Handling Dynamic Content: Scraping JavaScript-Heavy Websites with Selenium and Puppeteer
By Kainat Chaudhary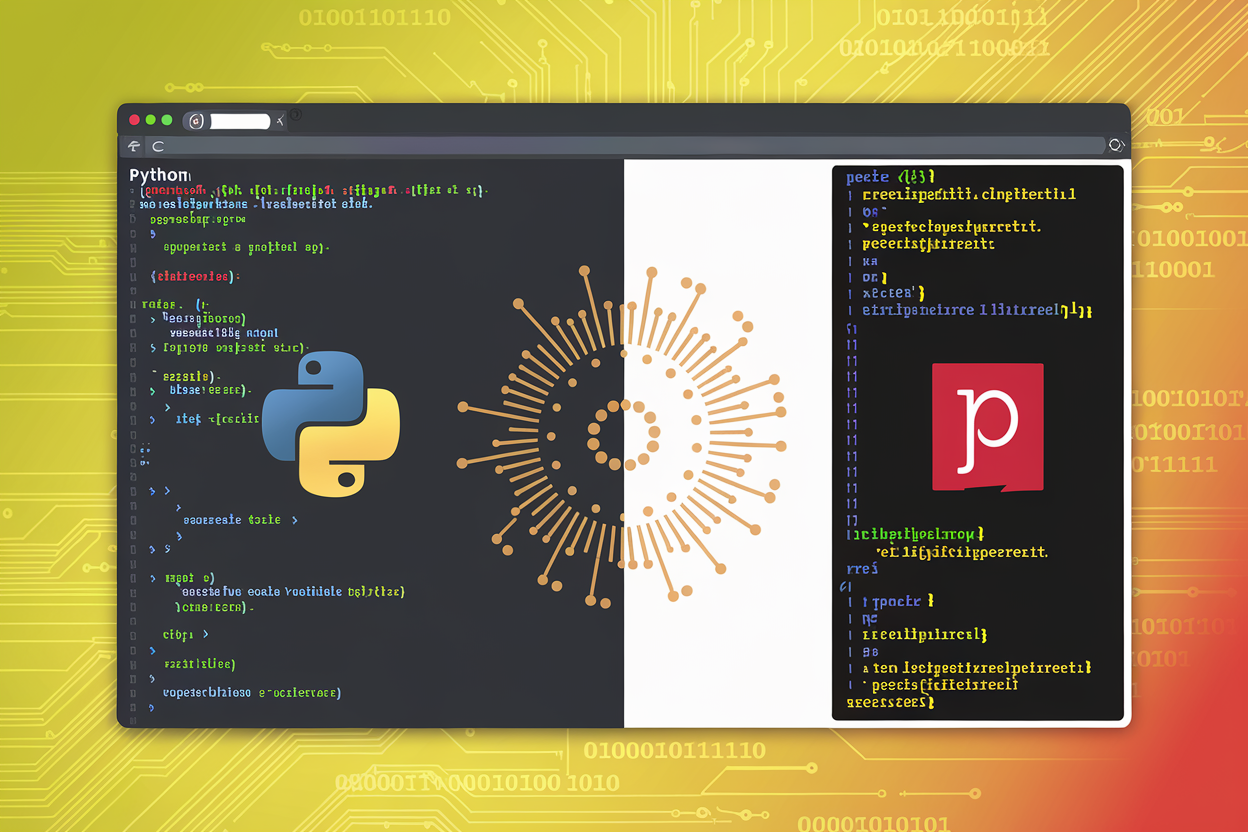
Introduction
Scraping data from websites that use JavaScript to dynamically load content can be challenging. Traditional scraping methods often fail to capture the dynamic elements rendered by JavaScript. However, tools like Selenium and Puppeteer offer powerful solutions for extracting data from such sites. This guide will explore how to use these tools to handle dynamic content and ensure you can effectively scrape data from JavaScript-heavy websites.
Understanding Dynamic Content
Dynamic content is content that is generated or updated in real-time by JavaScript on the client-side. Unlike static HTML, which is delivered fully formed from the server, dynamic content is often loaded asynchronously after the initial page load. This means that to scrape such content, you need to interact with the page and wait for the JavaScript to execute and render the content.
Scraping with Selenium
Selenium is a widely used tool for automating web browsers. It can be used to navigate through pages, interact with elements, and wait for content to load. Here’s a basic example of how to use Selenium with Python to scrape dynamic content:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.support.ui import WebDriverWait
from selenium.webdriver.support import expected_conditions as EC
# Set up the webdriver
driver = webdriver.Chrome()
# Navigate to the webpage
driver.get('https://example.com')
# Wait for dynamic content to load
try:
element = WebDriverWait(driver, 10).until(
EC.presence_of_element_located((By.CSS_SELECTOR, 'selector-for-dynamic-content'))
)
# Extract the content
content = element.text
finally:
driver.quit()
print(content)
In this example, we set up a Chrome webdriver, navigate to a webpage, and use WebDriverWait to wait until the dynamic content is present before extracting it. Adjust the `By.CSS_SELECTOR` to match the selector of the element you want to scrape.
Scraping with Puppeteer
Puppeteer is a Node.js library that provides a high-level API to control Chrome or Chromium over the DevTools Protocol. It is particularly well-suited for scraping dynamic content. Here’s an example of how to use Puppeteer to scrape a JavaScript-heavy website:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Wait for dynamic content to load
await page.waitForSelector('selector-for-dynamic-content');
const content = await page.$eval('selector-for-dynamic-content', element => element.textContent);
console.log(content);
await browser.close();
})();
In this Puppeteer example, we launch a browser instance, navigate to the webpage, and wait for the dynamic content to be present using `waitForSelector`. We then extract the content using `$eval` and print it.
Choosing Between Selenium and Puppeteer
Both Selenium and Puppeteer are effective tools for scraping JavaScript-heavy websites, but they have different strengths: - Selenium: Well-established with support for multiple browsers and languages. It’s ideal for cross-browser testing and has a large ecosystem. - Puppeteer: Specifically designed for Chromium-based browsers. It offers a more modern API and is optimized for performance and headless browsing. Your choice will depend on your specific requirements, such as the need for cross-browser support or a focus on performance.
Best Practices
- Respect the website's `robots.txt` and terms of service to avoid scraping restrictions or legal issues.
- Implement delays and throttling to avoid overwhelming the server with requests.
- Handle exceptions and errors gracefully to ensure your scraper can recover from failures.
- Use headless mode where appropriate to reduce resource usage and speed up scraping.
Conclusion
Scraping JavaScript-heavy websites requires handling dynamic content that is loaded or updated by JavaScript. Both Selenium and Puppeteer are powerful tools for this task, each with its own strengths. By understanding how to use these tools effectively, you can extract valuable data from websites that rely on dynamic content.
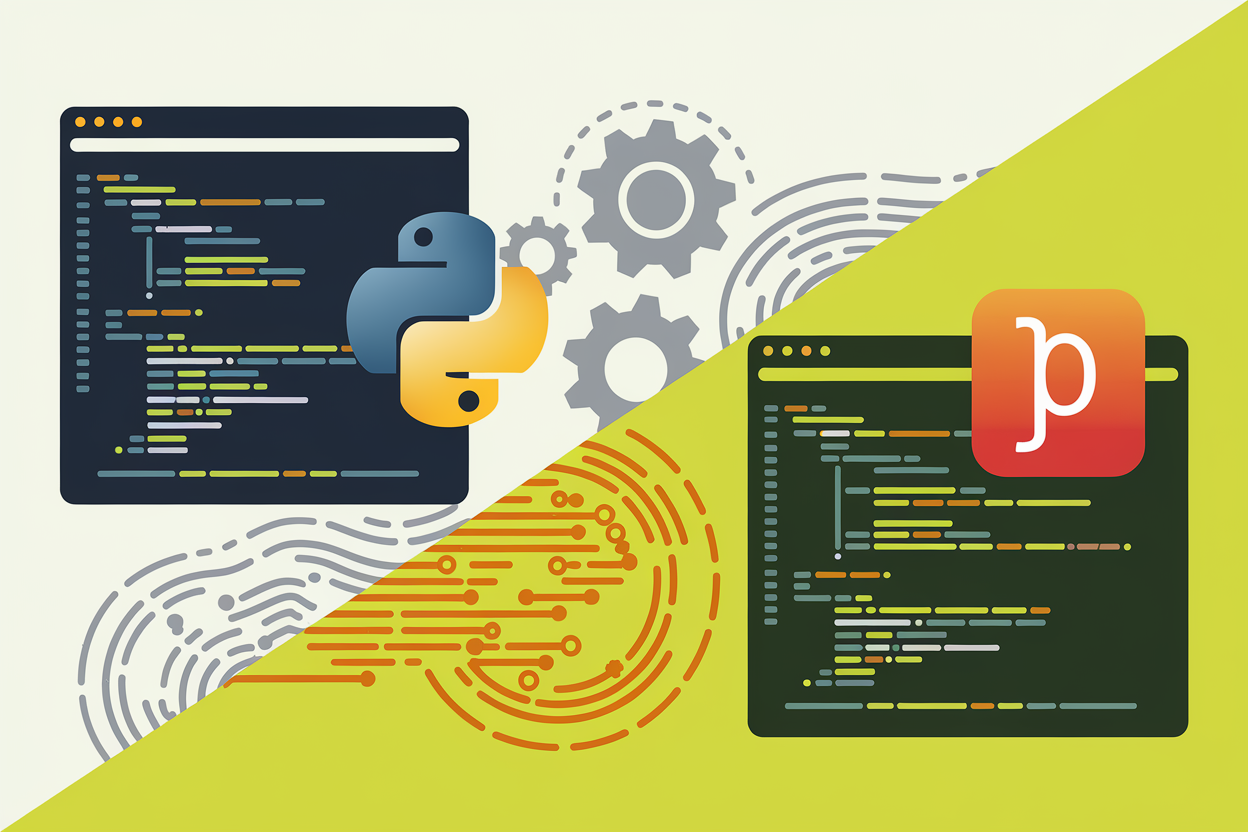
Automating Repetitive Tasks: Using Python and JavaScript for Web Automation
Learn how to automate repetitive tasks using Python and JavaScript. This guide covers automation with Selenium in Python and Puppeteer in JavaScript, providing examples and best practices for effective web automation.
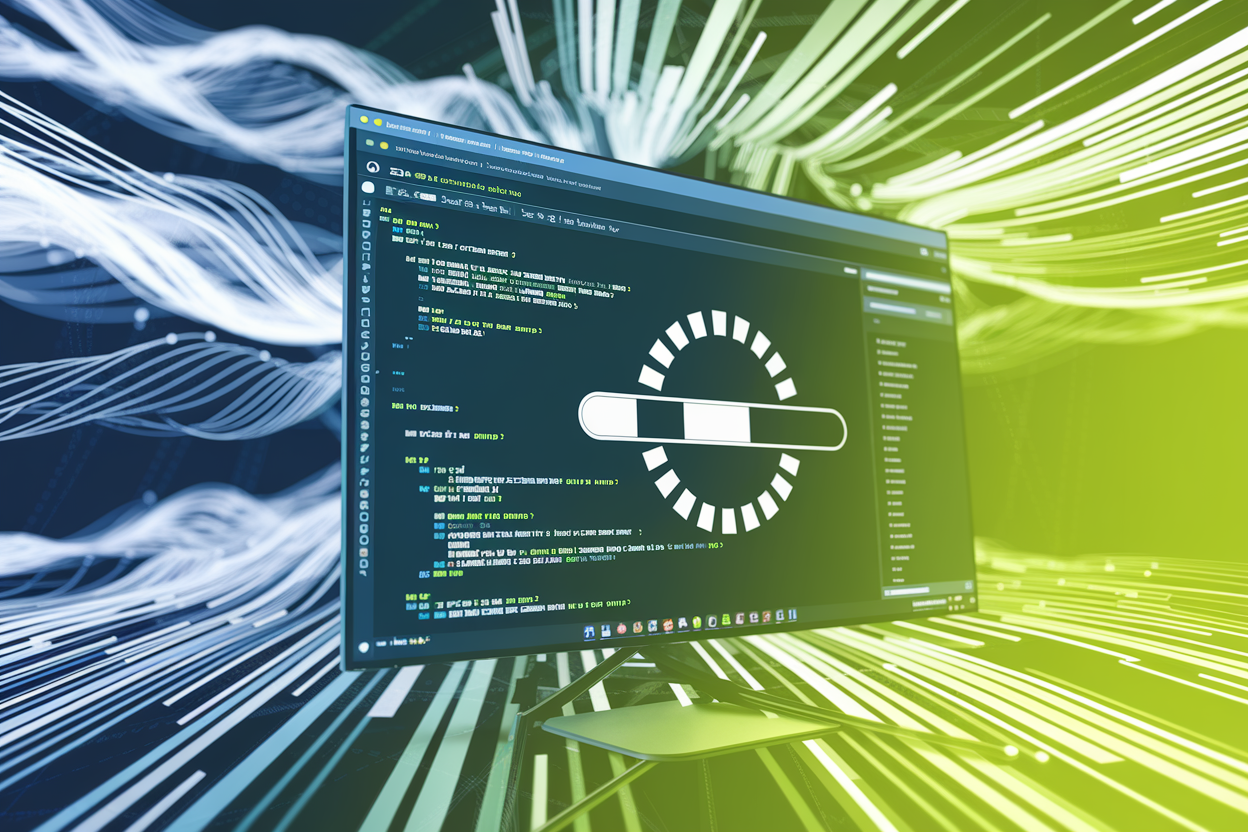
Mastering Puppeteer: Automating Web Tasks with Headless Browsers
Learn how to master Puppeteer for automating web tasks using headless browsers. This guide covers setup, basic examples, advanced features, and best practices for efficient web automation.
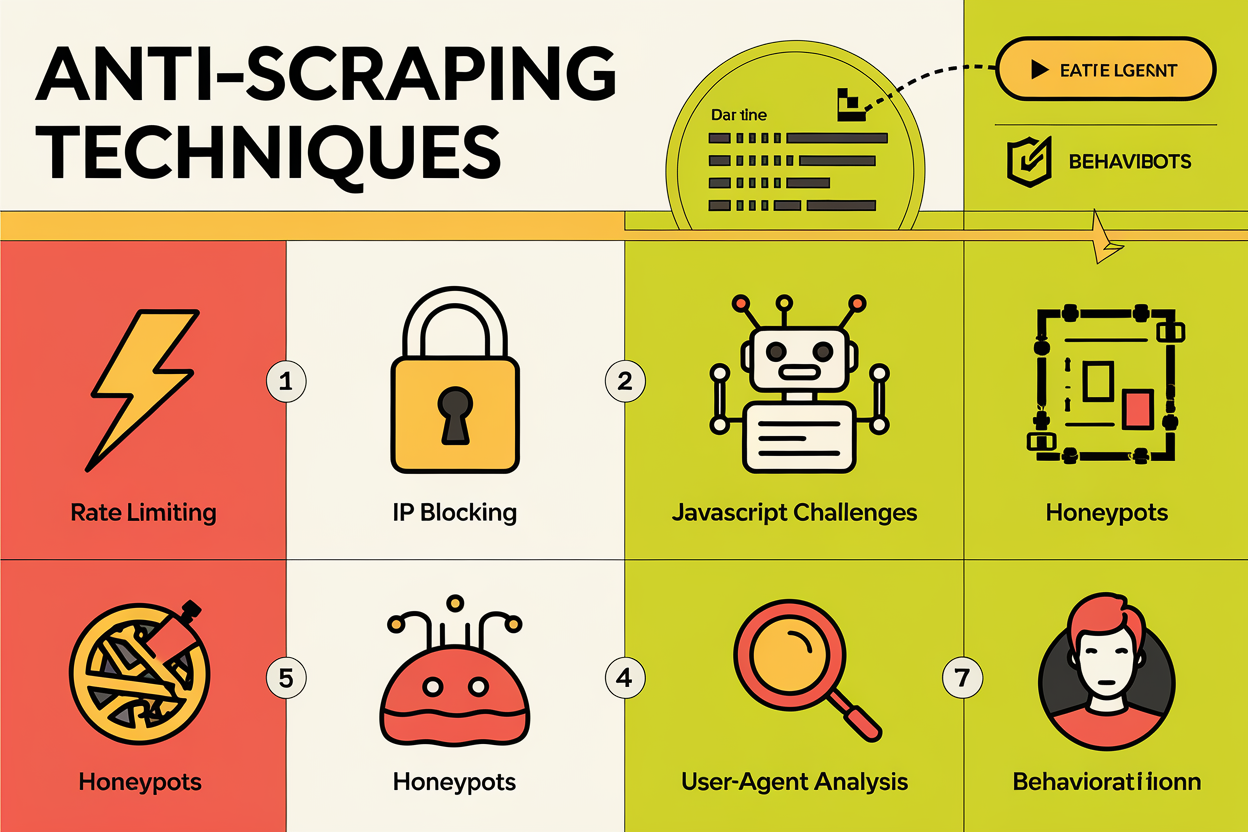
Understanding Anti-Scraping Techniques: How Websites Detect and Block Bots
Explore the anti-scraping techniques websites use to detect and block bots. Learn about rate limiting, IP blocking, CAPTCHAs, JavaScript challenges, honeypots, and more in this comprehensive guide.
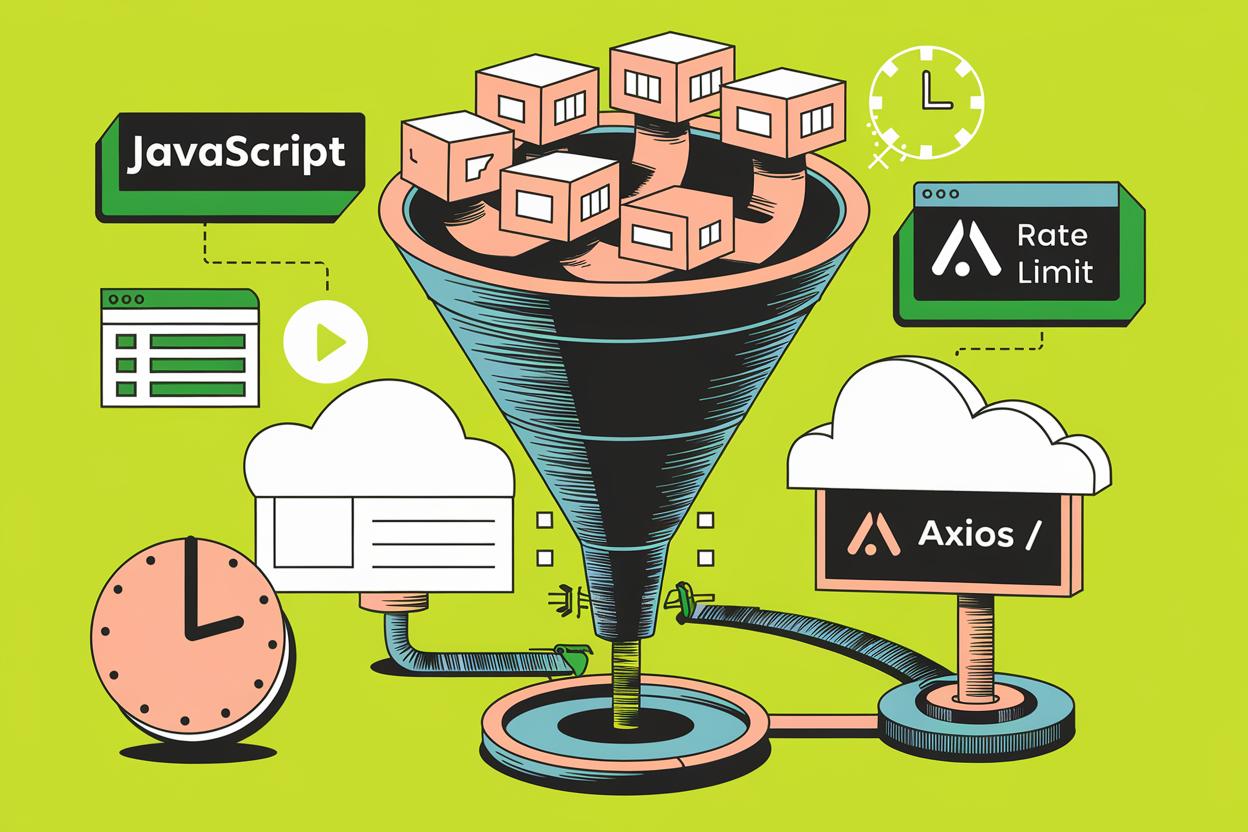
Handling API Rate Limits: Queueing API Requests with JavaScript
Learn how to manage API rate limits in JavaScript by queueing API requests. This guide covers the importance of rate limiting, use cases, and a practical example to get you started.