Handling API Rate Limits: Queueing API Requests with JavaScript
By Kainat Chaudhary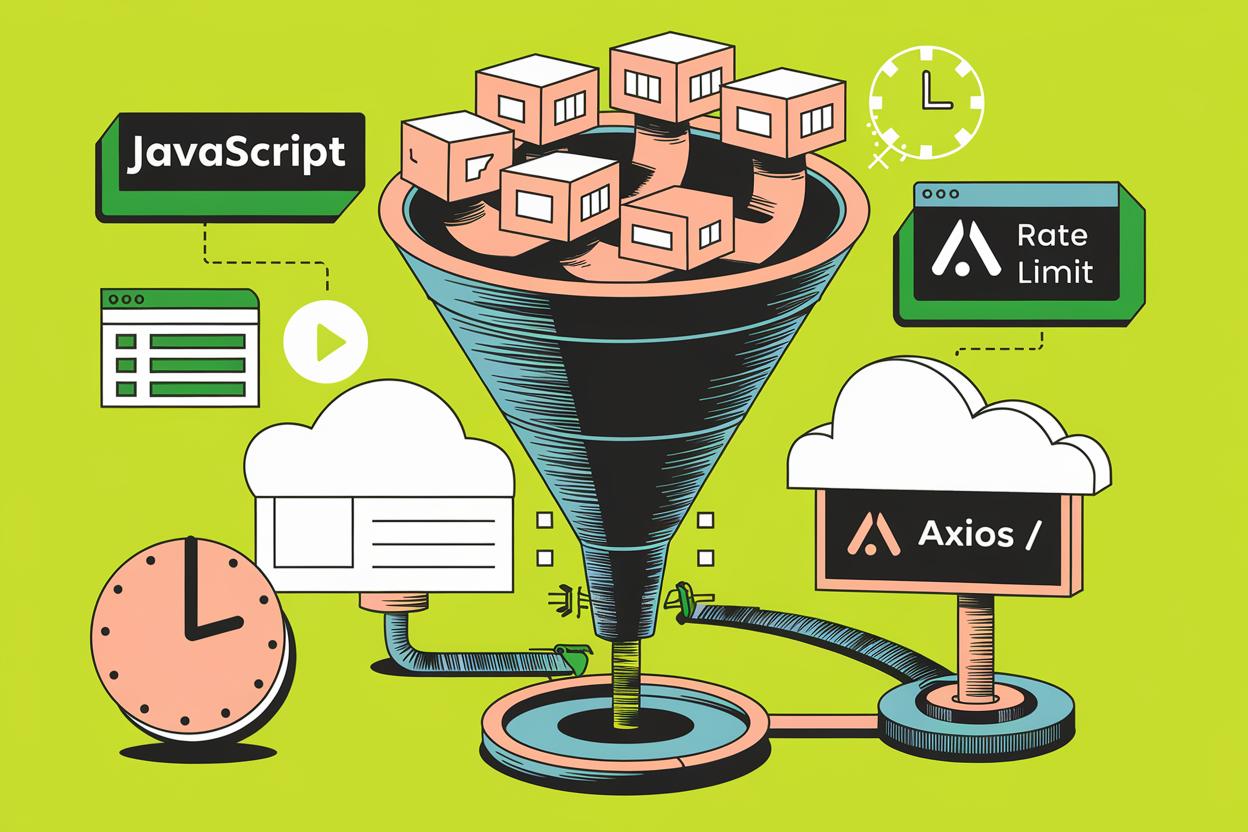
Introduction
When working with APIs, especially those that enforce rate limits, it's crucial to manage the number of requests sent in a given timeframe. Exceeding these limits can result in blocked requests, degraded performance, or even temporary bans from the API provider. In this post, we'll explore how to queue API requests in JavaScript to handle rate limits effectively, using a simple example code that demonstrates the concept.
Why Queue API Requests?
APIs often impose rate limits to ensure fair usage and maintain server stability. These limits cap the number of requests a user can make in a specific timeframe, such as 60 requests per minute. If your application exceeds these limits, you might face throttling, where requests are slowed down or rejected. Queueing requests allows you to control the flow of outgoing requests, ensuring they comply with the rate limits and prevent disruptions in service.
Use Cases for Queueing API Requests
- Preventing Rate Limit Errors: Avoid hitting API rate limits by spacing out requests appropriately.
- Improving Application Stability: Ensuring requests are sent at a controlled pace prevents sudden spikes that could affect your application's performance.
- Handling High Volume Data: When processing large datasets requiring multiple API calls, queueing helps manage the load efficiently.
- Ensuring Data Integrity: By controlling request timing, you reduce the risk of missing or duplicating data due to rejected requests.
Example: Queueing API Requests with JavaScript
Here's an example JavaScript class that manages API requests by queueing them and ensuring they respect a maximum rate of 4 requests per second. This approach helps avoid exceeding API rate limits and maintains smooth operation.
import axios from 'axios';
class APIRequestManager {
constructor(baseURL, maxRequestsPerSecond = 4) {
this.baseUrl = baseURL;
this.maxRequestsPerSecond = maxRequestsPerSecond;
this.queue = [];
this.processing = false;
}
async throttleRequests() {
const delayBetweenRequests = 1000 / this.maxRequestsPerSecond;
if (!this.processing) {
this.processing = true;
while (this.queue.length > 0) {
const { resolve, reject, request } = this.queue.shift();
try {
const response = await request();
resolve(response);
} catch (error) {
reject(error);
}
await new Promise(resolve => setTimeout(resolve, delayBetweenRequests));
}
this.processing = false;
}
}
enqueueRequest(request) {
return new Promise((resolve, reject) => {
this.queue.push({ request, resolve, reject });
this.throttleRequests();
});
}
async makeRequest(endpoint, method = 'GET', payload = null) {
const url = `${this.baseUrl}${endpoint}`;
const headers = {
'Content-Type': 'application/json'
};
return this.enqueueRequest(async () => {
try {
const response = await axios({ url, method, headers, data: payload });
return response.data;
} catch (error) {
console.error(`Request to ${url} failed:`, error.response?.data || error.message);
throw error;
}
});
}
}
How It Works
In the code above, `APIRequestManager` manages API requests by queueing them in an array. The `throttleRequests` method processes requests from the queue at a controlled rate, determined by the `maxRequestsPerSecond` setting. By adjusting the `delayBetweenRequests` value, you can control the frequency of API calls to stay within the API's rate limits.
Best Practices for Managing API Rate Limits
- Monitor API Responses: Keep an eye on API responses for any rate limit warnings or errors, and adjust your request rate accordingly.
- Implement Exponential Backoff: If requests fail due to rate limits, implement an exponential backoff strategy to gradually increase the delay between retries.
- Use API Rate Limit Headers: Some APIs provide headers that indicate the current rate limit status. Use this information to dynamically adjust your request rate.
- Test in a Controlled Environment: Before deploying your application, test your rate limiting logic in a controlled environment to ensure it behaves as expected under various conditions.
Conclusion
Handling API rate limits is essential for building reliable and scalable applications. By queueing API requests and controlling the rate at which they are sent, you can avoid common pitfalls associated with rate limits, such as throttling and request failures. Implementing a queueing mechanism like the one demonstrated in this guide ensures your application interacts smoothly with APIs, even under stringent rate limiting conditions.
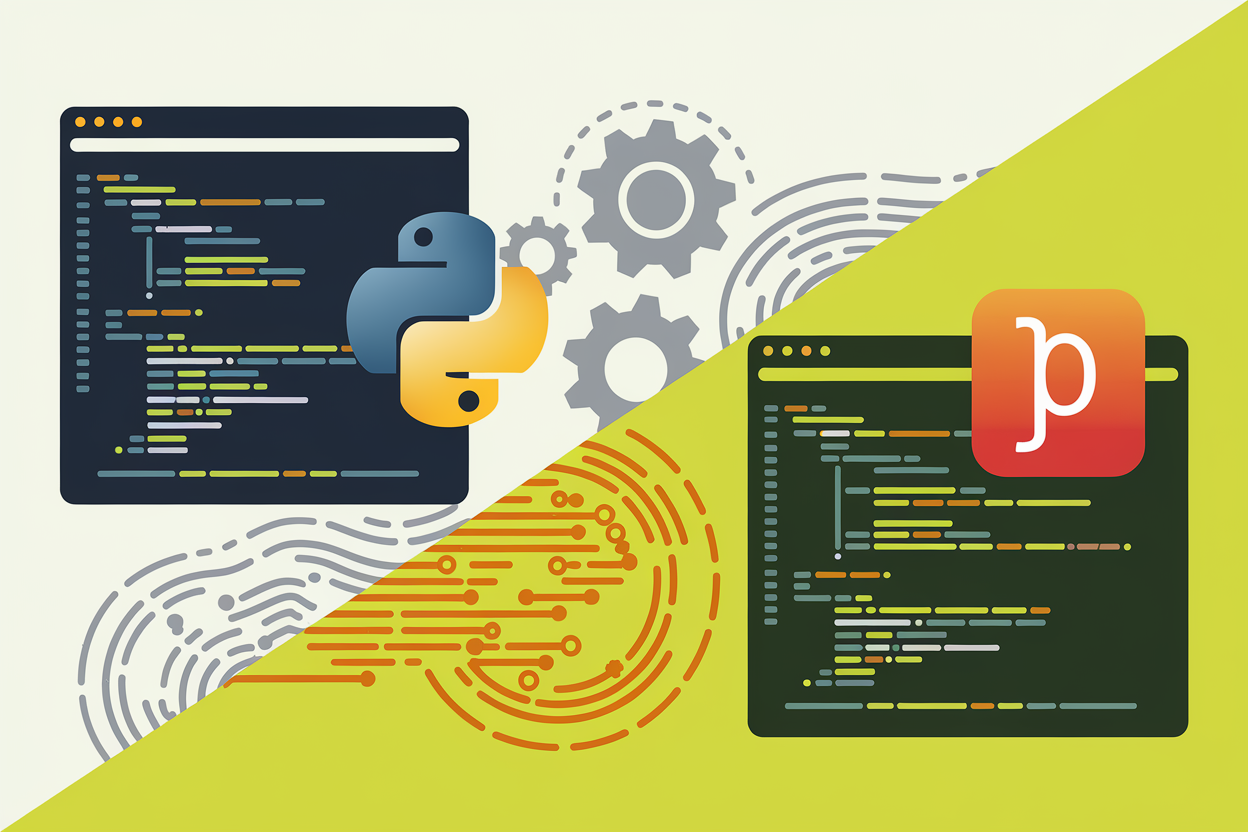
Automating Repetitive Tasks: Using Python and JavaScript for Web Automation
Learn how to automate repetitive tasks using Python and JavaScript. This guide covers automation with Selenium in Python and Puppeteer in JavaScript, providing examples and best practices for effective web automation.
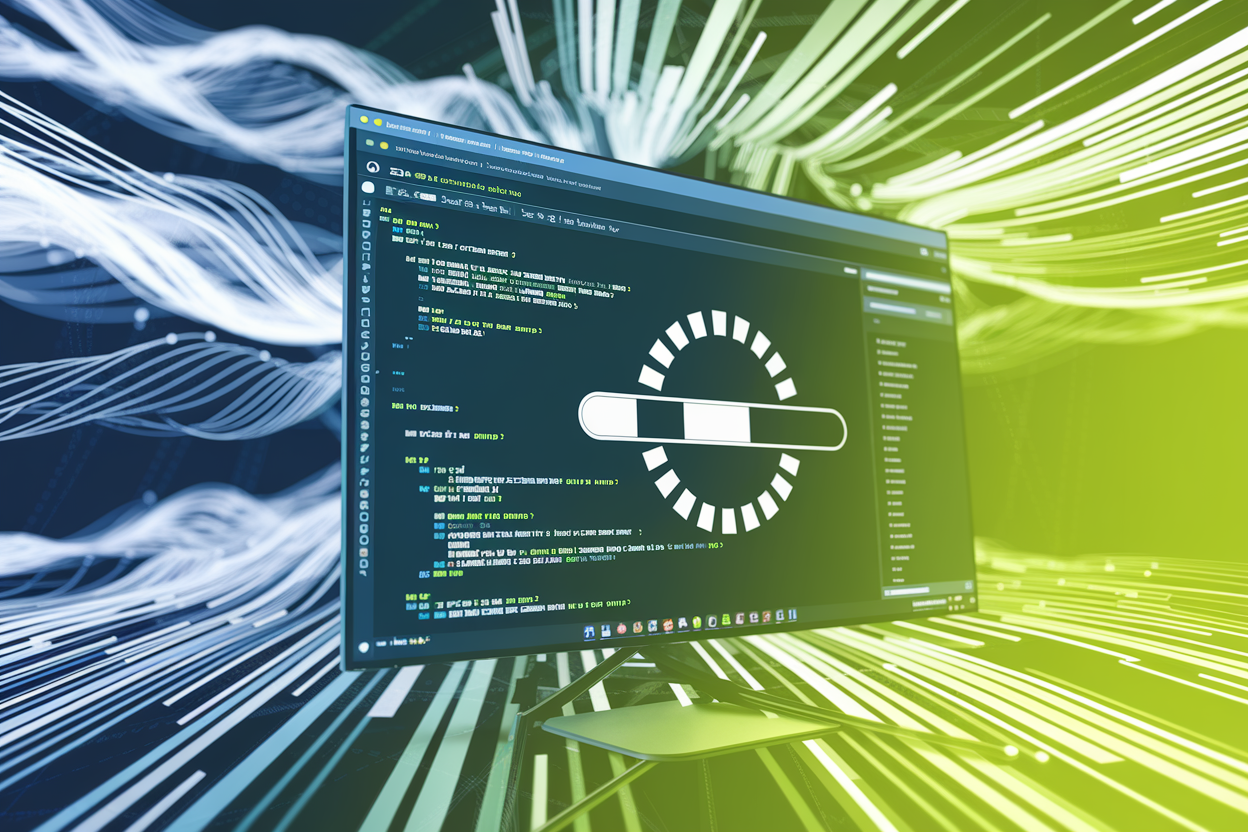
Mastering Puppeteer: Automating Web Tasks with Headless Browsers
Learn how to master Puppeteer for automating web tasks using headless browsers. This guide covers setup, basic examples, advanced features, and best practices for efficient web automation.
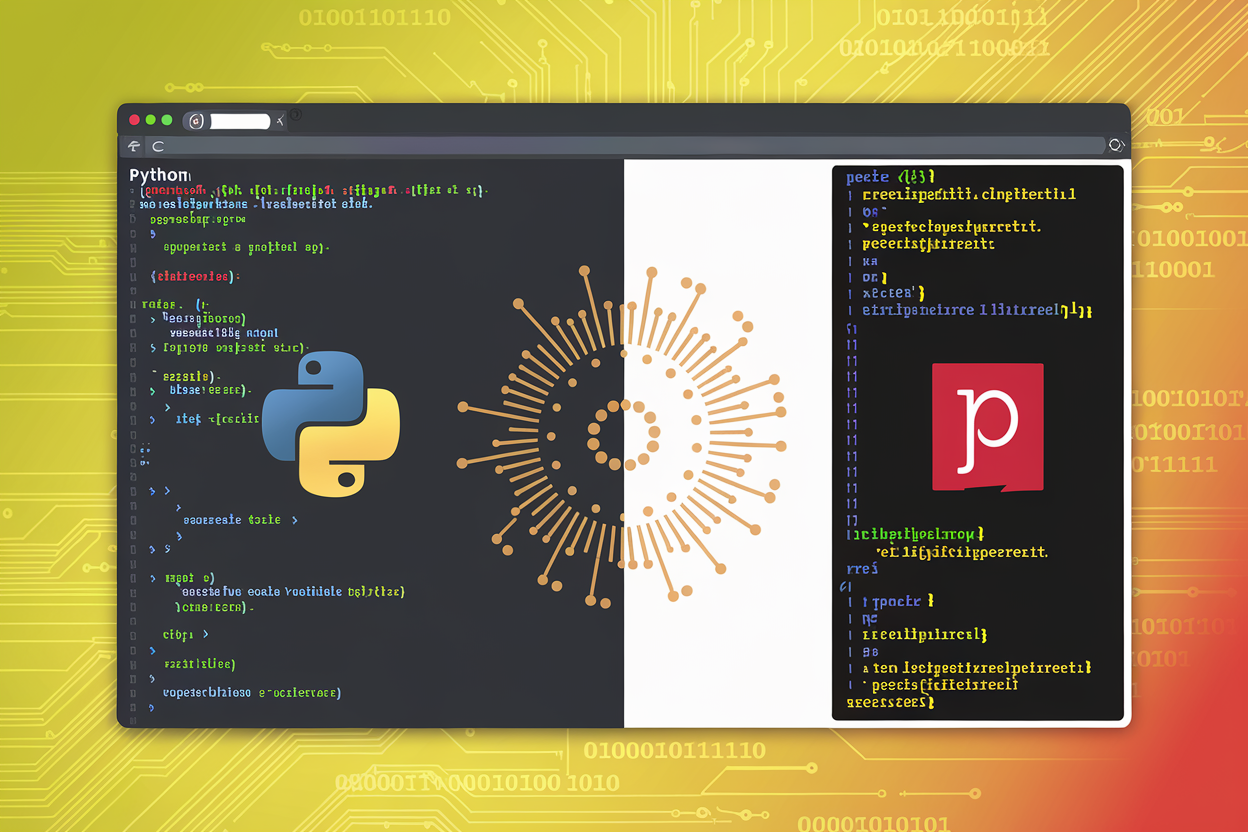
Handling Dynamic Content: Scraping JavaScript-Heavy Websites with Selenium and Puppeteer
Discover how to scrape JavaScript-heavy websites using Selenium and Puppeteer. This guide provides insights and code examples for handling dynamic content and extracting valuable data from web pages.
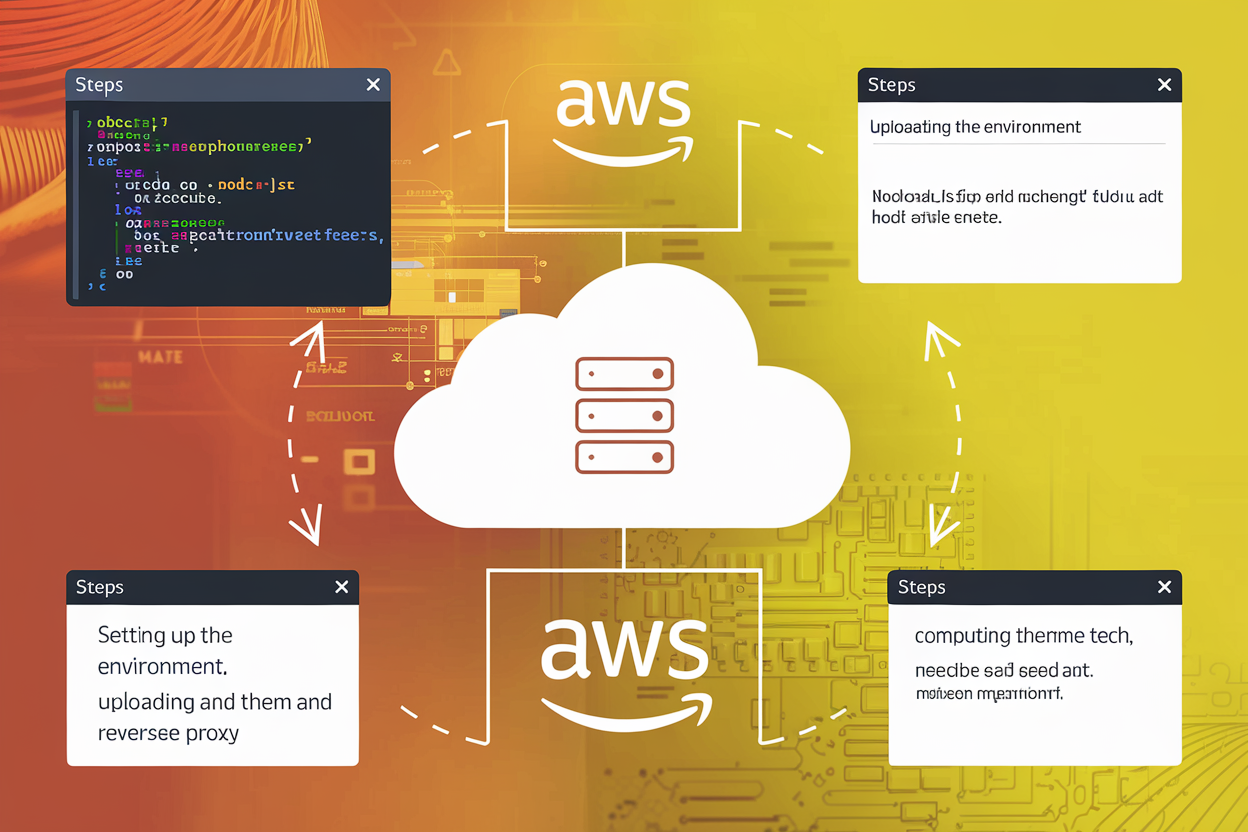
Deploying Node.js Apps on AWS: A Step-by-Step Guide
Learn how to deploy your Node.js applications on AWS EC2 with this step-by-step guide. From setting up your AWS account to configuring a reverse proxy, this guide covers everything you need for a successful deployment.