Building a RESTful API with Node.js and Express: A Step-by-Step Tutorial
By Kainat Chaudhary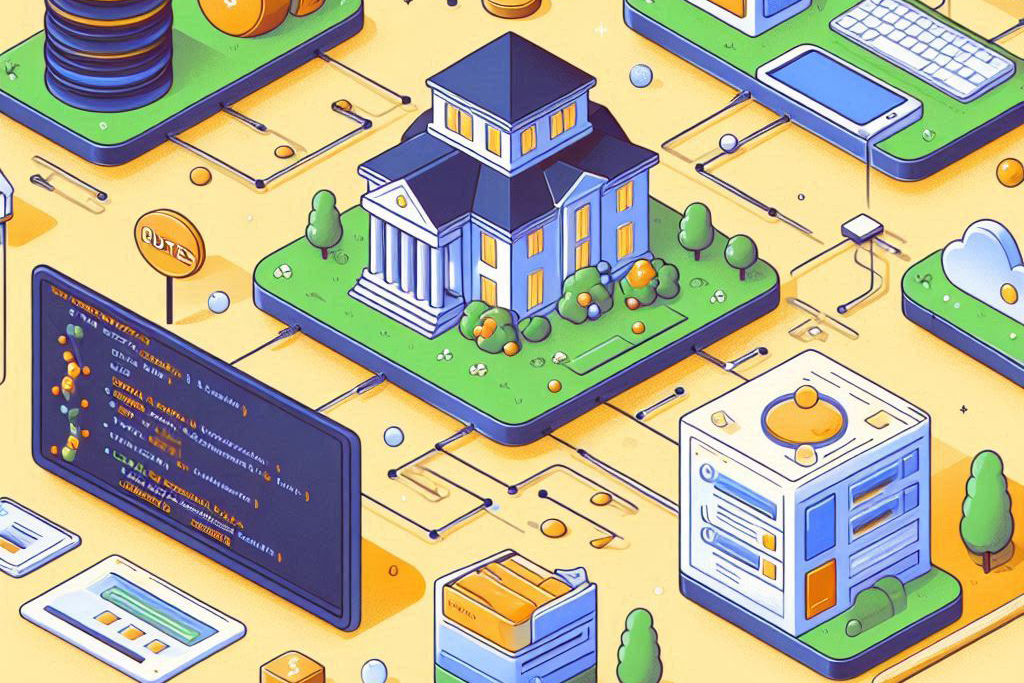
Creating a RESTful API is a fundamental skill for modern web development. Node.js, paired with the Express framework, provides a powerful and flexible platform for building APIs. In this step-by-step tutorial, we'll walk through the process of creating a simple RESTful API using Node.js and Express, covering everything from setting up the project to handling requests and responses.
Step 1: Setting Up Your Project
First, you'll need to set up your project and install the necessary dependencies. Start by creating a new directory for your project and initializing a new Node.js project using npm.
mkdir my-api
cd my-api
npm init -y
Next, install Express, a minimal and flexible Node.js web application framework, to handle routing and middleware.
npm install express
Step 2: Creating the Basic Server
Create a file named `server.js` in your project directory. This file will contain the basic setup for your Express server.
const express = require('express');
const app = express();
const port = 3000;
app.use(express.json());
app.get('/', (req, res) => {
res.send('Hello, world!');
});
app.listen(port, () => {
console.log(`Server running at http://localhost:${port}/`);
});
In this setup, we create an Express application, configure it to parse JSON requests, and define a simple route that returns 'Hello, world!' when accessed.
Step 3: Defining Routes and Controllers
To build a RESTful API, you'll need to define routes for handling different HTTP methods. Create a new directory called `routes` and add a file named `api.js` to define your routes.
const express = require('express');
const router = express.Router();
// Mock data
let items = [{ id: 1, name: 'Item 1' }];
// GET all items
router.get('/items', (req, res) => {
res.json(items);
});
// POST a new item
router.post('/items', (req, res) => {
const newItem = req.body;
newItem.id = items.length + 1;
items.push(newItem);
res.status(201).json(newItem);
});
module.exports = router;
In `api.js`, we define routes for getting all items and posting a new item. The routes handle requests by interacting with mock data.
Back in `server.js`, import and use this route module.
const apiRoutes = require('./routes/api');
app.use('/api', apiRoutes);
Step 4: Testing the API
With your API set up, it’s time to test it. You can use tools like Postman or curl to make requests to your API endpoints.
To test, start your server using:
node server.js
Then, use Postman to make GET and POST requests to `http://localhost:3000/api/items` and verify that your API responds as expected.
Step 5: Handling Errors and Validation
For a production-ready API, you need to handle errors and validate input. You can use middleware for error handling and validation libraries like Joi or express-validator.
Add error handling middleware in `server.js`:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
Best Practices
Here are some best practices for building RESTful APIs with Node.js and Express: - Use environment variables to manage configuration settings. - Implement authentication and authorization for secure access. - Log requests and errors for better monitoring and debugging. - Follow REST principles to maintain a consistent and intuitive API design.
Building a RESTful API with Node.js and Express is a great way to create scalable and maintainable web services. By following these steps and best practices, you can build a robust API that meets your application's needs and provides a seamless experience for users.
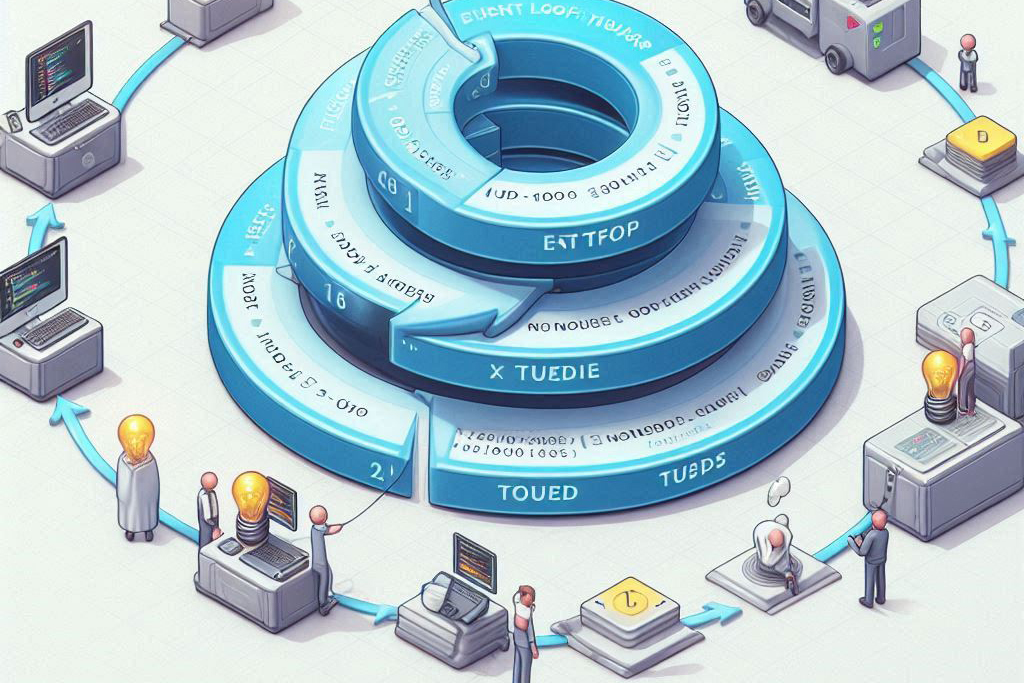
Understanding the Event Loop in Node.js: How It Works and Why It Matters
Explore the concept of the event loop in Node.js, its operation, significance, and best practices for efficient asynchronous programming. Learn how the event loop contributes to Node.js’s performance and scalability.
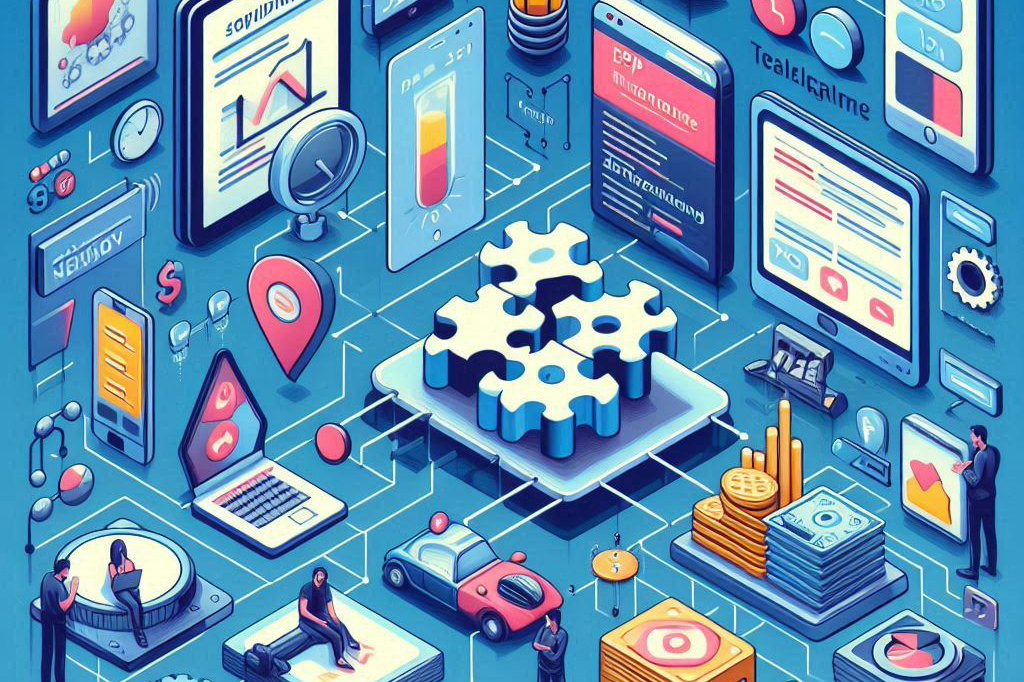
Why Node.js is Becoming Increasingly Popular: Use Cases and Benefits
Discover why Node.js is becoming increasingly popular among developers. Learn about its diverse use cases and the benefits it offers for building modern applications.
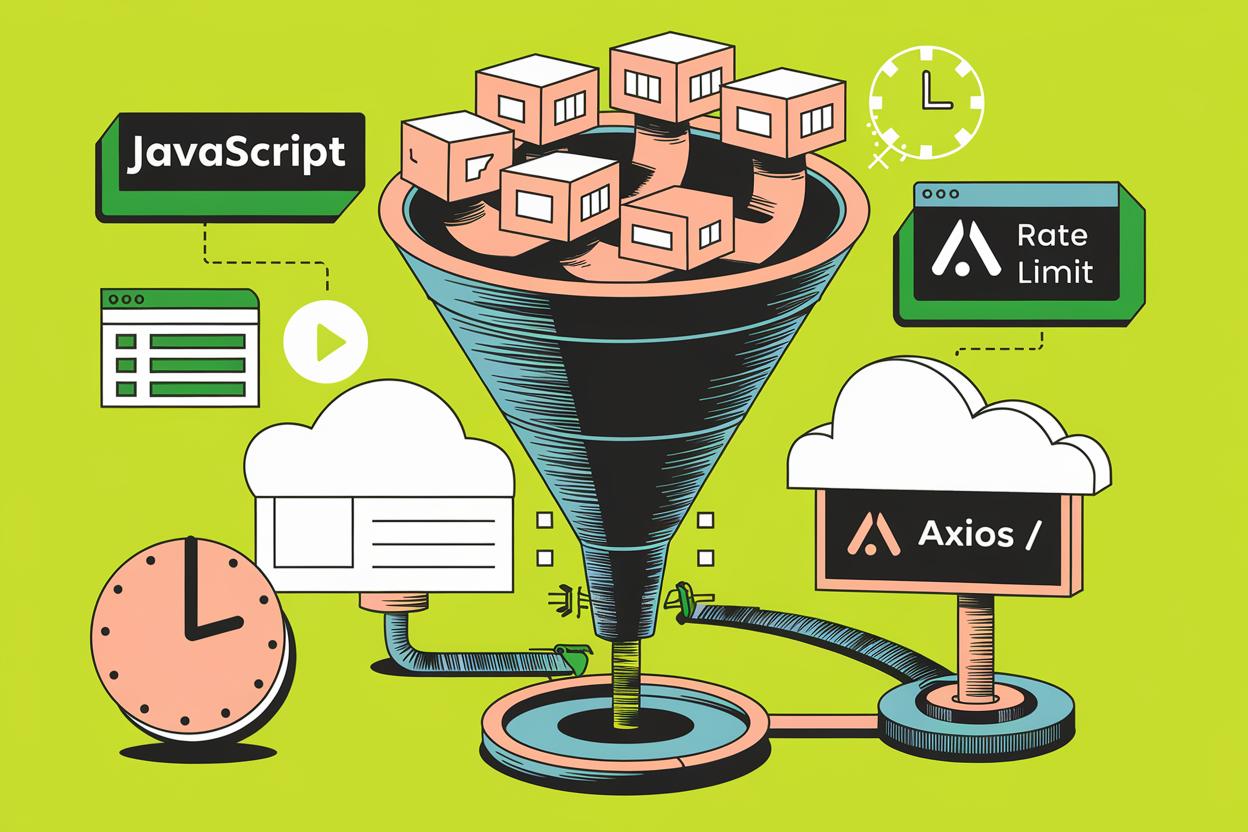
Handling API Rate Limits: Queueing API Requests with JavaScript
Learn how to manage API rate limits in JavaScript by queueing API requests. This guide covers the importance of rate limiting, use cases, and a practical example to get you started.