Mastering Puppeteer: Automating Web Tasks with Headless Browsers
By Kainat Chaudhary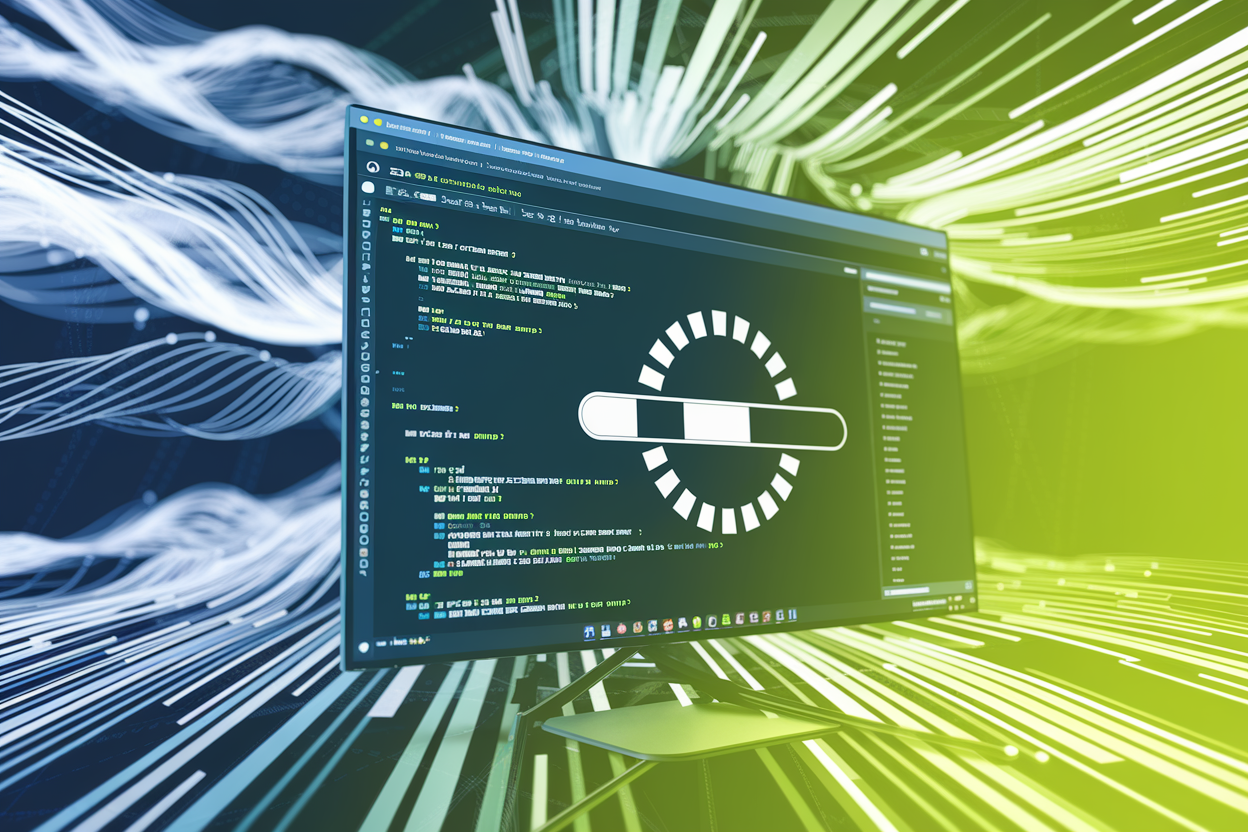
Introduction
Puppeteer is a powerful Node.js library that provides a high-level API to control headless Chrome or Chromium browsers. It is widely used for automating web tasks, performing end-to-end testing, and scraping dynamic content. This guide will help you master Puppeteer, enabling you to efficiently automate various web tasks with headless browsers.
Getting Started with Puppeteer
To start using Puppeteer, you need to install it via npm. Puppeteer comes with its own version of Chromium, so there's no need to install a separate browser. Here's how to set up Puppeteer in your Node.js project:
npm install puppeteer
Basic Puppeteer Example
Here’s a simple example of how to use Puppeteer to open a webpage, take a screenshot, and extract some text from it:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
// Take a screenshot
await page.screenshot({ path: 'screenshot.png' });
const text = await page.evaluate(() => document.body.innerText);
console.log(text);
await browser.close();
})();
In this example, we launch a headless browser, navigate to a webpage, take a screenshot, and extract the text content from the page. Puppeteer’s API provides powerful methods for interacting with web pages and extracting information.
Advanced Puppeteer Features
Puppeteer offers several advanced features that can enhance your automation tasks, including:
- Headless Mode: Run the browser in the background without a visible UI, which is useful for automated tasks and testing.
- Network Interception: Modify network requests and responses to simulate different conditions or manipulate content.
- Form Submission: Automate form filling and submission to test web applications or scrape data.
- Interaction Simulation: Simulate user interactions like clicks, typing, and scrolling to test or scrape dynamic content.
Headless vs. Full Browser Mode
Puppeteer supports both headless and full browser modes. Headless mode is often used for automation and testing due to its performance benefits, while full browser mode is useful for debugging and visual verification. You can switch between these modes with a simple configuration change:
const browser = await puppeteer.launch({ headless: false });
Use Cases for Puppeteer
- End-to-End Testing: Automate browser interactions to test web applications thoroughly.
- Web Scraping: Extract dynamic content from websites that rely heavily on JavaScript.
- Performance Monitoring: Measure page load times and other performance metrics.
- UI Testing: Test visual aspects of web pages and ensure consistency across different screen sizes and devices.
Best Practices
- Error Handling: Implement error handling to manage unexpected issues and improve script robustness.
- Performance Optimization: Optimize your scripts to minimize execution time and resource usage.
- Respect Robots.txt: Ensure your automation respects the website’s `robots.txt` file and terms of service.
- Secure Your Data: Be cautious with sensitive data and avoid exposing it in logs or error messages.
Conclusion
Puppeteer is a versatile tool for automating web tasks with headless browsers. By mastering Puppeteer, you can efficiently perform a wide range of tasks, from automated testing to web scraping. With its powerful features and flexibility, Puppeteer is an essential tool for modern web automation.
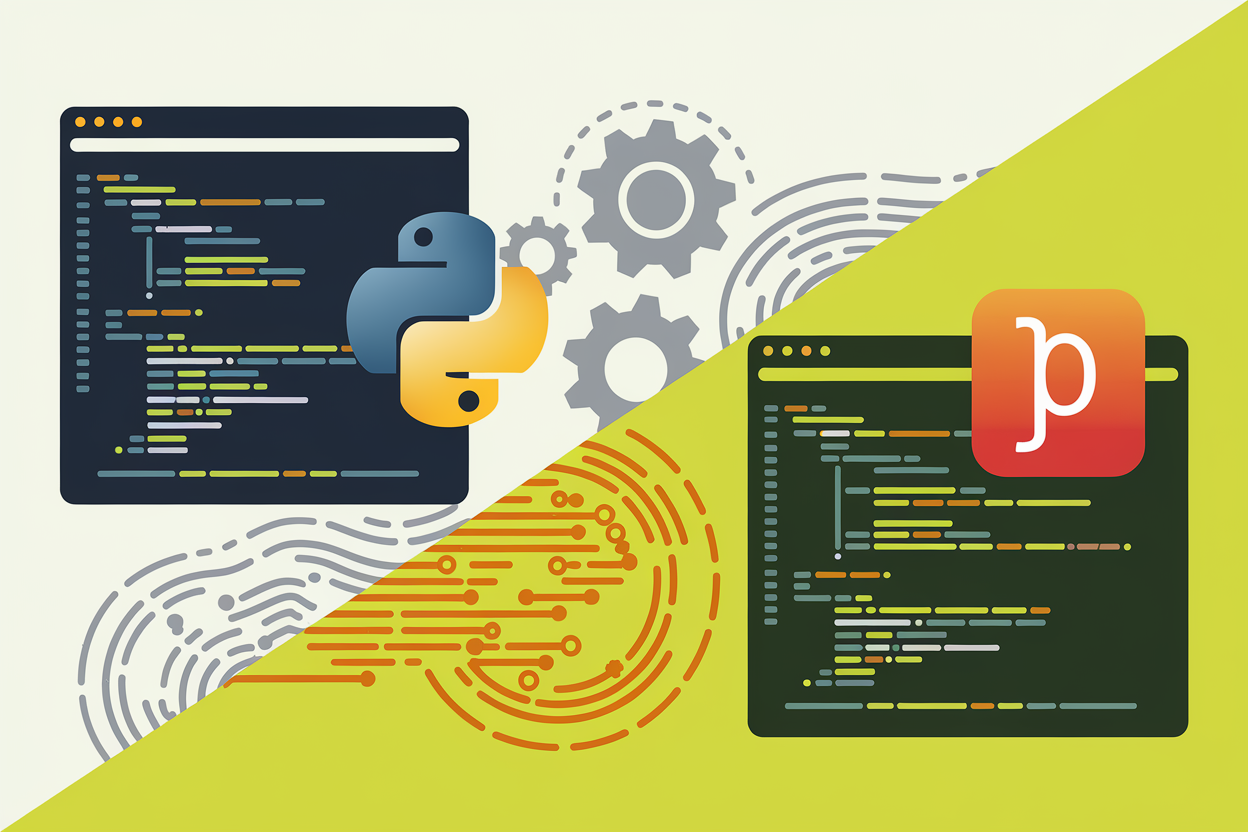
Automating Repetitive Tasks: Using Python and JavaScript for Web Automation
Learn how to automate repetitive tasks using Python and JavaScript. This guide covers automation with Selenium in Python and Puppeteer in JavaScript, providing examples and best practices for effective web automation.
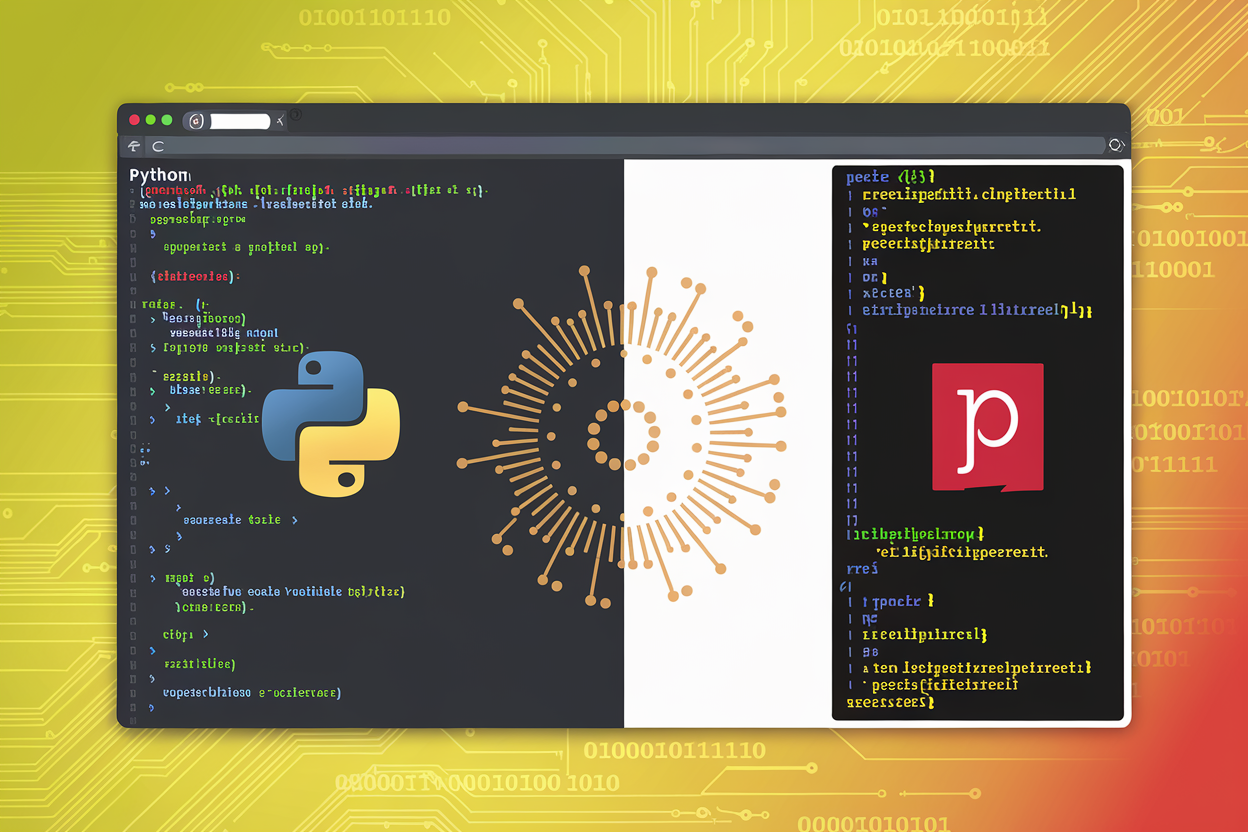
Handling Dynamic Content: Scraping JavaScript-Heavy Websites with Selenium and Puppeteer
Discover how to scrape JavaScript-heavy websites using Selenium and Puppeteer. This guide provides insights and code examples for handling dynamic content and extracting valuable data from web pages.
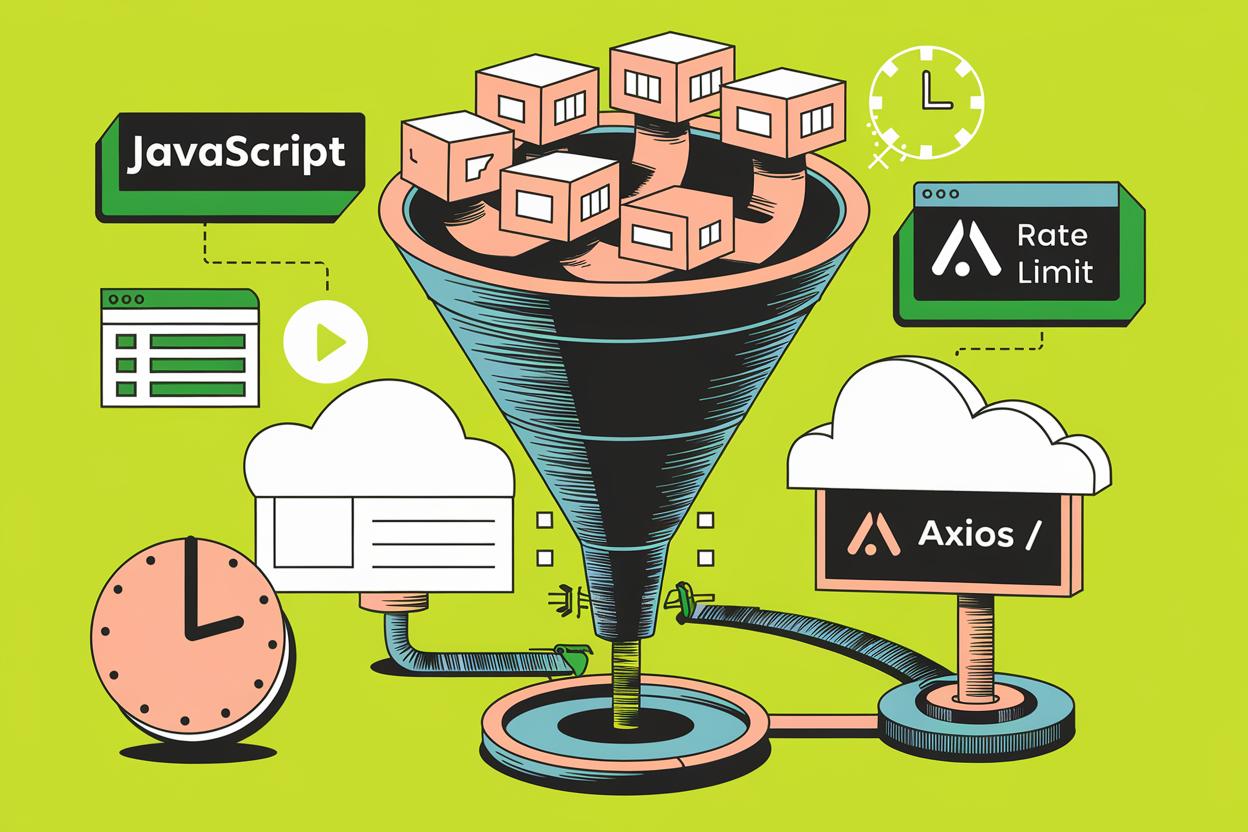
Handling API Rate Limits: Queueing API Requests with JavaScript
Learn how to manage API rate limits in JavaScript by queueing API requests. This guide covers the importance of rate limiting, use cases, and a practical example to get you started.