Handling State Management in React: A Guide to Redux and Context API
By Kainat Chaudhary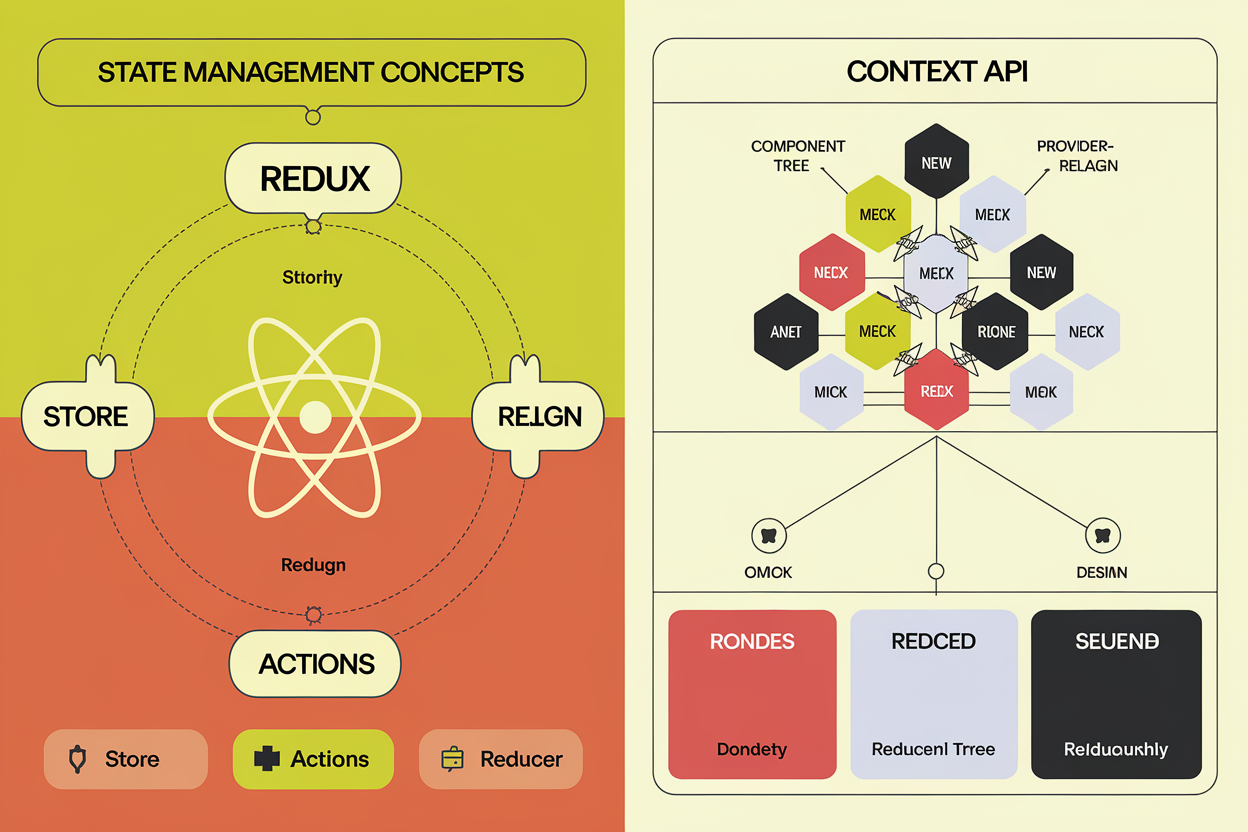
Introduction
State management is a crucial aspect of building robust and maintainable React applications. As applications grow in complexity, managing state across components becomes increasingly challenging. Two popular solutions for managing state in React are Redux and Context API. Both offer distinct approaches to handling state, and understanding their differences, benefits, and use cases can help developers choose the right tool for their needs. In this guide, we'll explore Redux and Context API, how they work, and when to use each for effective state management in React applications.
1. What is Redux?
Redux is a predictable state container for JavaScript applications. It centralizes the state of your application in a single store, allowing components to access and update state in a controlled manner. Redux follows a strict unidirectional data flow, making it easier to understand how state changes over time. Redux is ideal for complex applications with a lot of shared or deeply nested state, where you need a predictable way to manage state changes across various parts of the app.
2. Core Concepts of Redux
- Store: The single source of truth that holds the state of your application.
- Actions: Plain JavaScript objects that describe what happened in the app. Actions are dispatched to trigger state changes.
- Reducers: Pure functions that take the current state and an action as arguments and return a new state based on the action type.
- Middleware: Functions that extend Redux's capabilities, allowing you to intercept actions or perform side effects like API calls.
3. Setting Up Redux in a React App
To use Redux in a React application, you'll need to install Redux and React-Redux, the official bindings for integrating Redux with React. Here's a basic setup example:
import { createStore } from 'redux';
import { Provider } from 'react-redux';
import rootReducer from './reducers';
import App from './App';
const store = createStore(rootReducer);
const Root = () => (
<Provider store={store}>
<App />
</Provider>
);
export default Root;
4. What is Context API?
The Context API is a React feature that allows you to share state across the component tree without passing props down manually at every level. It's a simpler solution for state management compared to Redux, making it ideal for smaller applications or when state is not too deeply nested. Context API provides a way to create global state that can be accessed from any component within the Context Provider, reducing the need for prop drilling.
5. Using Context API in React
To use Context API, you create a context, provide it at a high level in your component tree, and consume it in any component that needs access to the state. Here's an example setup:
import React, { createContext, useContext, useState } from 'react';
const MyContext = createContext();
const MyProvider = ({ children }) => {
const [state, setState] = useState(initialState);
return (
<MyContext.Provider value={{ state, setState }}>
{children}
</MyContext.Provider>
);
};
const MyComponent = () => {
const { state, setState } = useContext(MyContext);
return (
<div>
<p>{state.someValue}</p>
<button onClick={() => setState({ someValue: 'New Value' })}>Change Value</button>
</div>
);
};
6. Comparing Redux and Context API
- Redux Pros: Suitable for large-scale applications with complex state management needs; offers a predictable state flow; great for debugging with tools like Redux DevTools.
- Redux Cons: Can be complex and verbose; involves boilerplate code and setup.
- Context API Pros: Simple to use and set up; eliminates the need for prop drilling; built directly into React.
- Context API Cons: May cause performance issues if not used carefully; less suitable for very complex state management.
7. When to Use Redux vs. Context API
Choose Redux if your application requires advanced state management, has a large or deeply nested state, or needs middleware for handling side effects like API calls. Redux is also a good choice if you want strong tooling support for debugging and time-travel debugging capabilities. Use Context API for simpler state management needs, when the state is localized to a few components, or when you want to avoid the boilerplate associated with Redux. It’s perfect for managing themes, user authentication, or other small pieces of state that don't require complex logic.
Conclusion
Both Redux and Context API provide powerful tools for managing state in React applications. Understanding their strengths and use cases can help you decide which one is the best fit for your project. By using Redux, you gain a robust and predictable state management solution suitable for complex applications. Meanwhile, Context API offers a simpler and more integrated approach, perfect for smaller projects or less complex state needs. Evaluate your application’s requirements, and choose the state management solution that best aligns with your development goals.
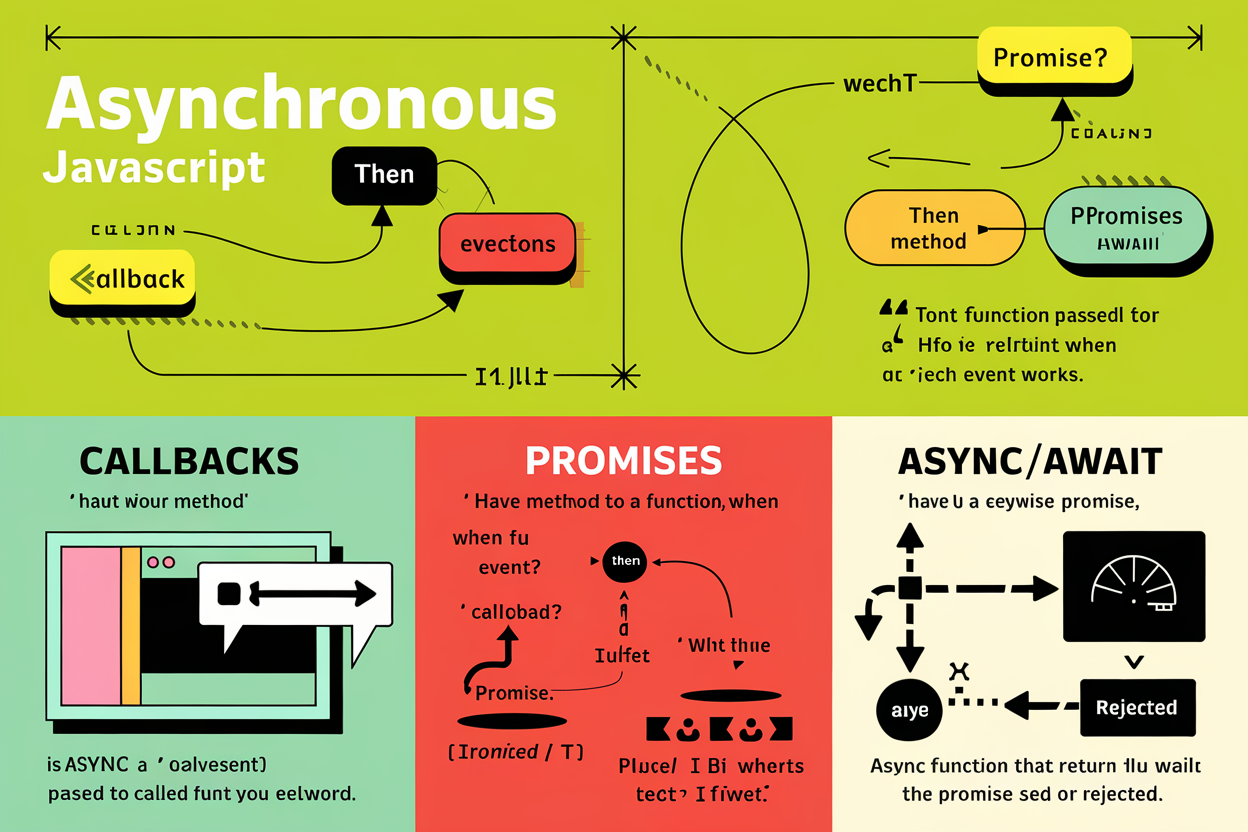
Asynchronous JavaScript: Mastering Promises, Async/Await, and Callbacks
Dive into asynchronous JavaScript with this guide to mastering Promises, async/await, and callbacks. Learn how to handle asynchronous operations efficiently and improve your coding skills.
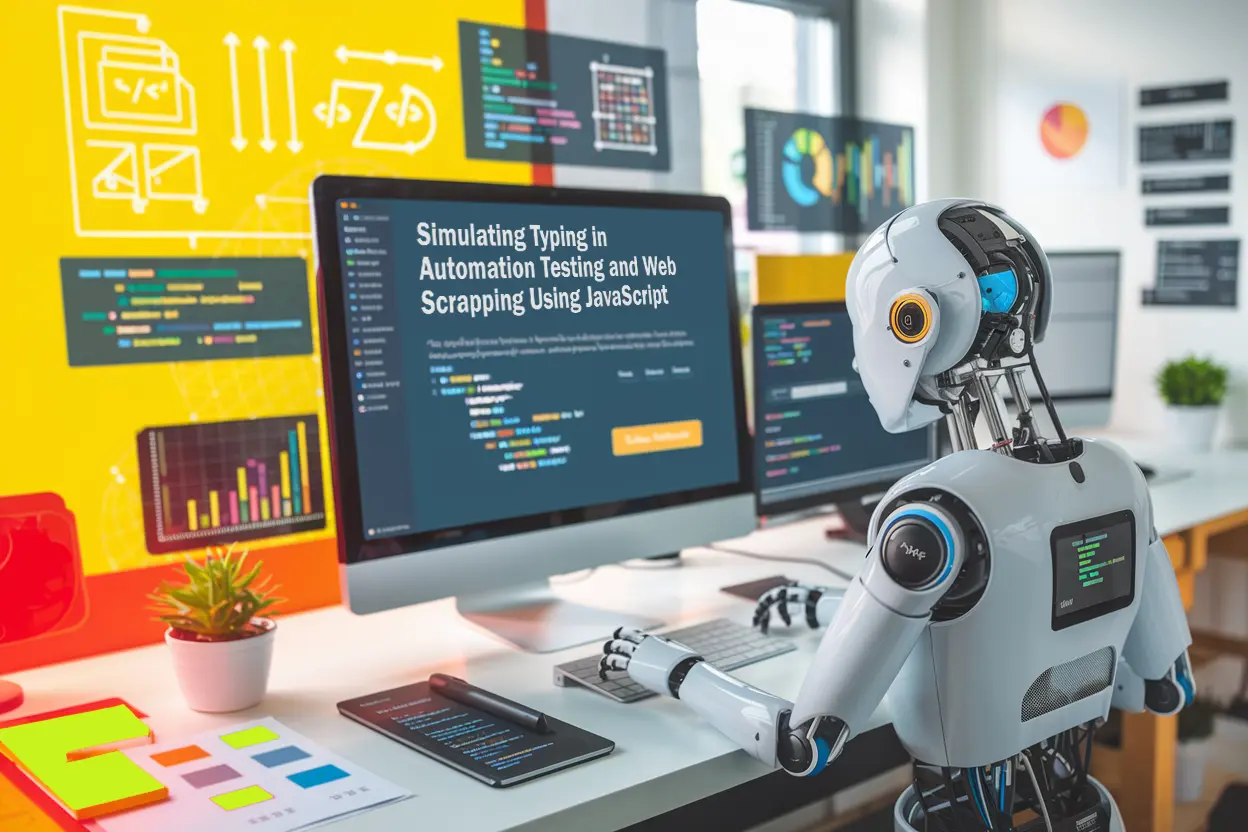
Automating User Interaction: Simulating Typing in Automation Testing and Web Scraping
Learn how to simulate typing in automation testing and web scraping using JavaScript. This guide explores the benefits and use cases of simulating user interactions and mimicking real typing events.
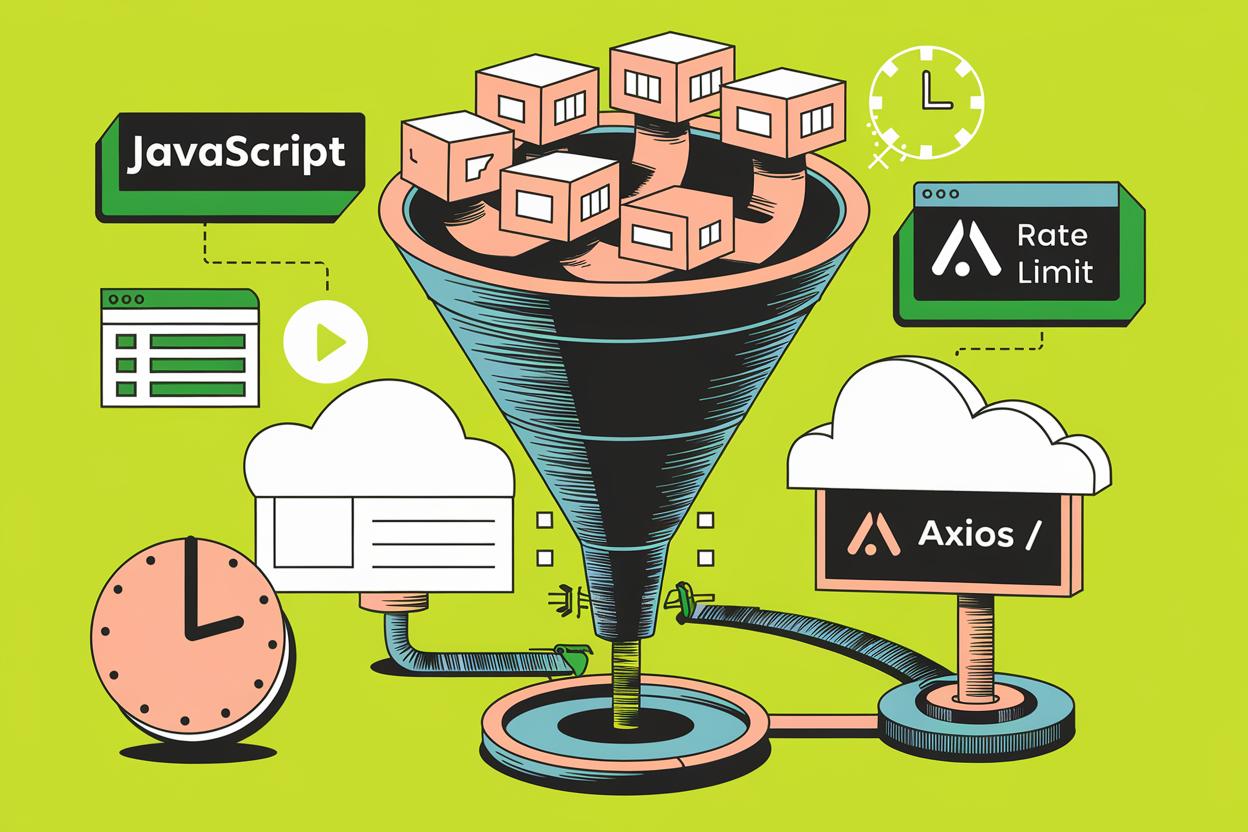
Handling API Rate Limits: Queueing API Requests with JavaScript
Learn how to manage API rate limits in JavaScript by queueing API requests. This guide covers the importance of rate limiting, use cases, and a practical example to get you started.
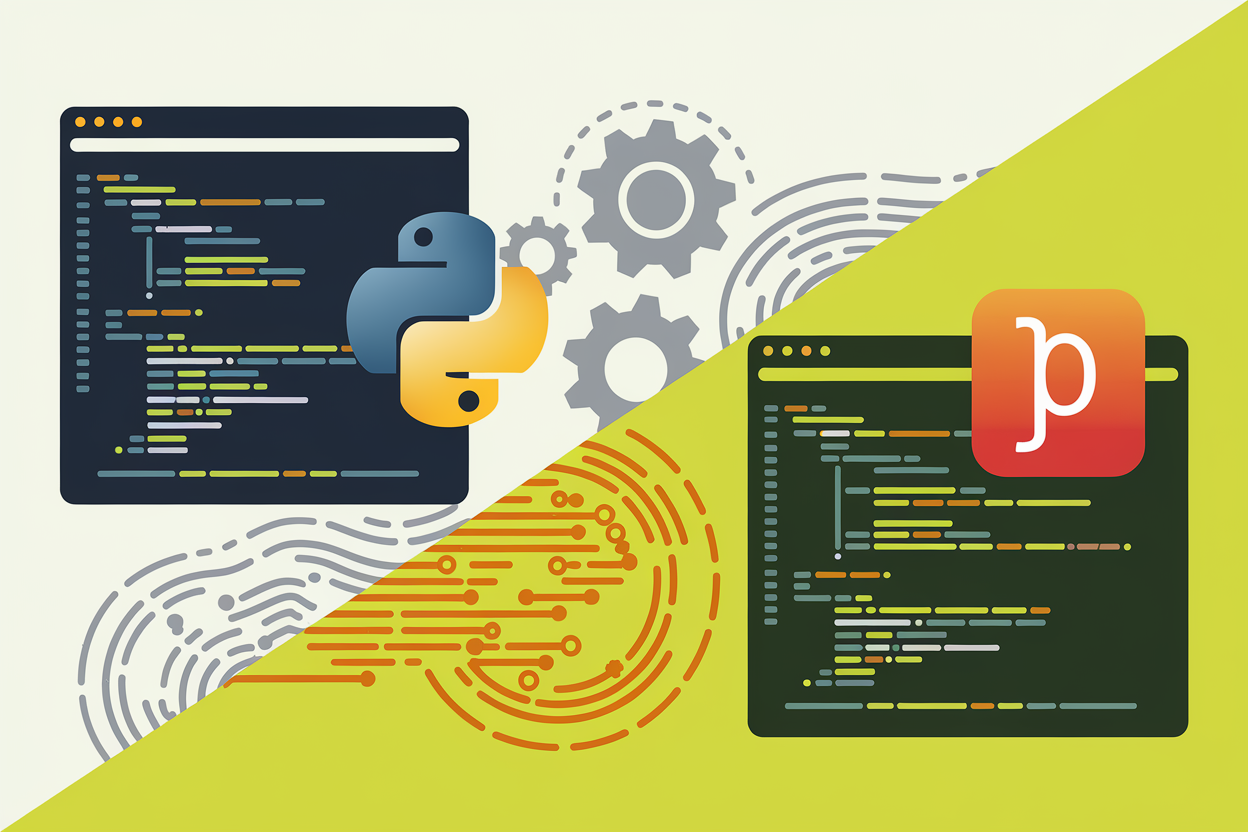
Automating Repetitive Tasks: Using Python and JavaScript for Web Automation
Learn how to automate repetitive tasks using Python and JavaScript. This guide covers automation with Selenium in Python and Puppeteer in JavaScript, providing examples and best practices for effective web automation.
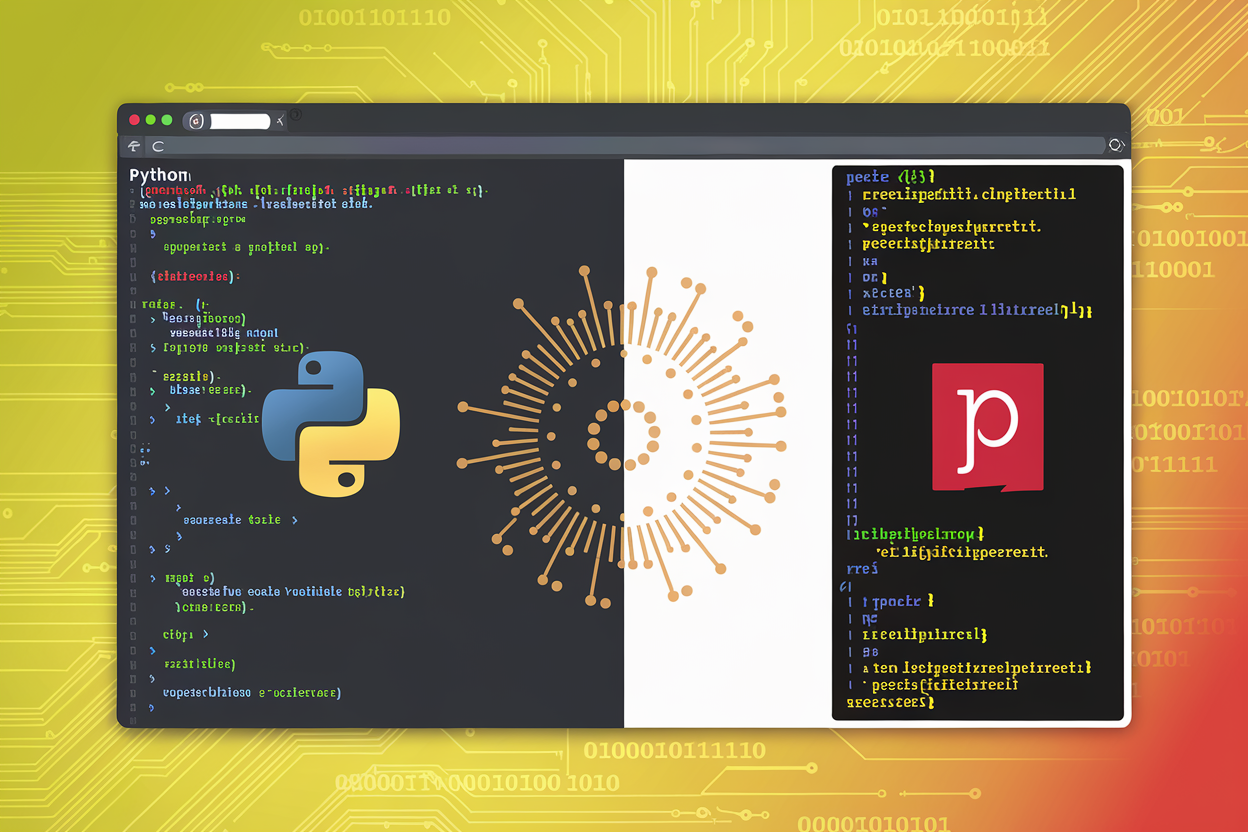
Handling Dynamic Content: Scraping JavaScript-Heavy Websites with Selenium and Puppeteer
Discover how to scrape JavaScript-heavy websites using Selenium and Puppeteer. This guide provides insights and code examples for handling dynamic content and extracting valuable data from web pages.