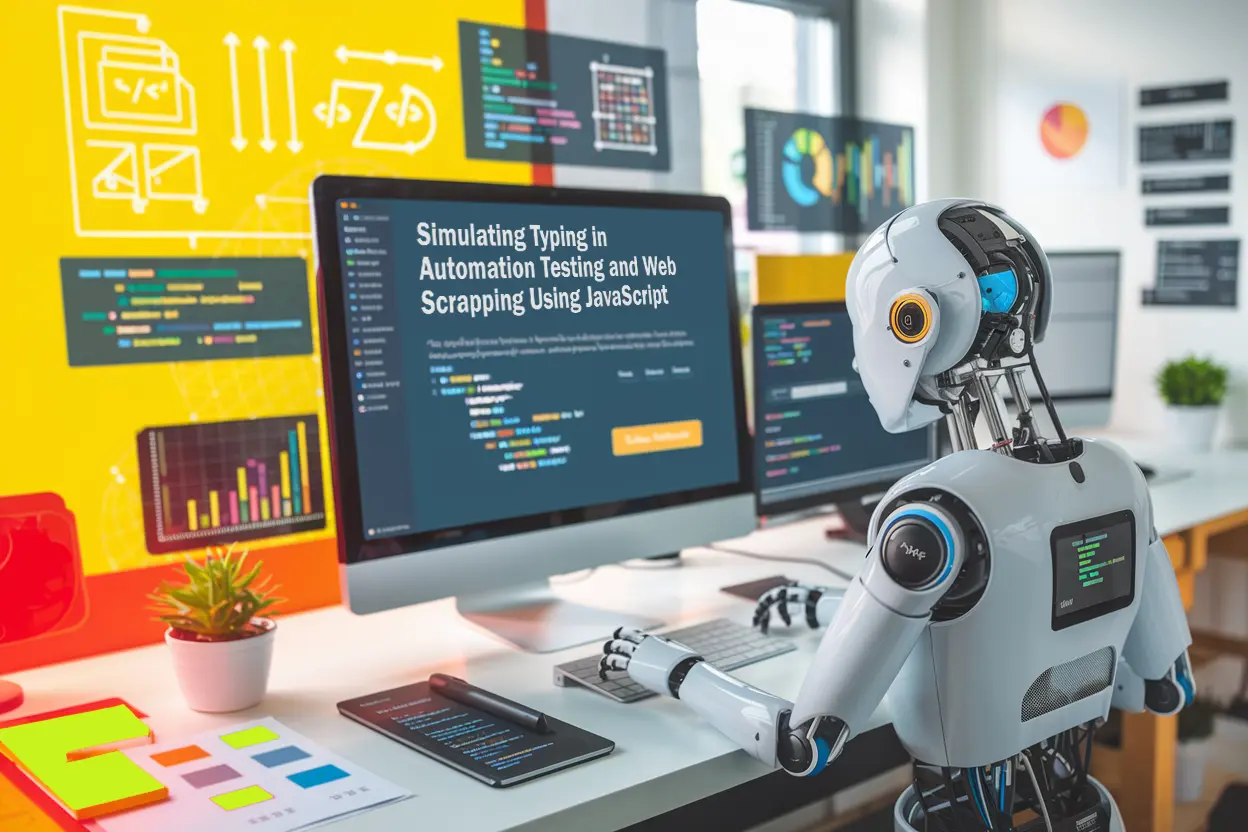
Introduction
Automation testing and web scraping are crucial aspects of modern web development and data extraction. Automation testing ensures that your web applications function as intended, while web scraping allows you to extract valuable data from websites efficiently. In both scenarios, interacting with web elements—like input fields—becomes essential. Sometimes, simply setting an input field's value directly in code doesn't trigger the necessary events that a real user interaction would. This is where simulating user interactions, like typing, becomes vital.
In this article, we will explore a practical use case of a JavaScript function that simulates typing into an input field. We'll walk through how and why this approach is used in automation testing and web scraping.
The following JavaScript functions help simulate typing into an input field, making it appear as though a real user is interacting with the page:
function sleep(ms) {
return new Promise((resolve) => setTimeout(resolve, ms));
}
function setNativeValue(element, value) {
const valueSetter = Object.getOwnPropertyDescriptor(element, 'value').set;
const prototype = Object.getPrototypeOf(element);
const prototypeValueSetter = Object.getOwnPropertyDescriptor(prototype, 'value').set;
if (valueSetter && valueSetter !== prototypeValueSetter) {
prototypeValueSetter.call(element, value);
} else {
valueSetter.call(element, value);
}
element.dispatchEvent(new Event('input', { bubbles: true }));
}
async function simulateTyping(element, text) {
element.focus();
setNativeValue(element, '');
for (let i = 0; i < text.length; i++) {
setNativeValue(element, element.value + text[i]);
element.dispatchEvent(new KeyboardEvent('keydown', { bubbles: true, cancelable: true }));
element.dispatchEvent(new KeyboardEvent('keypress', { bubbles: true, cancelable: true }));
element.dispatchEvent(new KeyboardEvent('keyup', { bubbles: true, cancelable: true }));
element.dispatchEvent(new Event('input', { bubbles: true }));
await sleep(50 + Math.random() * 100);
}
element.dispatchEvent(new Event('change', { bubbles: true }));
element.dispatchEvent(new Event('blur', { bubbles: true }));
}
How It Works
- sleep(ms): This is a utility function that pauses the execution for a specified number of milliseconds. It is used to simulate the natural pauses between keystrokes that occur during typing.
- setNativeValue(element, value): This function sets the value of an input field in a way that mimics the native behavior of typing. It directly modifies the value property of the input element and then dispatches an input event to notify the browser and any event listeners that the value has changed.
- simulateTyping(element, text): This is the main function that simulates typing. It: Focuses on the input field. Clears any existing text. Iterates over each character in the provided text string, appending it to the input field's value. Dispatches keydown, keypress, keyup, input, and change events to simulate the natural sequence of events that occur when a user types. Uses the sleep function to introduce random pauses between keystrokes, making the typing appear more human-like.
Use Cases
1. Automation Testing: When testing web applications, especially forms, it’s crucial to simulate user behavior accurately. For instance, many frameworks may not trigger the same events as a real user would when setting the value of an input field programmatically. This can lead to scenarios where the application behaves differently during testing compared to a real-world usage.
Using simulateTyping ensures that all associated events (like input, change, blur, etc.) are triggered in the correct order, allowing for more accurate and reliable test outcomes. This method is particularly useful in End-to-End (E2E) testing with frameworks like Selenium or Cypress, where you want to mimic user interactions closely.
2. Web Scraping Web scraping often requires filling out and submitting forms to access certain data. Some websites use JavaScript to detect if the input is being typed by a real user or filled automatically. Directly setting the value may bypass these checks, causing the website to behave differently, potentially blocking the scraper.
By using simulateTyping, the scraper mimics human interaction, reducing the chance of being detected and increasing the likelihood of successful data extraction. This method can be particularly useful when scraping data from websites that implement anti-bot measures or require user interaction to load dynamic content.
Why Use This Approach?
- Accurate Simulation: Simulating typing events ensures that the input field behaves as if a real user is interacting with it, triggering all necessary events and validations.
- Reliable Testing: In automation testing, this approach leads to more reliable and consistent test results by mimicking real user behavior.
- Anti-Bot Measures: When web scraping, simulating typing can help bypass anti-bot measures and access data that requires user interaction.
- Dynamic Content: Websites with dynamic content that loads based on user input may require simulated typing to trigger the necessary updates.
Conclusion
Simulating user input by mimicking typing is a powerful technique in both automation testing and web scraping. It ensures that your interactions with web elements are as close to real-world usage as possible, leading to more reliable and accurate results. By using the simulateTyping function, you can automate complex user interactions, overcome anti-bot measures, and enhance the robustness of your tests and scrapers.
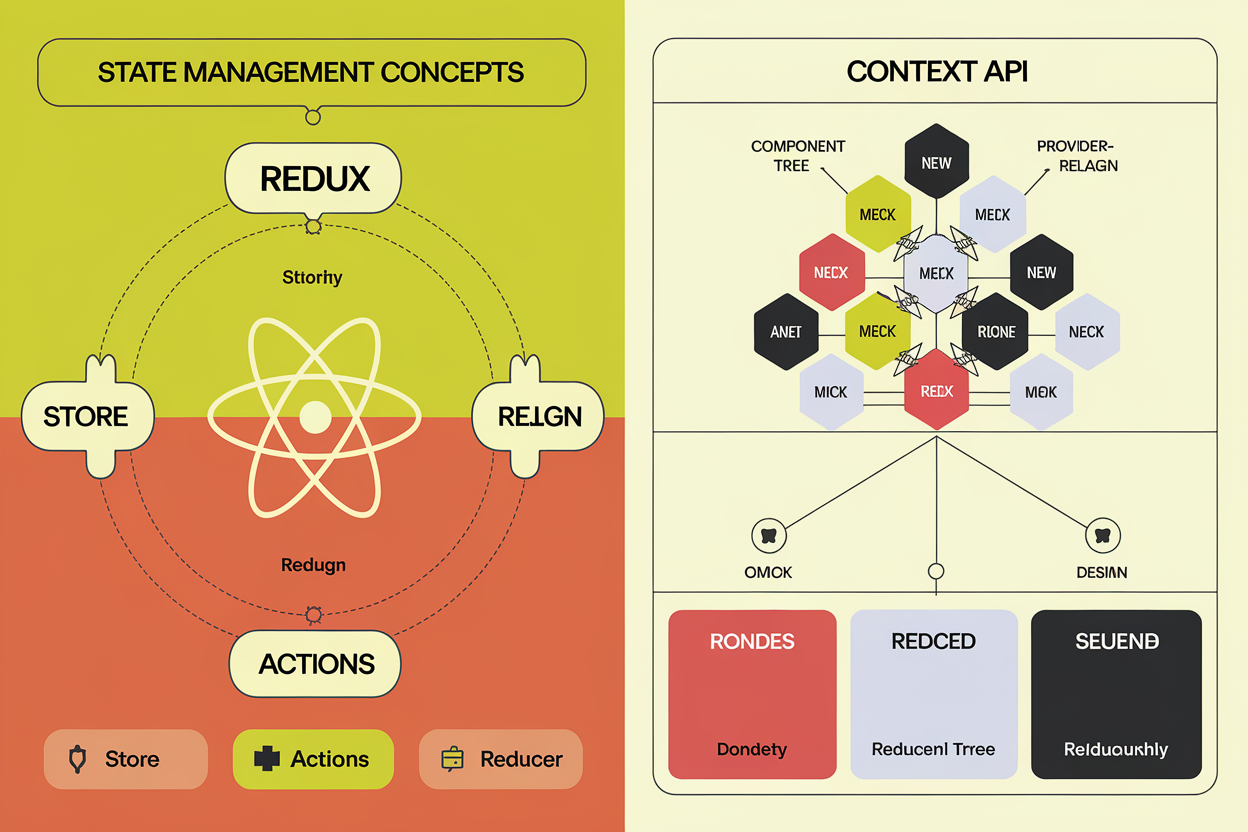
Handling State Management in React: A Guide to Redux and Context API
Learn how to handle state management in React with Redux and Context API. This guide explores the differences, benefits, and use cases of each approach, helping you choose the best solution for your React applications.
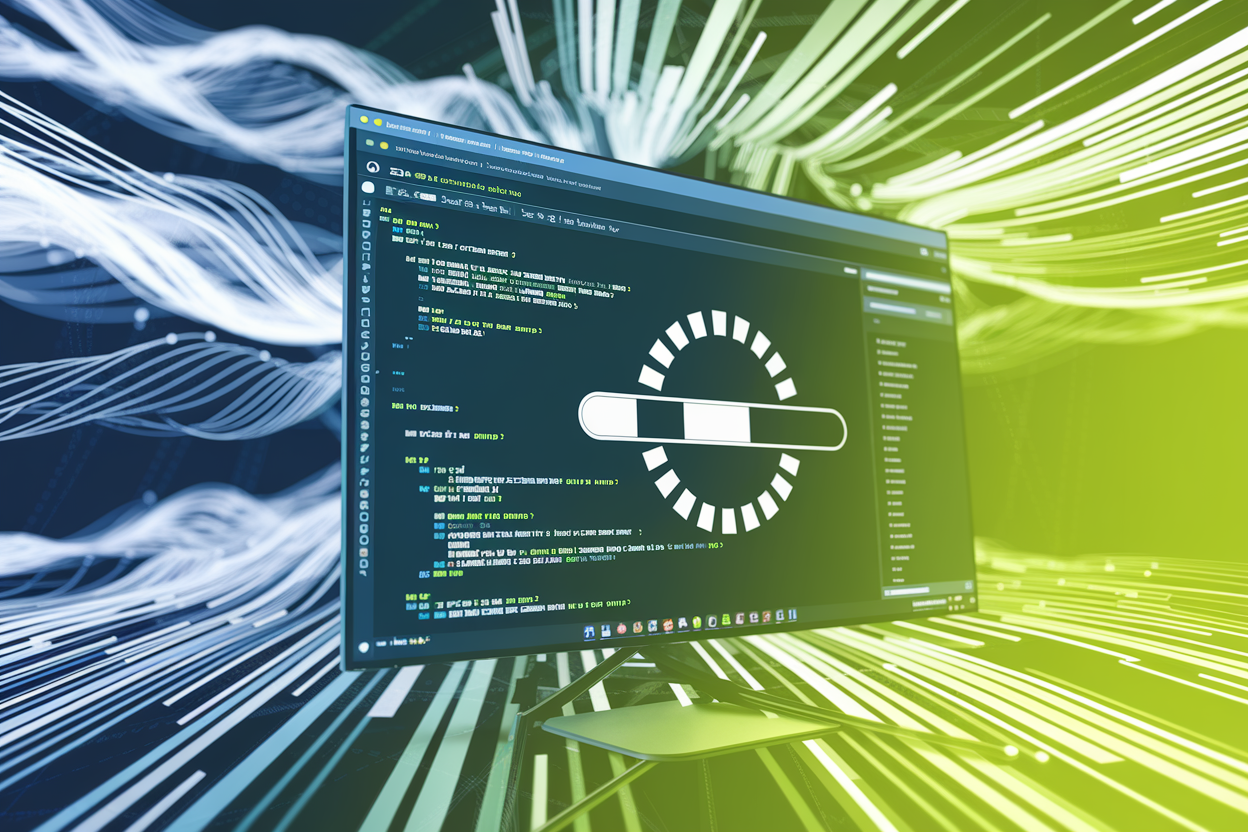
Mastering Puppeteer: Automating Web Tasks with Headless Browsers
Learn how to master Puppeteer for automating web tasks using headless browsers. This guide covers setup, basic examples, advanced features, and best practices for efficient web automation.
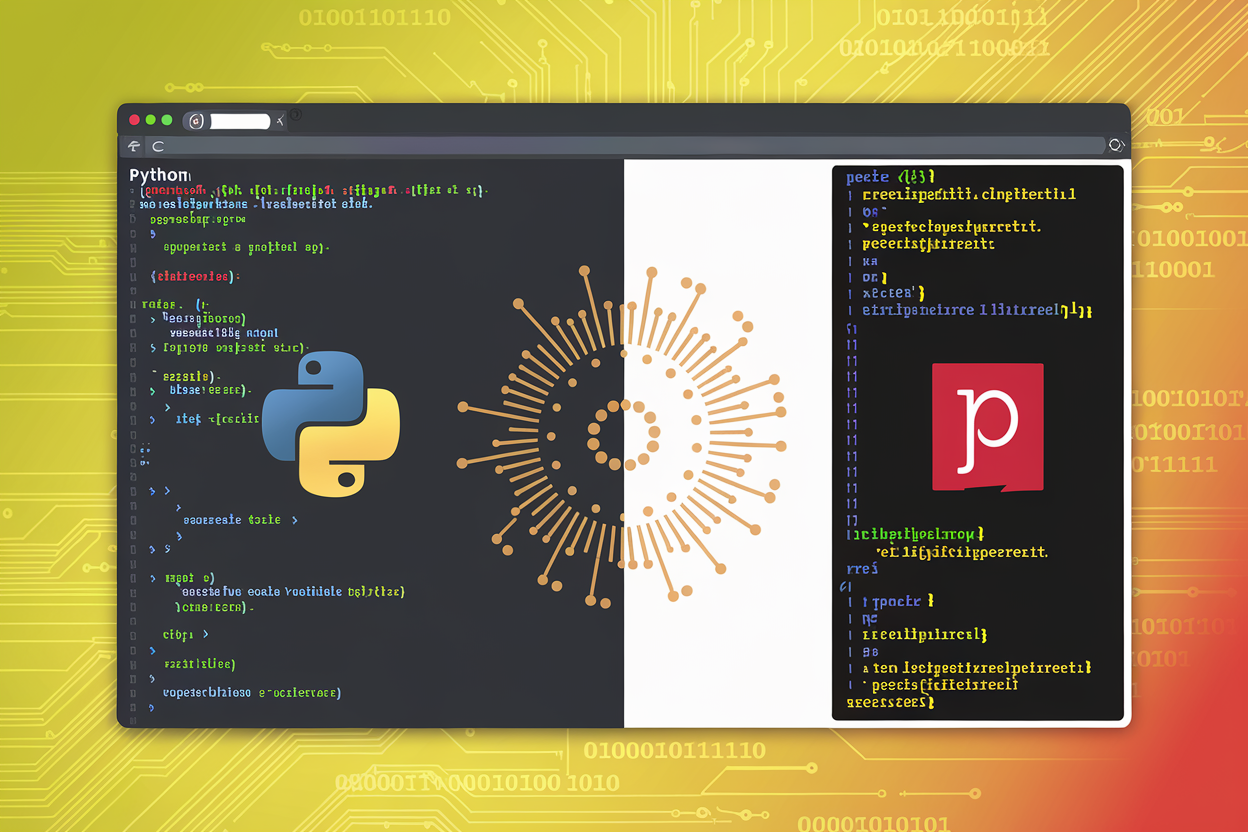
Handling Dynamic Content: Scraping JavaScript-Heavy Websites with Selenium and Puppeteer
Discover how to scrape JavaScript-heavy websites using Selenium and Puppeteer. This guide provides insights and code examples for handling dynamic content and extracting valuable data from web pages.
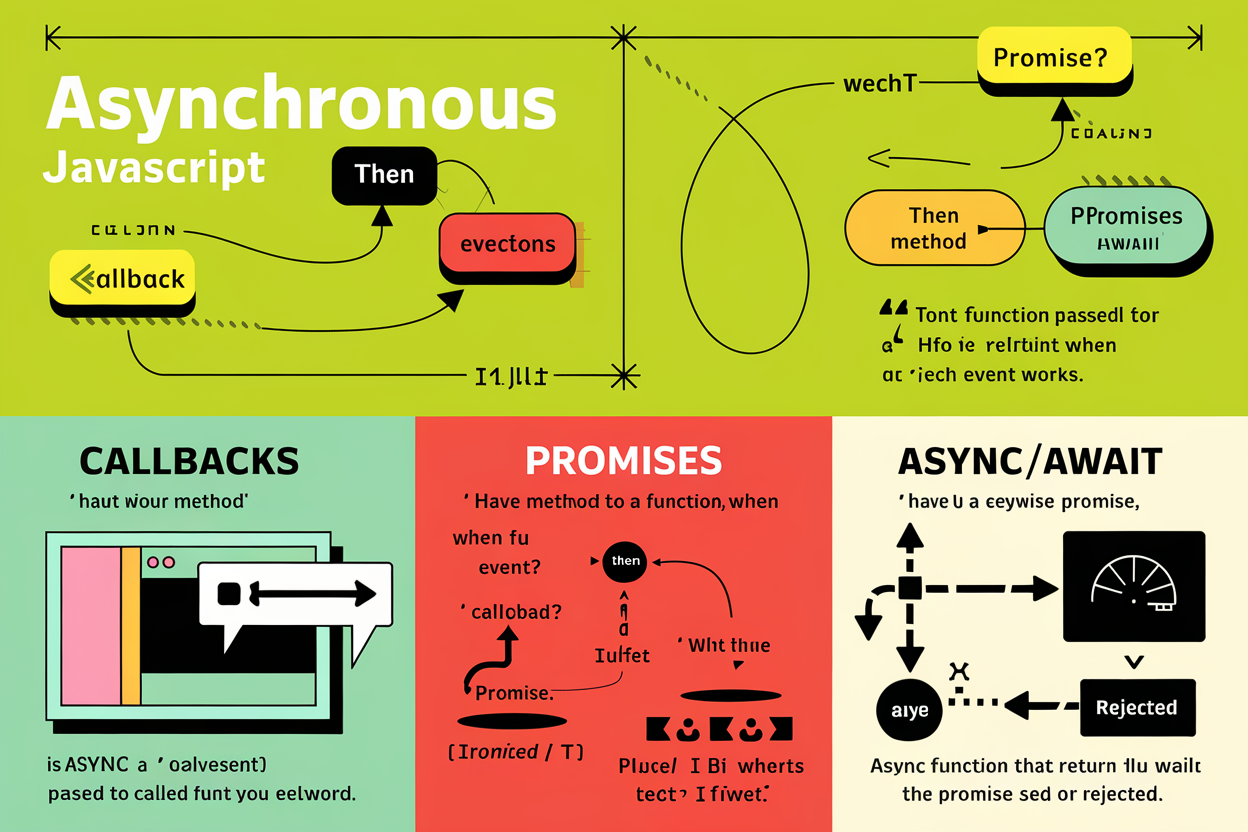
Asynchronous JavaScript: Mastering Promises, Async/Await, and Callbacks
Dive into asynchronous JavaScript with this guide to mastering Promises, async/await, and callbacks. Learn how to handle asynchronous operations efficiently and improve your coding skills.
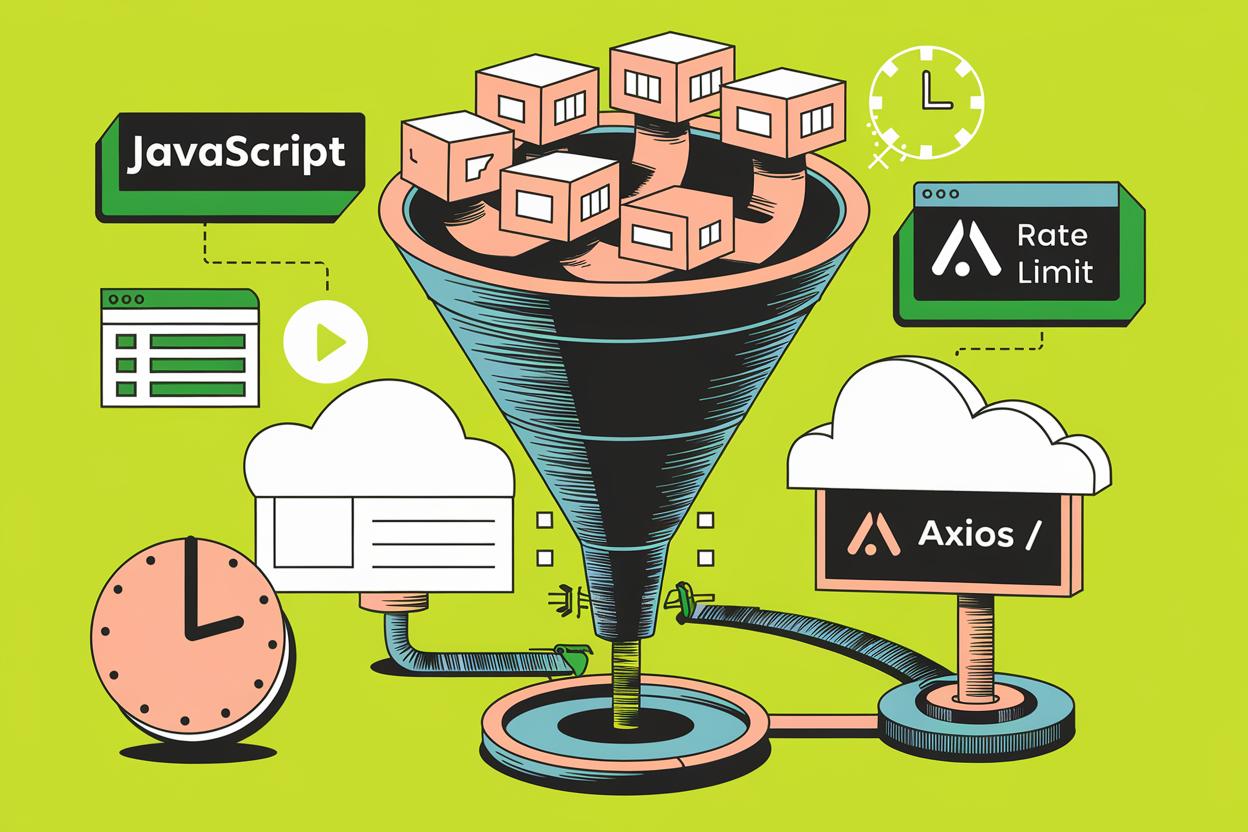
Handling API Rate Limits: Queueing API Requests with JavaScript
Learn how to manage API rate limits in JavaScript by queueing API requests. This guide covers the importance of rate limiting, use cases, and a practical example to get you started.
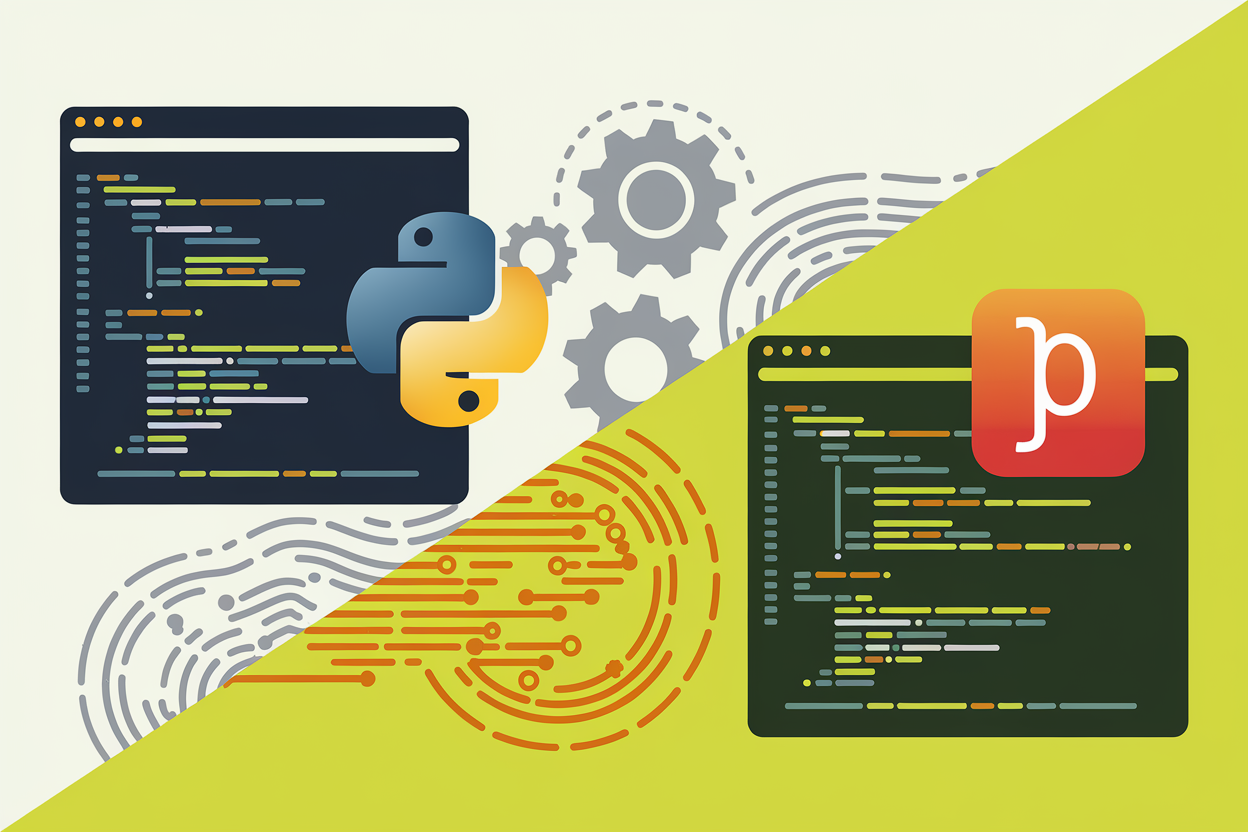
Automating Repetitive Tasks: Using Python and JavaScript for Web Automation
Learn how to automate repetitive tasks using Python and JavaScript. This guide covers automation with Selenium in Python and Puppeteer in JavaScript, providing examples and best practices for effective web automation.