Optimizing Performance: Using Clusters in a Node.js App
By Kainat Chaudhary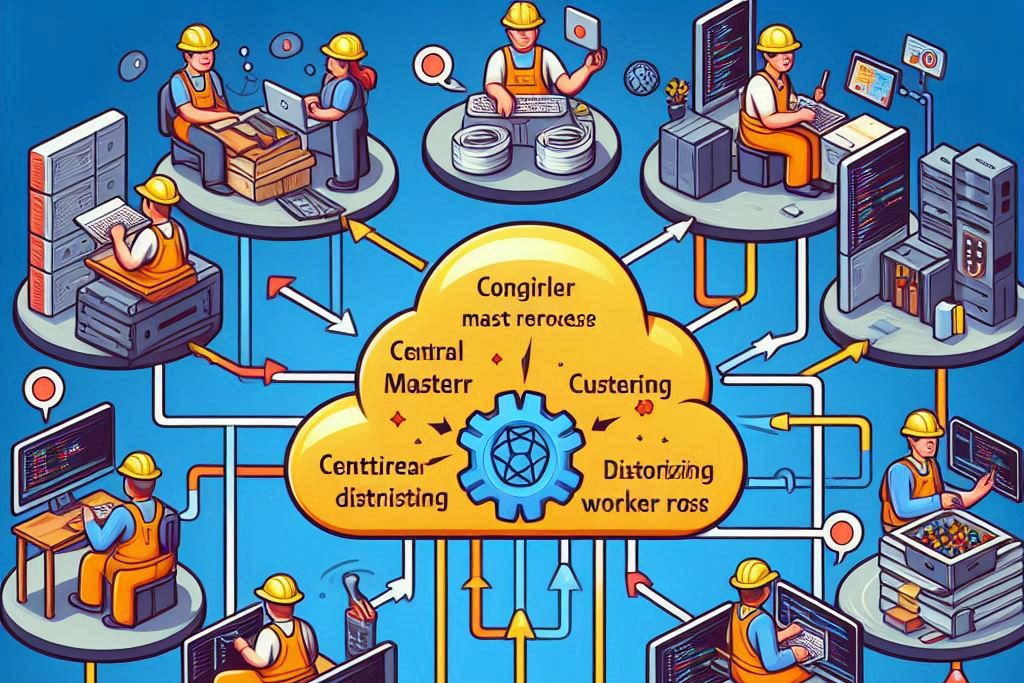
Node.js is known for its single-threaded, event-driven architecture, which makes it efficient for handling I/O operations. However, this single-threaded nature can become a bottleneck when dealing with CPU-intensive tasks. To overcome this limitation and fully utilize multi-core systems, Node.js provides a clustering module. In this post, we'll explore how to use clusters in a Node.js application to optimize performance.
What is Clustering?
Clustering allows you to create multiple instances of your Node.js application, each running in its own process. A master process manages these instances, or worker processes, and distributes incoming requests among them. This approach helps to balance the load and take advantage of multi-core systems.
Benefits of Using Clusters
- Improved performance and scalability
- Better utilization of multi-core processors
- Increased reliability and fault tolerance
- Reduced risk of single points of failure
Setting Up Clusters in Node.js
Setting up clustering in Node.js is straightforward. The cluster module provides all the necessary tools to fork worker processes and manage them. Here's a basic example of how to set up clustering in a Node.js application:
const cluster = require('cluster');
const http = require('http');
const os = require('os');
if (cluster.isMaster) {
const numCPUs = os.cpus().length;
console.log(`Master process is running. Forking ${numCPUs} workers...`);
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died. Forking a new worker...`);
cluster.fork();
});
} else {
http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello, World!');
}).listen(8000);
console.log(`Worker ${process.pid} started`);
}
Explanation
In this example, the master process forks a number of worker processes equal to the number of CPU cores. Each worker process runs a simple HTTP server. If a worker process dies, the master process forks a new worker to replace it. This ensures that the application remains resilient and can handle high loads efficiently.
Advanced Clustering Techniques
While the basic setup is useful, there are more advanced techniques to optimize clustering in Node.js:
- Load Balancing: Use a reverse proxy like Nginx to distribute requests more efficiently.
- Sticky Sessions: Ensure that user sessions are consistently handled by the same worker process.
- Graceful Shutdown: Implement graceful shutdowns to handle worker restarts without disrupting the service.
- Monitoring: Use monitoring tools to track the performance and health of each worker process.
Best Practices
To make the most out of clustering in Node.js, follow these best practices:
- Keep the master process lightweight to avoid performance bottlenecks.
- Regularly monitor and maintain worker processes to ensure optimal performance.
- Test your clustering setup thoroughly to identify and resolve potential issues.
- Consider using additional Node.js process management tools like PM2 for enhanced functionality.
Using clusters in Node.js is a powerful way to enhance the performance and scalability of your applications. By distributing the load across multiple processes, you can fully utilize the capabilities of multi-core systems and build robust, high-performing applications. Implement clustering in your Node.js projects to take advantage of these benefits and ensure your applications can handle demanding workloads.
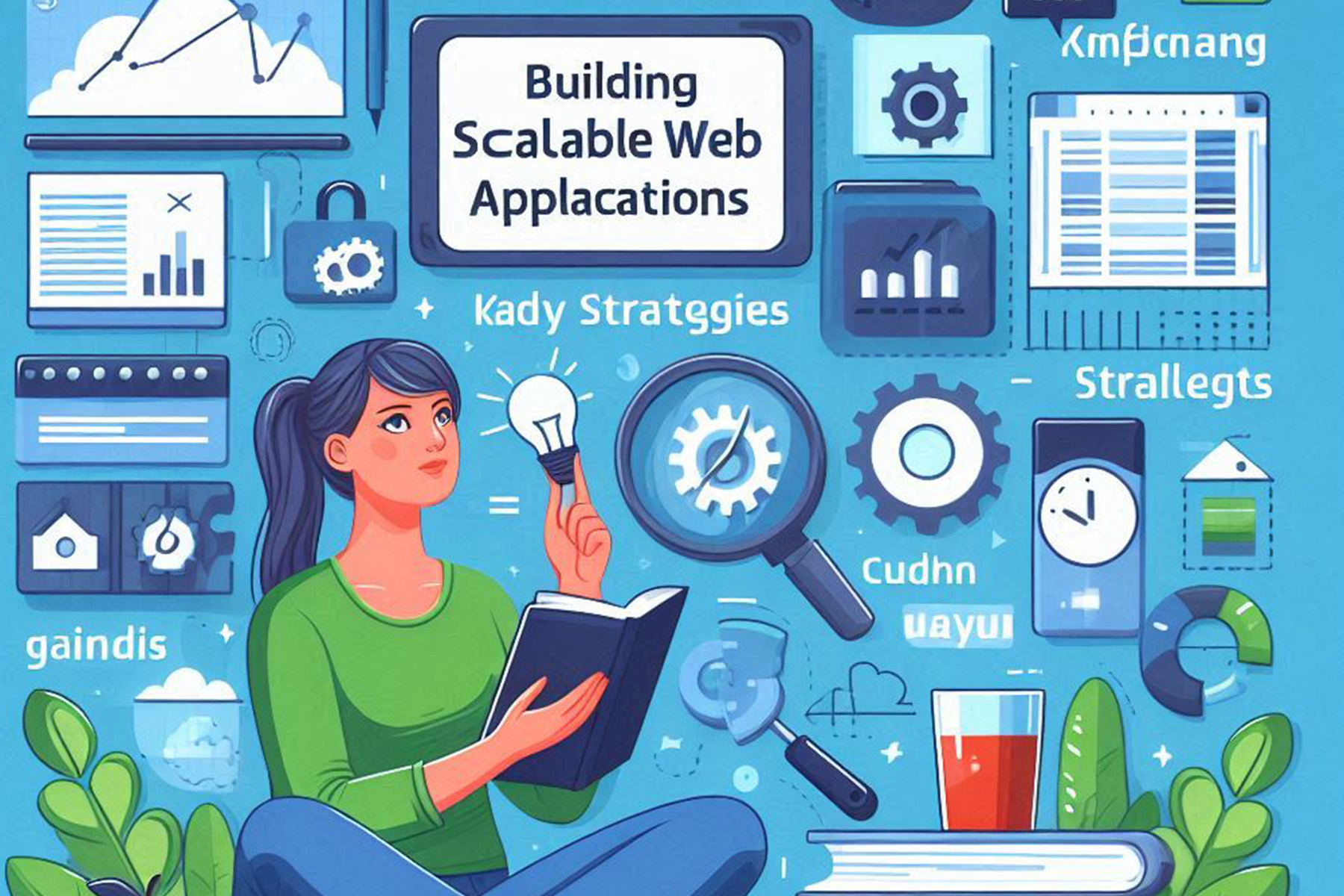
Building Scalable Web Applications: Key Strategies and Techniques
Learn how to design and build scalable web applications that can handle increasing traffic and data. Explore key strategies and techniques to ensure your web applications are robust, efficient, and capable of growing with your business.
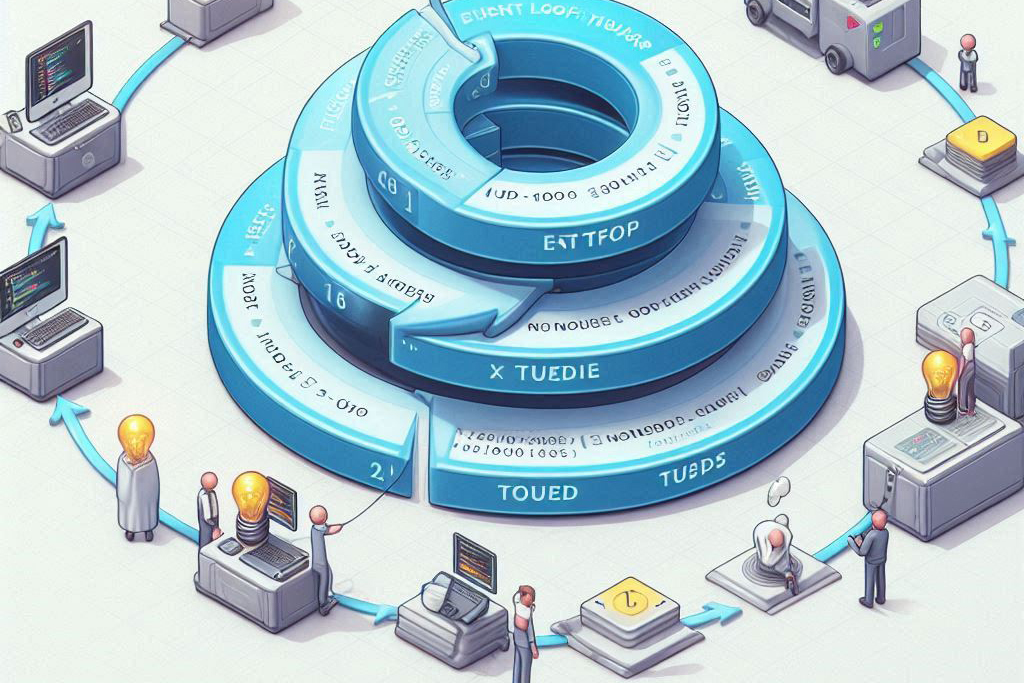
Understanding the Event Loop in Node.js: How It Works and Why It Matters
Explore the concept of the event loop in Node.js, its operation, significance, and best practices for efficient asynchronous programming. Learn how the event loop contributes to Node.js’s performance and scalability.
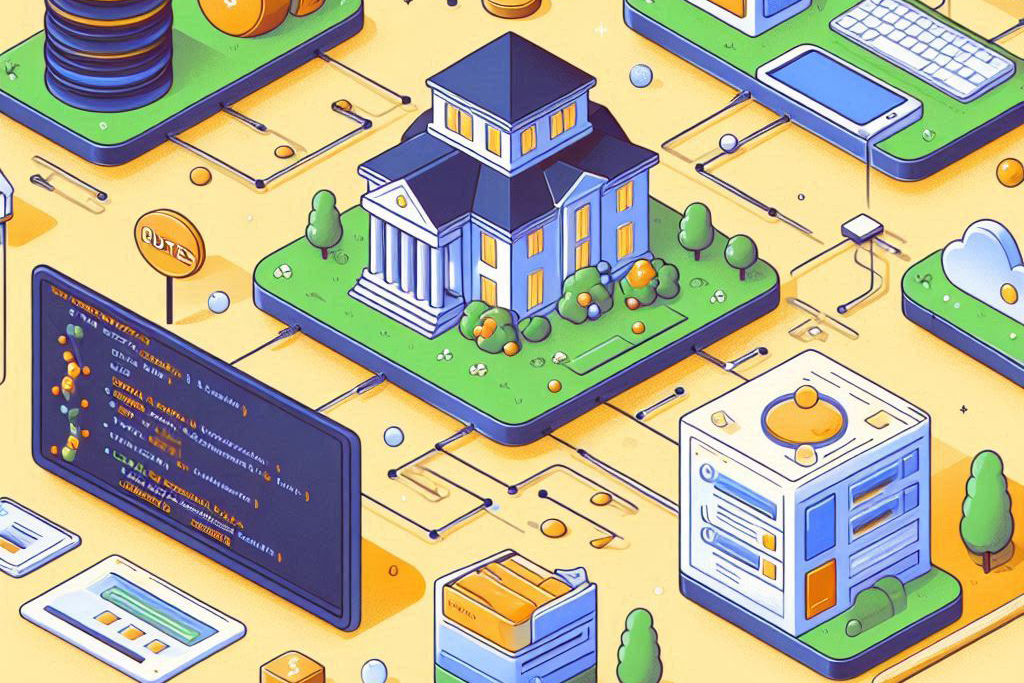
Building a RESTful API with Node.js and Express: A Step-by-Step Tutorial
Learn how to build a RESTful API using Node.js and Express in this step-by-step tutorial. From setting up the project to defining routes and handling errors, discover how to create a robust and scalable API.