Asynchronous JavaScript: Mastering Promises, Async/Await, and Callbacks
By Kainat Chaudhary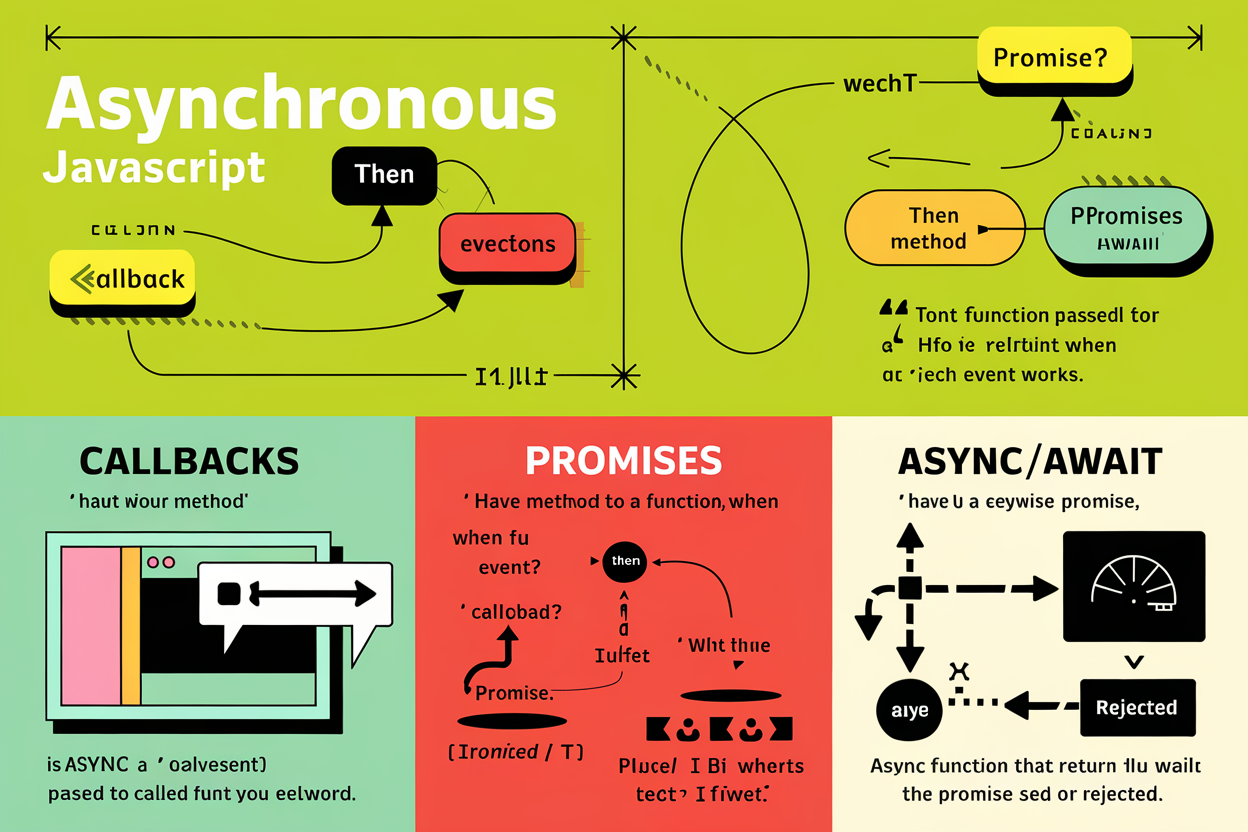
Introduction
Asynchronous programming is a cornerstone of modern JavaScript, enabling developers to handle time-consuming operations like data fetching, file reading, and user interactions without blocking the main thread. By mastering asynchronous concepts such as Promises, async/await, and callbacks, you can create more efficient and responsive applications. In this article, we'll dive deep into these asynchronous patterns, explore how they work, and learn how to use them effectively.
Understanding Callbacks
Callbacks are one of the earliest and most fundamental ways to handle asynchronous operations in JavaScript. A callback is simply a function passed as an argument to another function, which is then executed after the completion of an asynchronous task.
While callbacks are straightforward, they can lead to issues like 'callback hell' when nested too deeply, making the code hard to read and maintain. Despite this, callbacks are still widely used, especially in legacy codebases and some APIs.
function fetchData(callback) {
setTimeout(() => {
callback('Data fetched!');
}, 1000);
}
fetchData((message) => {
console.log(message);
});
Introducing Promises
Promises provide a more powerful and flexible way to manage asynchronous operations in JavaScript. A Promise represents an operation that hasn't completed yet but is expected to in the future. Promises have three states: pending, fulfilled, and rejected. They allow you to chain asynchronous actions, making the code easier to follow compared to nested callbacks.
Using `.then()`, `.catch()`, and `.finally()` methods, Promises handle successful results, errors, and cleanup operations, respectively. Promises simplify the handling of multiple asynchronous tasks and help avoid the deeply nested structures of callbacks.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Data fetched with Promise!');
}, 1000);
});
}
fetchData()
.then((message) => console.log(message))
.catch((error) => console.error(error));
Mastering Async/Await
Async/await is a more modern approach built on top of Promises, allowing you to write asynchronous code that looks and behaves like synchronous code. By using the `async` keyword before a function, it automatically returns a Promise. The `await` keyword pauses the execution of an async function until the Promise is fulfilled, making the code much more readable and easier to understand.
Async/await simplifies complex promise chains and makes it easier to handle errors using standard `try` and `catch` blocks. This pattern is highly favored in modern JavaScript development for its readability and simplicity.
async function fetchData() {
try {
const message = await new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Data fetched with Async/Await!');
}, 1000);
});
console.log(message);
} catch (error) {
console.error(error);
}
}
fetchData();
Choosing the Right Approach
When deciding between callbacks, Promises, and async/await, consider the complexity of the task and the readability of your code. Callbacks are simple but can lead to messy code when used extensively. Promises offer a cleaner and more manageable way to handle asynchronous tasks, while async/await takes it a step further by making asynchronous code look synchronous.
- Use Callbacks for simple, single-step asynchronous operations where readability isn't a concern.
- Opt for Promises when dealing with multiple asynchronous tasks that need to be chained together.
- Choose Async/Await for the best readability, especially when working with multiple Promises and needing clear, sequential execution.
Conclusion
Mastering asynchronous JavaScript with callbacks, Promises, and async/await is essential for building efficient and responsive applications. Each approach has its strengths and is suited to different scenarios. By understanding these patterns, you can choose the right tools for your project, write cleaner code, and create better user experiences.
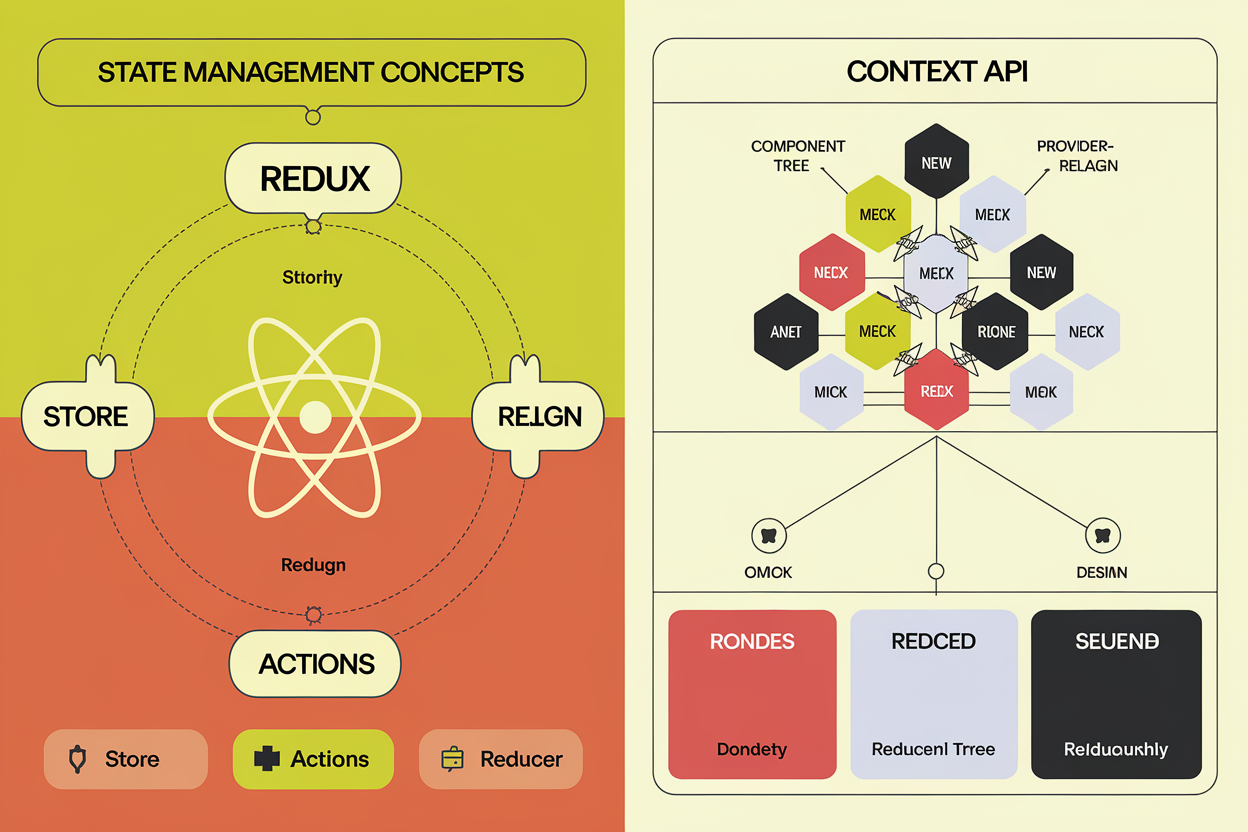
Handling State Management in React: A Guide to Redux and Context API
Learn how to handle state management in React with Redux and Context API. This guide explores the differences, benefits, and use cases of each approach, helping you choose the best solution for your React applications.
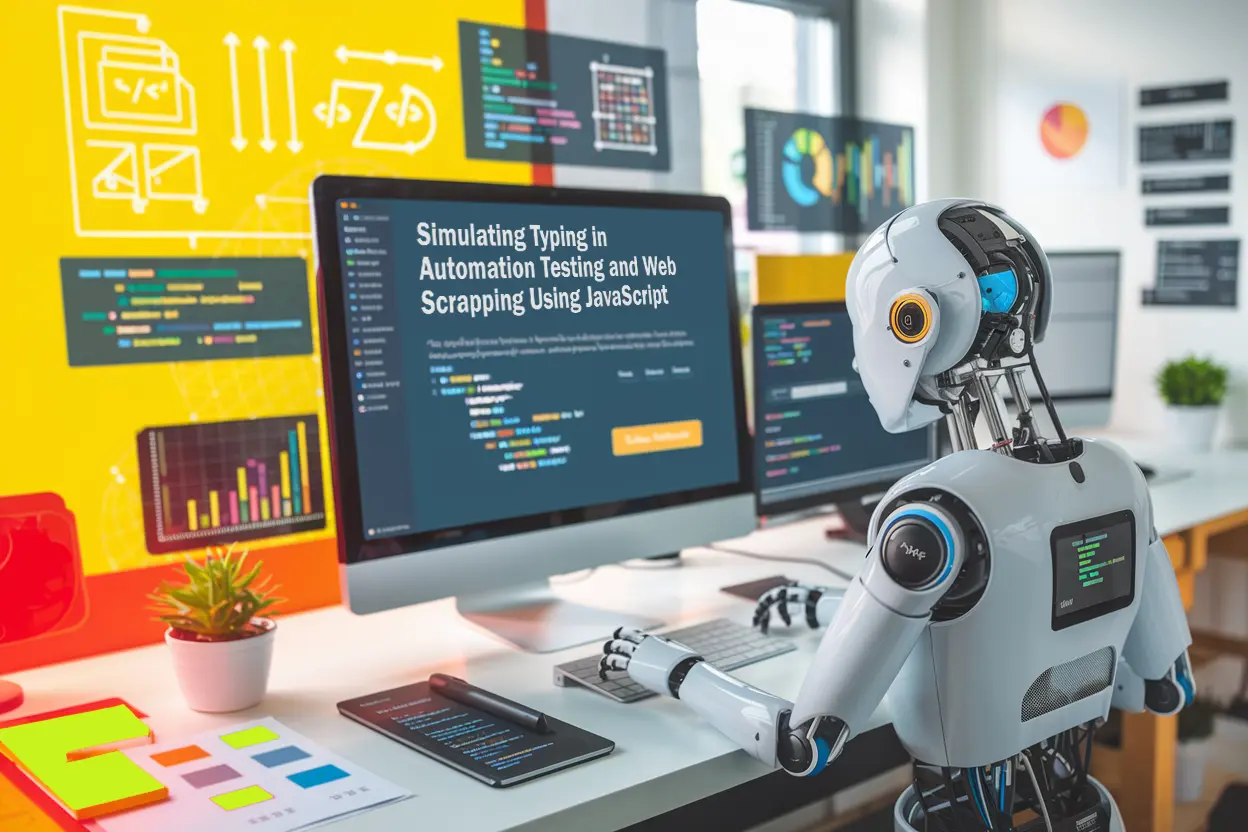
Automating User Interaction: Simulating Typing in Automation Testing and Web Scraping
Learn how to simulate typing in automation testing and web scraping using JavaScript. This guide explores the benefits and use cases of simulating user interactions and mimicking real typing events.
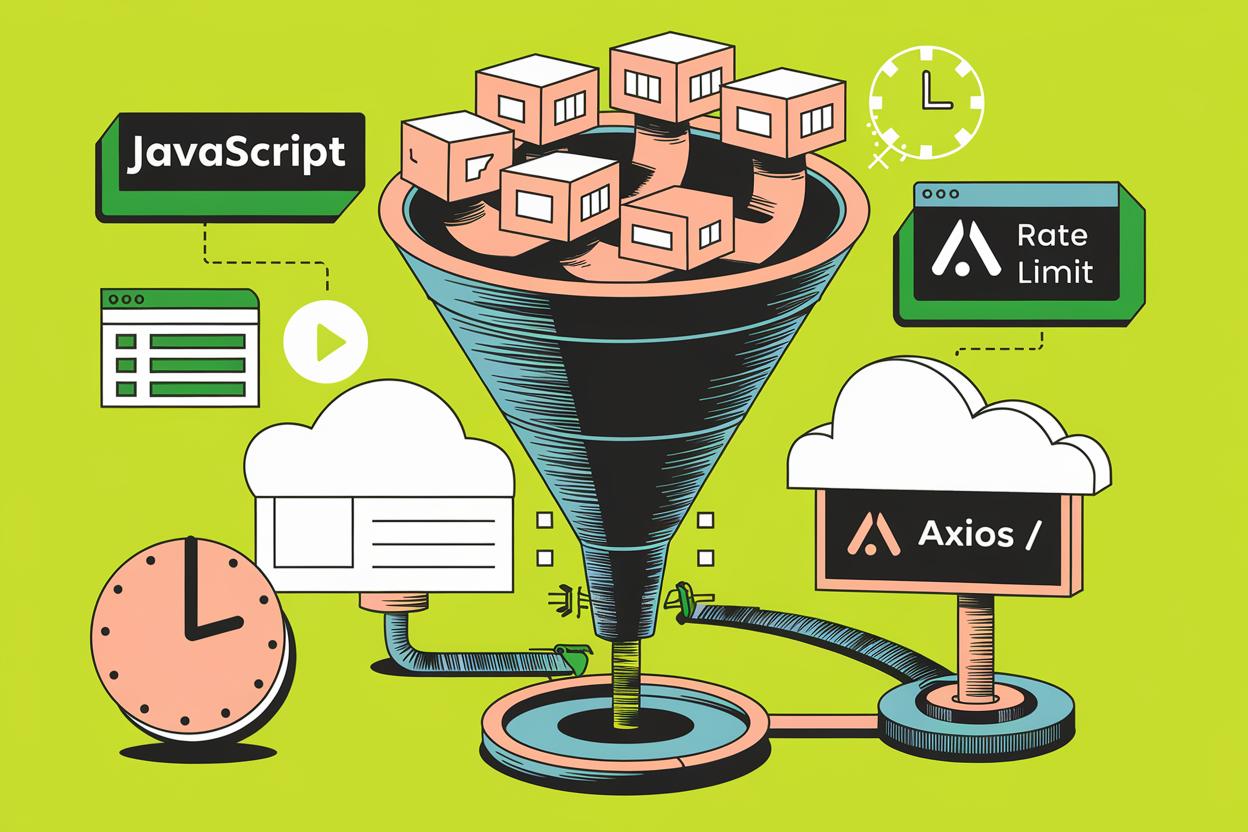
Handling API Rate Limits: Queueing API Requests with JavaScript
Learn how to manage API rate limits in JavaScript by queueing API requests. This guide covers the importance of rate limiting, use cases, and a practical example to get you started.
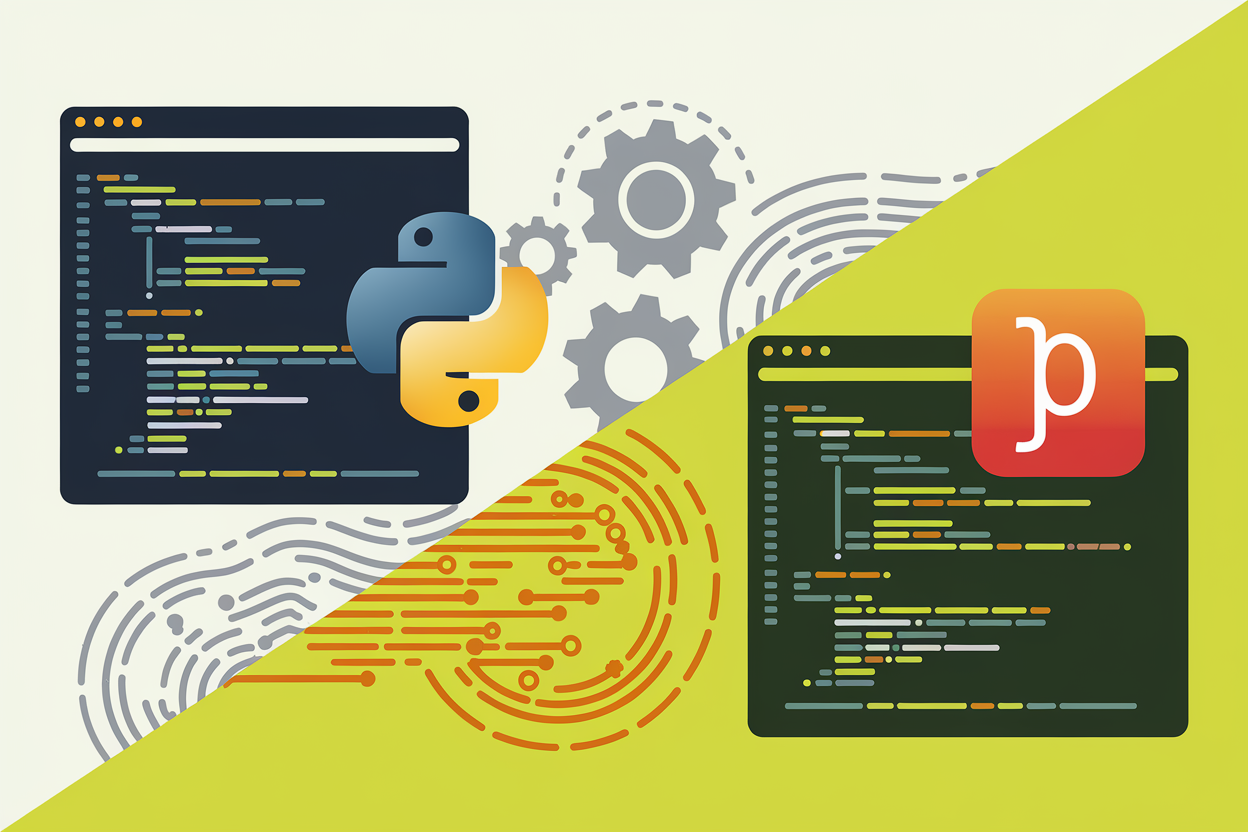
Automating Repetitive Tasks: Using Python and JavaScript for Web Automation
Learn how to automate repetitive tasks using Python and JavaScript. This guide covers automation with Selenium in Python and Puppeteer in JavaScript, providing examples and best practices for effective web automation.