Automating Repetitive Tasks: Using Python and JavaScript for Web Automation
By Kainat Chaudhary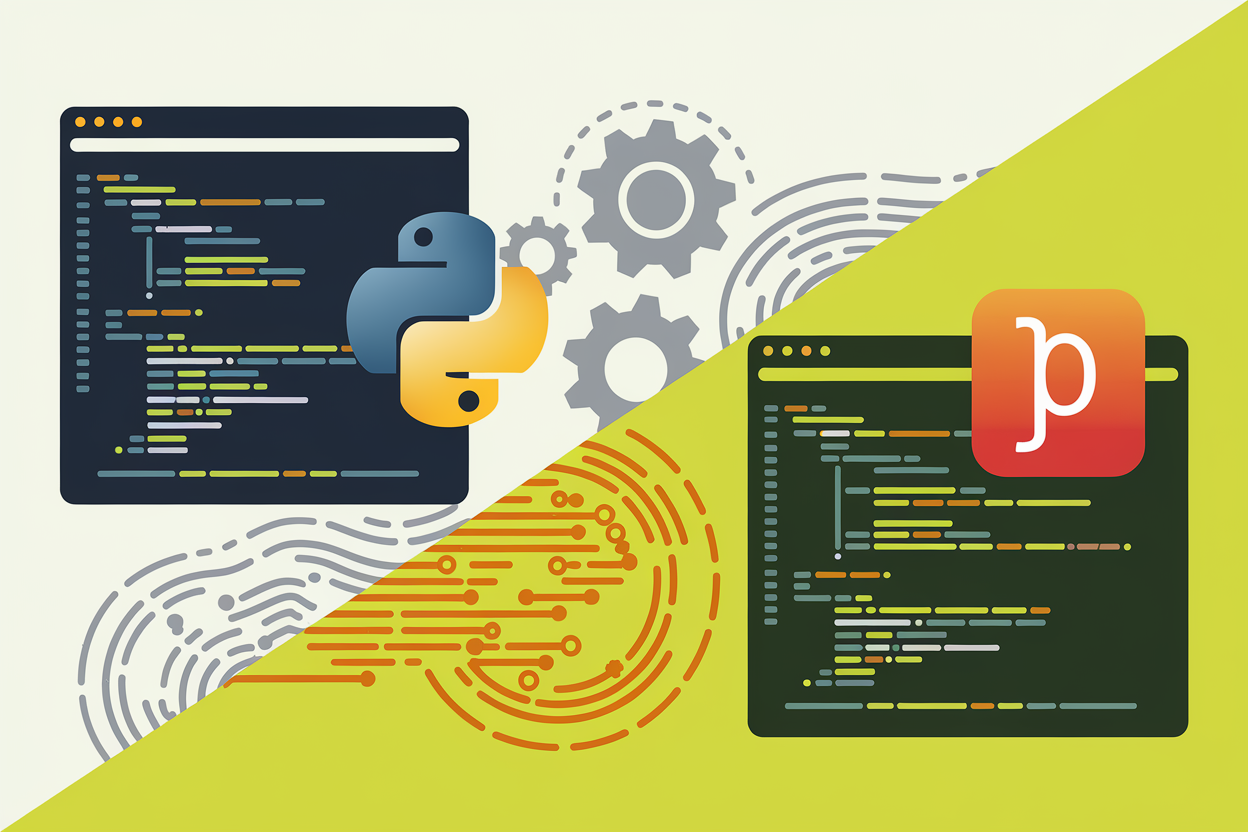
Introduction
Repetitive tasks can be time-consuming and error-prone when done manually. Fortunately, web automation allows you to streamline these tasks, saving time and improving accuracy. In this blog post, we'll explore how to automate repetitive tasks using Python and JavaScript, two popular programming languages for web automation.
Automating with Python
Python is a versatile language known for its simplicity and readability. It has several libraries that make web automation straightforward. One of the most popular libraries for this purpose is Selenium. Here's how you can use Selenium with Python to automate repetitive web tasks:
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.common.keys import Keys
# Set up the WebDriver
driver = webdriver.Chrome()
# Open a website
driver.get('https://example.com')
# Find an element and interact with it
search_box = driver.find_element(By.NAME, 'q')
search_box.send_keys('Python automation')
search_box.send_keys(Keys.RETURN)
# Wait for results to load and scrape data
# ... (additional code for scraping)
# Close the browser
driver.quit()
In this example, we use Selenium to open a website, find a search box, and perform a search. Selenium handles the browser automation and interaction, making it easy to automate repetitive web tasks.
Automating with JavaScript
JavaScript is another powerful language for web automation, especially for client-side tasks. Puppeteer, a Node.js library, is commonly used for automating web interactions with JavaScript. Here's a basic example of using Puppeteer to automate a web task:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Interact with web elements
await page.type('input[name="q"]', 'JavaScript automation');
await page.keyboard.press('Enter');
// ... (additional code for scraping)
await browser.close();
})();
This Puppeteer script launches a browser, opens a webpage, types a query into a search box, and performs a search. Puppeteer provides a high-level API for interacting with web pages, making it a great choice for automating tasks in JavaScript.
Comparing Python and JavaScript for Web Automation
Both Python and JavaScript offer powerful tools for web automation. Here’s a brief comparison to help you choose the best option for your needs: - Python: Selenium is a robust library for browser automation in Python. It is ideal for complex automation tasks and is widely used in both testing and data scraping. - JavaScript: Puppeteer is optimized for use with Chromium-based browsers and provides a modern API for web automation. It is particularly useful for automating client-side interactions and is often used for testing and web scraping.
Best Practices
- Ensure your automation scripts handle exceptions and errors gracefully to avoid disruptions.
- Respect the website’s `robots.txt` and terms of service to prevent legal issues or blocking.
- Implement delays and throttling to avoid overloading the server with requests.
- Regularly update your scripts to adapt to changes in website structure or functionality.
Conclusion
Automating repetitive tasks using Python and JavaScript can significantly boost productivity and accuracy. Whether you choose Python with Selenium or JavaScript with Puppeteer, both offer powerful solutions for web automation. By following best practices and using the right tools, you can streamline your workflows and handle repetitive tasks efficiently.
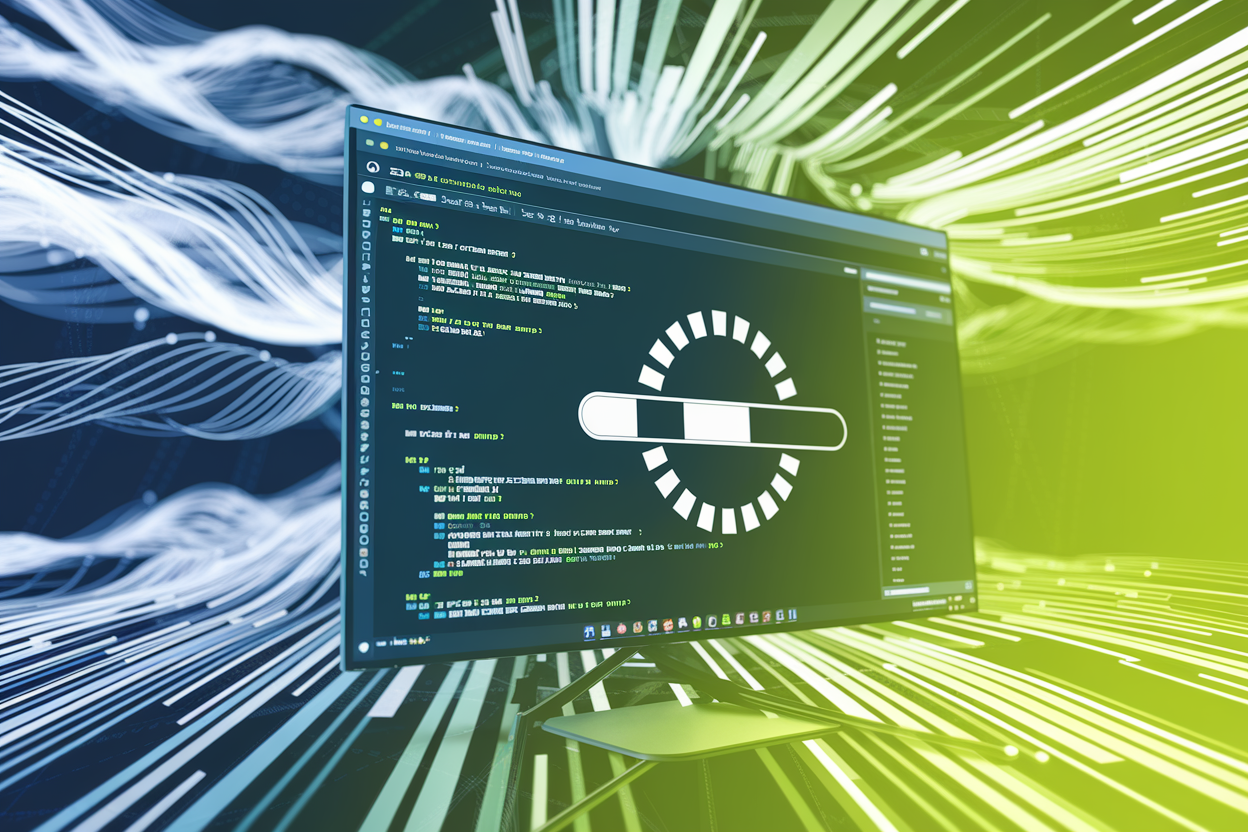
Mastering Puppeteer: Automating Web Tasks with Headless Browsers
Learn how to master Puppeteer for automating web tasks using headless browsers. This guide covers setup, basic examples, advanced features, and best practices for efficient web automation.
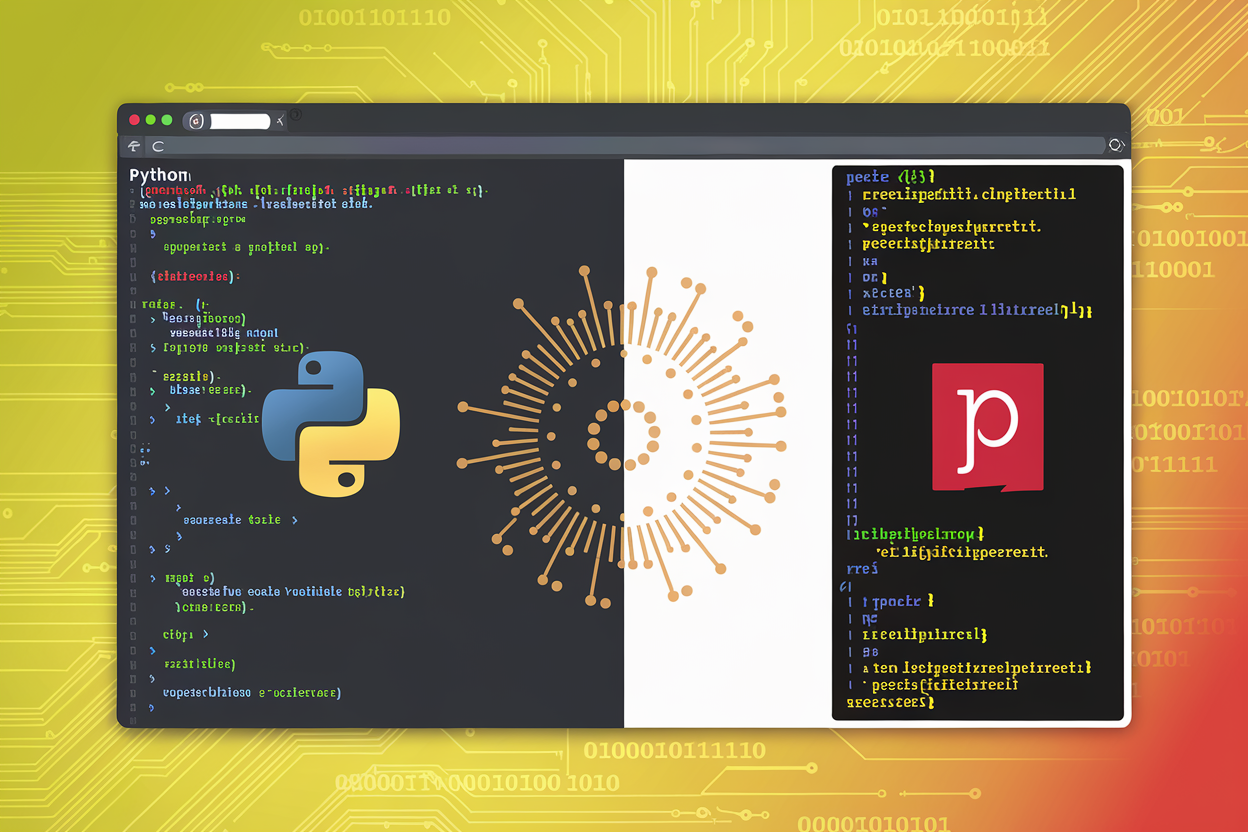
Handling Dynamic Content: Scraping JavaScript-Heavy Websites with Selenium and Puppeteer
Discover how to scrape JavaScript-heavy websites using Selenium and Puppeteer. This guide provides insights and code examples for handling dynamic content and extracting valuable data from web pages.
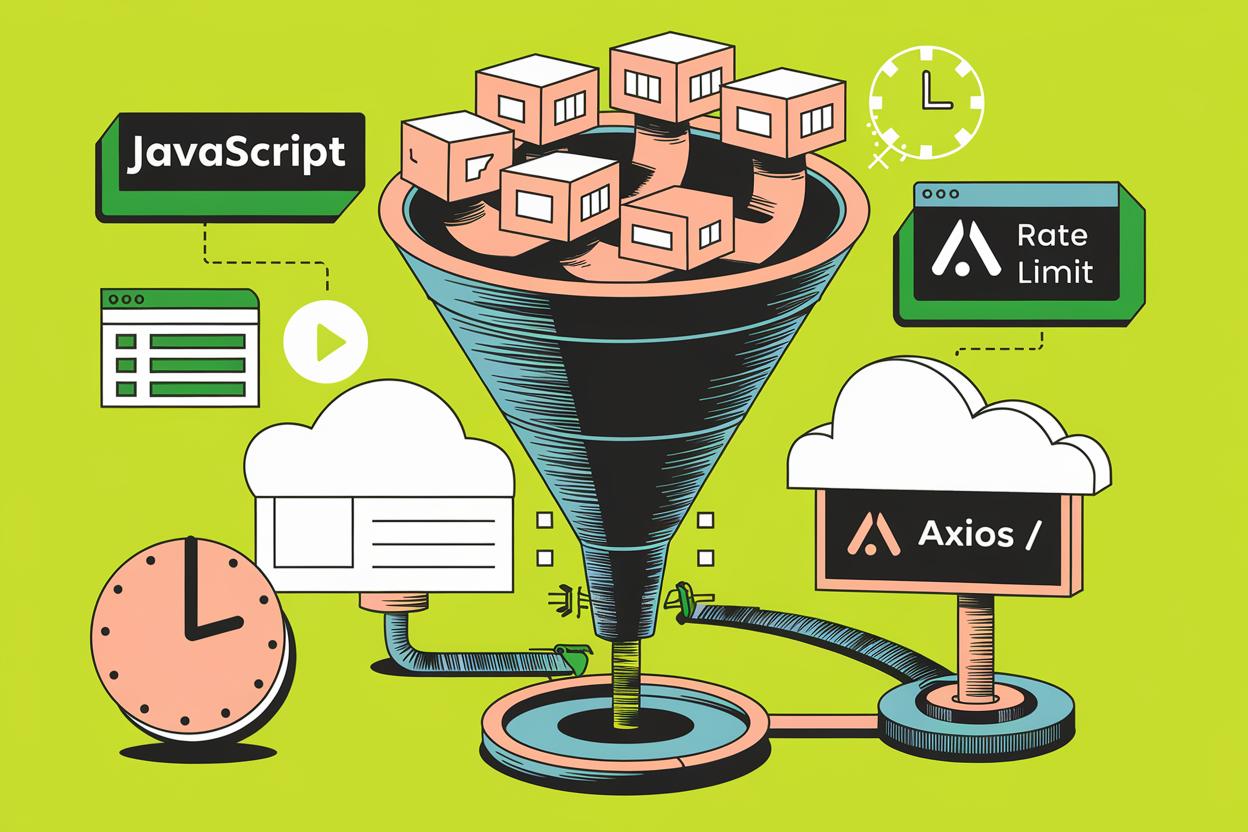
Handling API Rate Limits: Queueing API Requests with JavaScript
Learn how to manage API rate limits in JavaScript by queueing API requests. This guide covers the importance of rate limiting, use cases, and a practical example to get you started.