Understanding CORS: Cross-Origin Resource Sharing
By Kainat Chaudhary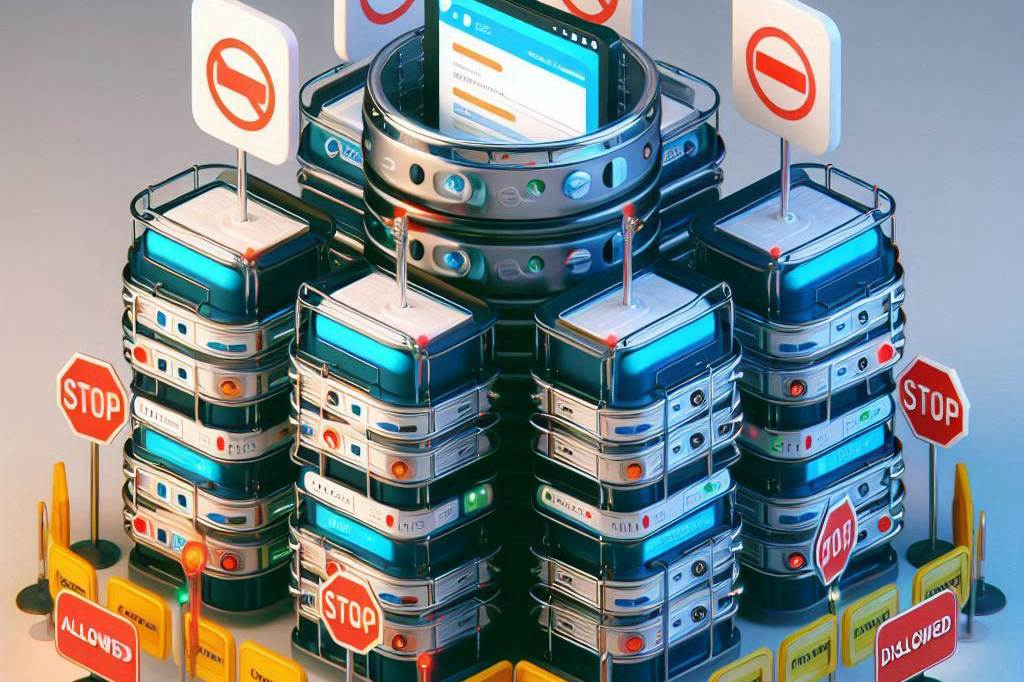
As a web developer, ensuring secure and efficient communication between different origins is crucial. Cross-Origin Resource Sharing (CORS) is a vital concept that helps manage this interaction securely. In this post, I'll delve into what CORS is, why it’s important, and how to implement it effectively in your web applications.
What is CORS?
CORS stands for Cross-Origin Resource Sharing. It is a security feature implemented by browsers to prevent malicious websites from making requests to a different domain than the one that served the original web page. Essentially, it is a mechanism that allows a server to specify who can access its resources and how they can be accessed.
"CORS is a relaxation of the same-origin policy implemented by browsers."
Why is CORS Important?
CORS is essential for the security and integrity of web applications. Without CORS, a malicious website could potentially make unauthorized requests to a different website, accessing sensitive data or performing actions on behalf of the user. By enforcing CORS, browsers ensure that only authorized domains can access resources, protecting users from cross-origin attacks such as Cross-Site Request Forgery (CSRF).
- Prevents unauthorized access
- Protects against cross-origin attacks
- Ensures secure communication
How Does CORS Work?
CORS works by adding HTTP headers to responses from the server. These headers indicate whether the requesting origin is allowed to access the resource. The key headers involved in CORS are:
- Access-Control-Allow-Origin: Specifies the allowed origin(s).
- Access-Control-Allow-Methods: Lists the HTTP methods that can be used.
- Access-Control-Allow-Headers: Lists the headers that can be used.
- Access-Control-Allow-Credentials: Indicates whether credentials are allowed.
Implementing CORS
Implementing CORS in your web application involves configuring your server to send the appropriate headers. Here’s an example of how to enable CORS in a Node.js application using the Express framework:
const express = require('express');
const cors = require('cors');
const app = express();
const corsOptions = {
origin: 'http://example.com', // specify the allowed origin
methods: 'GET,POST,PUT,DELETE', // specify allowed methods
allowedHeaders: 'Content-Type,Authorization', // specify allowed headers
credentials: true // allow credentials
};
app.use(cors(corsOptions));
app.get('/data', (req, res) => {
res.json({ message: 'This is CORS-enabled data.' });
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Handling Preflight Requests
For certain types of requests, such as those with custom headers or HTTP methods other than GET and POST, browsers send a preflight request. This is an OPTIONS request sent to the server to check if the actual request is safe to send. The server must respond with the appropriate CORS headers to allow the actual request.
app.options('/data', (req, res) => {
res.header('Access-Control-Allow-Origin', 'http://example.com');
res.header('Access-Control-Allow-Methods', 'GET,POST,PUT,DELETE');
res.header('Access-Control-Allow-Headers', 'Content-Type,Authorization');
res.send();
});
Best Practices for CORS
To ensure secure and efficient implementation of CORS, follow these best practices:
- Only allow trusted origins.
- Restrict allowed methods and headers.
- Use credentials only when necessary.
- Regularly review and update CORS settings.
Understanding and implementing CORS is crucial for the security and functionality of web applications. By properly configuring CORS headers, you can ensure secure cross-origin communication while protecting your users and resources. Whether you're building a new application or enhancing an existing one, make sure to integrate CORS to safeguard your web interactions.
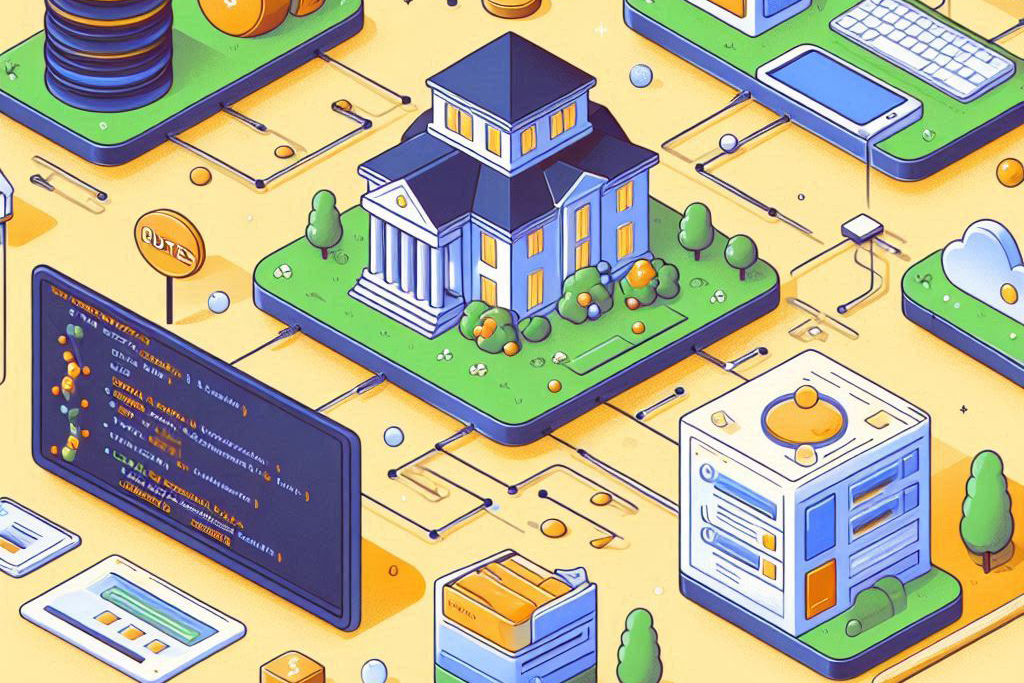
Building a RESTful API with Node.js and Express: A Step-by-Step Tutorial
Learn how to build a RESTful API using Node.js and Express in this step-by-step tutorial. From setting up the project to defining routes and handling errors, discover how to create a robust and scalable API.
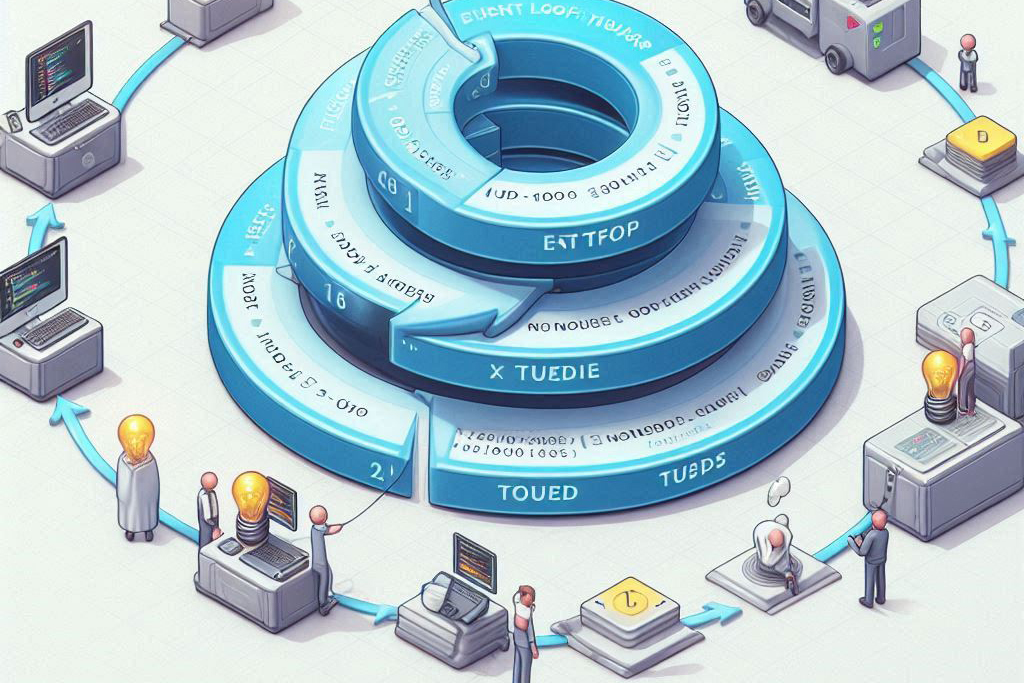
Understanding the Event Loop in Node.js: How It Works and Why It Matters
Explore the concept of the event loop in Node.js, its operation, significance, and best practices for efficient asynchronous programming. Learn how the event loop contributes to Node.js’s performance and scalability.
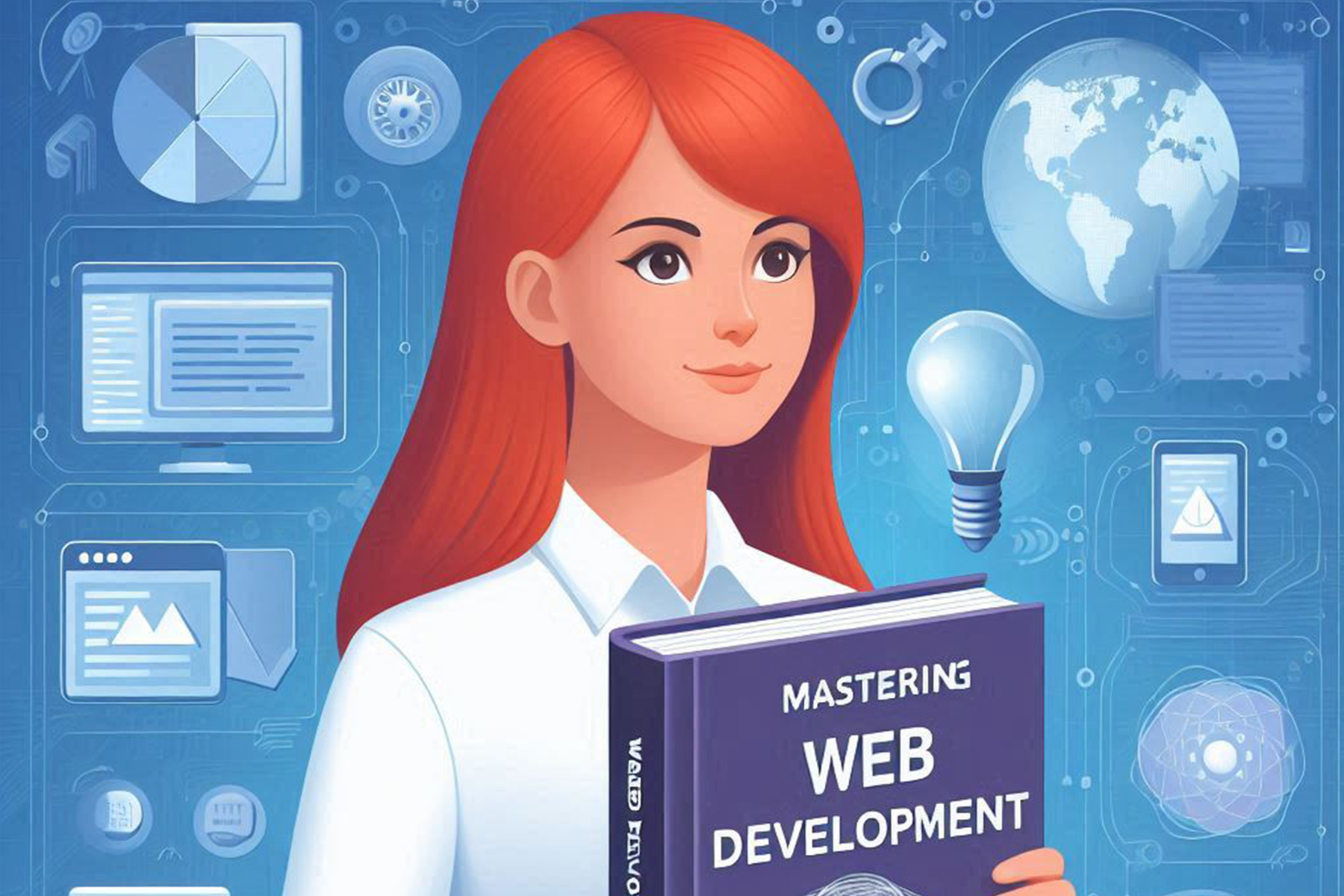
Mastering Web Development: Essential Skills and Best Practices for Modern Web Developers
Explore the core skills and best practices that every web developer should master. Learn how to build efficient, scalable, and user-friendly web applications by staying up-to-date with the latest technologies and methodologies.
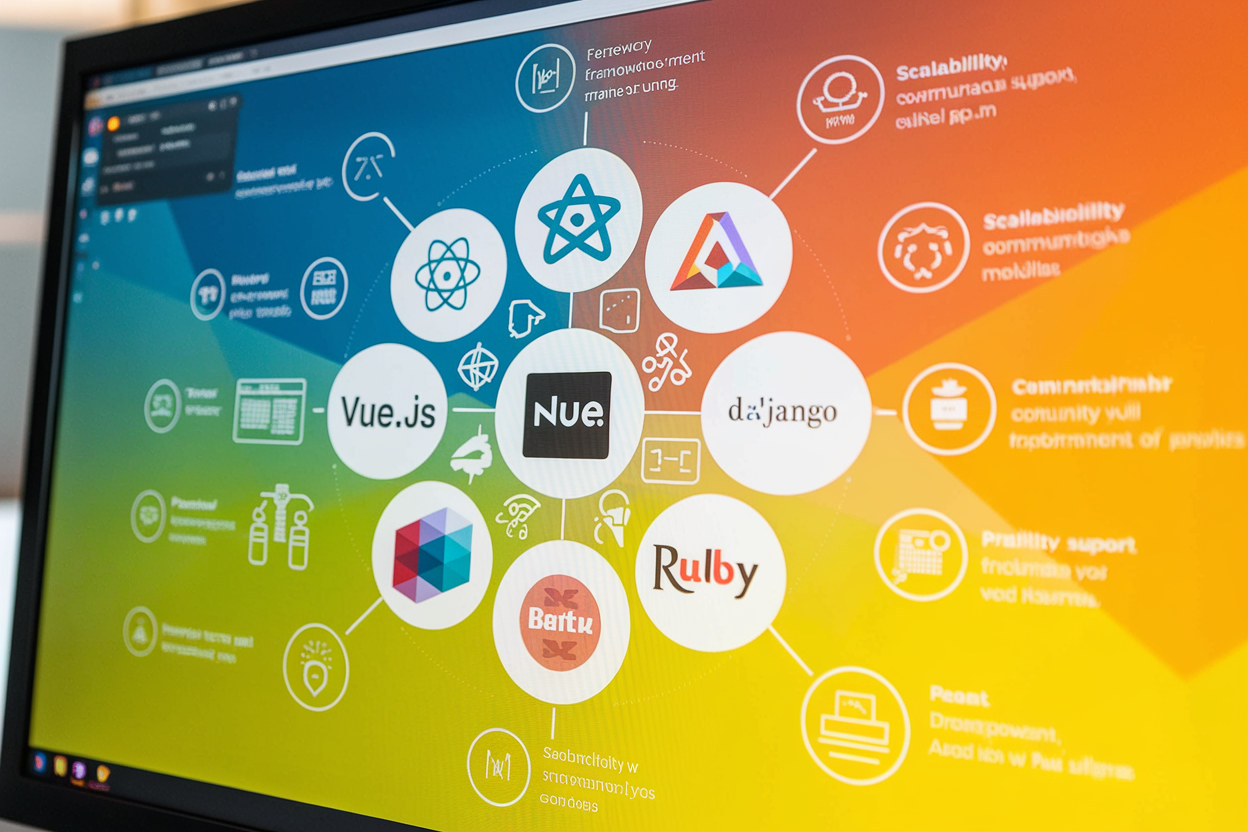
Web Development Frameworks: Choosing the Right One for Your Project
Discover the essential factors to consider when selecting a web development framework for your project. Explore popular frameworks and their unique features to make an informed choice.