How Machine Learning Algorithms Work: A Deep Dive into the Basics
By Kainat Chaudhary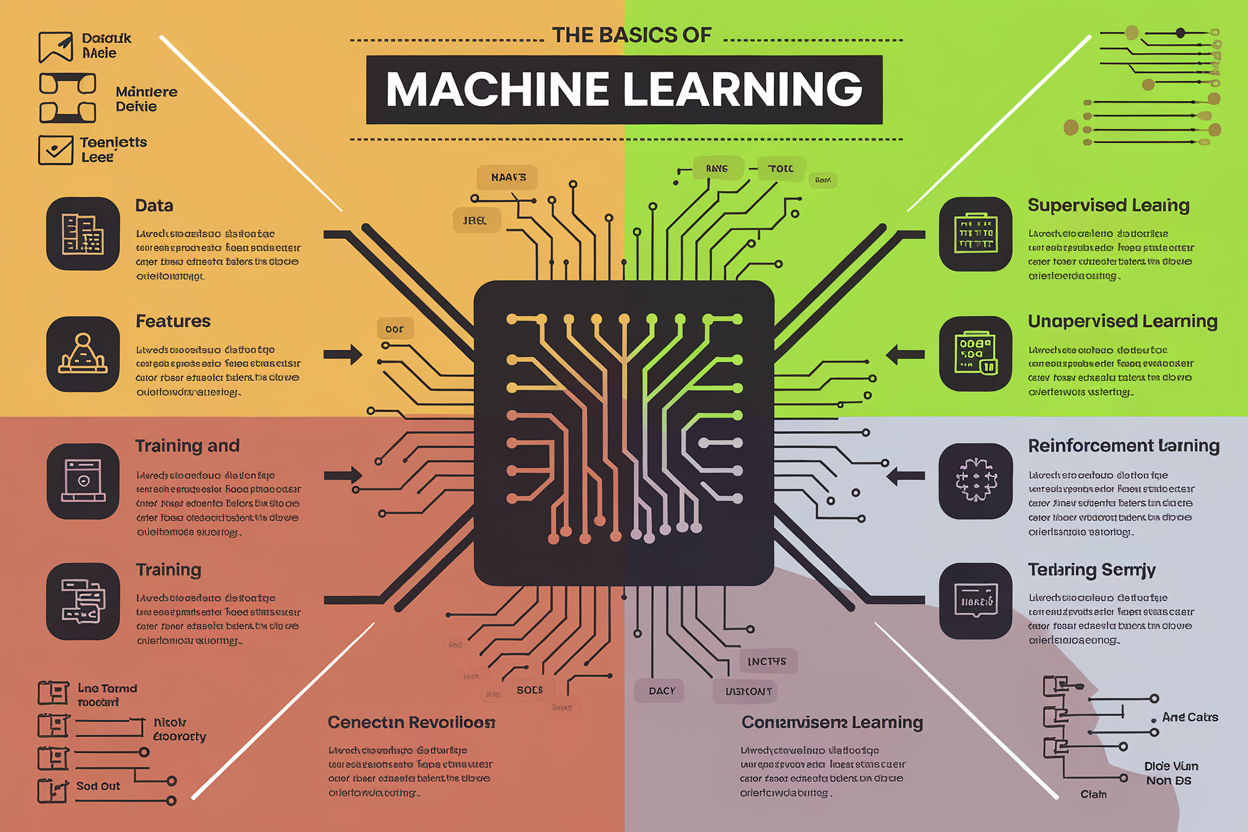
Introduction to Machine Learning
Machine learning (ML) is a subset of artificial intelligence (AI) that focuses on building systems that can learn from and make decisions based on data. Unlike traditional programming where explicit instructions are given, ML algorithms learn from patterns in data to make predictions or decisions. This post will delve into the basics of how these algorithms work, providing a solid foundation for understanding this transformative technology.
Key Concepts in Machine Learning
- Data: The raw input that algorithms use to learn and make predictions. This can be structured (like tables) or unstructured (like text and images).
- Features: Individual measurable properties or characteristics of the data. For example, in a dataset of houses, features might include size, location, and number of rooms.
- Labels: The output or target variable that the algorithm is trying to predict or classify. For instance, in a classification task, labels could be categories like 'spam' or 'not spam'.
- Training: The process where the algorithm learns from the data. During training, the algorithm adjusts its parameters to minimize errors and improve predictions.
- Testing: Evaluating the performance of the trained model using new, unseen data to assess its accuracy and generalizability.
Types of Machine Learning Algorithms
Machine learning algorithms can be categorized based on how they learn from data. The main types are:
- Supervised Learning: Algorithms learn from labeled data and make predictions based on this training. Common algorithms include Linear Regression, Logistic Regression, and Support Vector Machines (SVMs).
- Unsupervised Learning: Algorithms find patterns or structures in unlabeled data. Examples include Clustering algorithms like K-means and Dimensionality Reduction techniques like Principal Component Analysis (PCA).
- Reinforcement Learning: Algorithms learn by interacting with an environment and receiving feedback in the form of rewards or penalties. This approach is commonly used in robotics and game AI.
How Algorithms Learn: A Basic Example
Let’s consider a simple example of a supervised learning algorithm: Linear Regression. Suppose you want to predict house prices based on features like size and location. The algorithm will use historical data of house prices to learn the relationship between the features and the price. During training, it adjusts the coefficients of the features to minimize the difference between predicted and actual prices, eventually providing a model that can predict prices for new houses.
import numpy as np
from sklearn.linear_model import LinearRegression
# Example data
X = np.array([[1500, 3], [2000, 4], [2500, 5]]) # Features: [size, number of rooms]
Y = np.array([300000, 400000, 500000]) # Labels: Prices
# Initialize and train the model
model = LinearRegression()
model.fit(X, Y)
# Predict new data
new_data = np.array([[1800, 3]])
predicted_price = model.predict(new_data)
print(predicted_price) # Output: Predicted price for the new data
Challenges in Machine Learning
While machine learning holds great promise, it also comes with challenges that need to be addressed. Some common issues include:
- Data Quality: Poor quality or insufficient data can lead to inaccurate models.
- Overfitting: When a model learns too much from the training data, it may perform poorly on new data.
- Bias: Algorithms can inherit biases present in the training data, leading to unfair or inaccurate predictions.
- Complexity: Understanding and tuning algorithms can be complex, requiring a deep knowledge of both data and model parameters.
The Future of Machine Learning
Machine learning continues to evolve rapidly, with advancements in algorithms, computational power, and data availability driving new possibilities. From improving healthcare to transforming industries, the potential applications are vast. As technology progresses, machine learning will play an increasingly integral role in shaping the future.
Understanding the basics of machine learning algorithms provides a foundation for exploring more advanced topics and applications. Whether you’re a developer looking to integrate ML into your projects or simply curious about this exciting field, grasping these core concepts is the first step towards harnessing the power of data and AI.
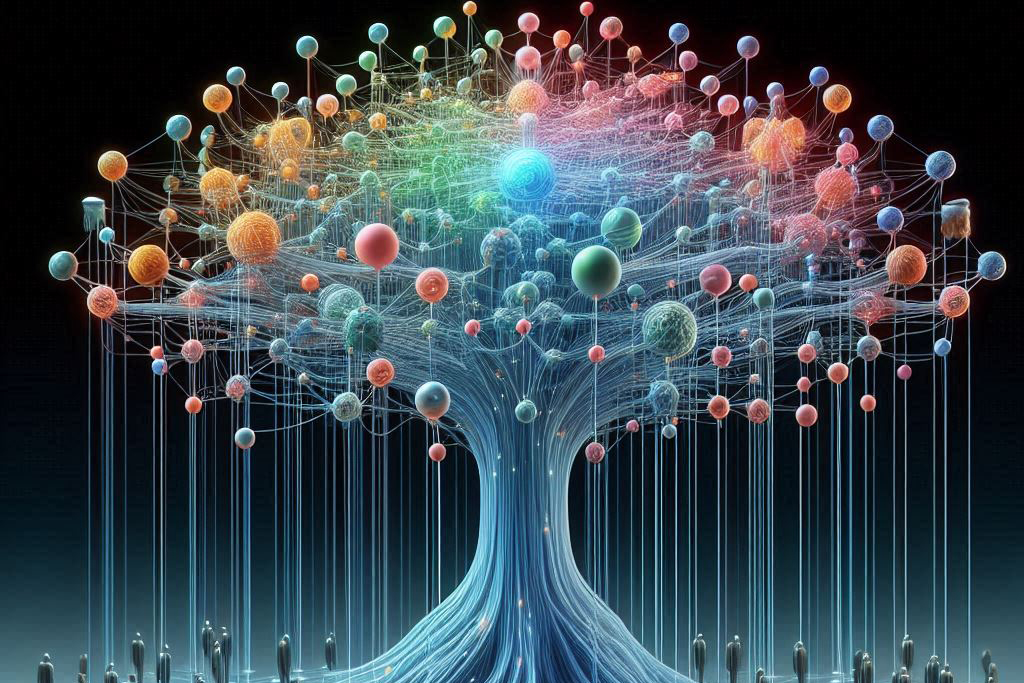
Understanding Neural Networks: The Building Blocks of Deep Learning
Dive into the fundamentals of neural networks and explore how these building blocks of deep learning drive innovations in AI.
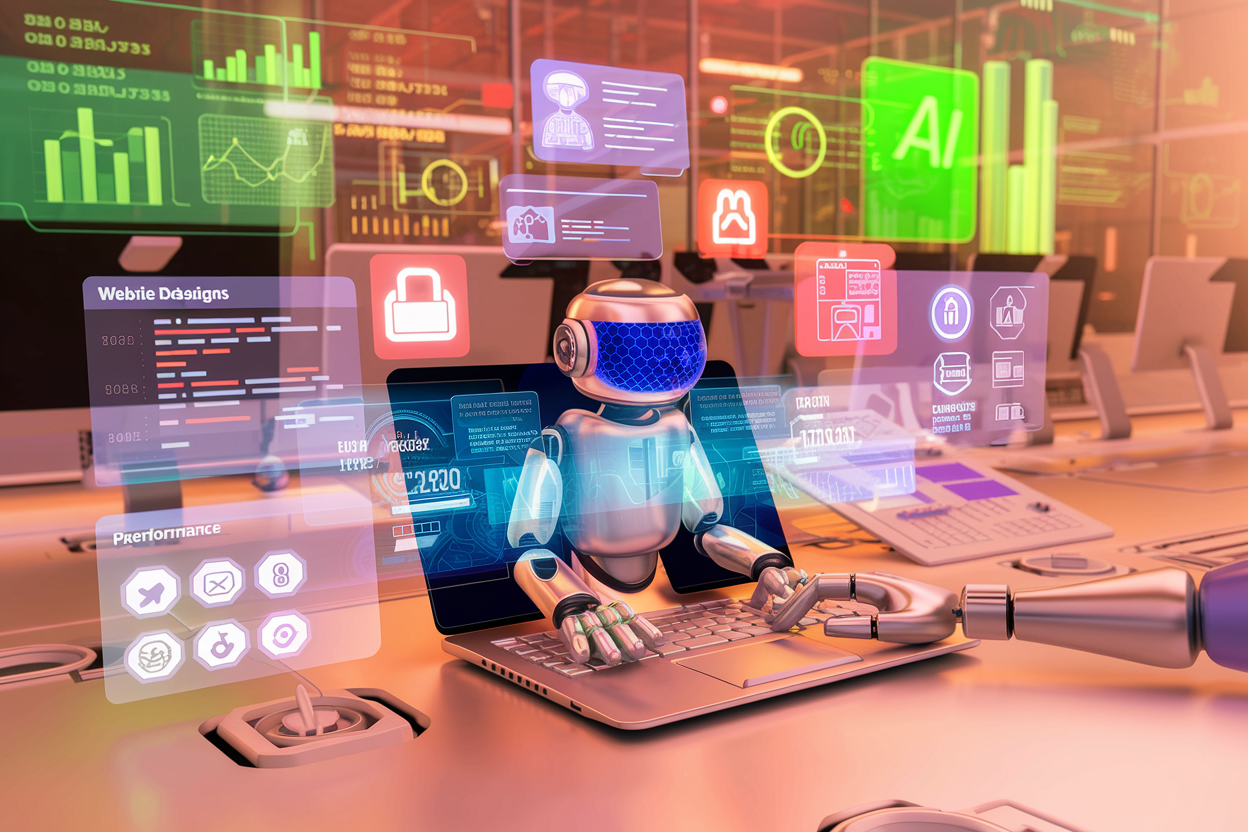
AI in Web Development: How Machine Learning is Shaping the Future of Websites
Explore how artificial intelligence and machine learning are revolutionizing web development and shaping the future of websites.
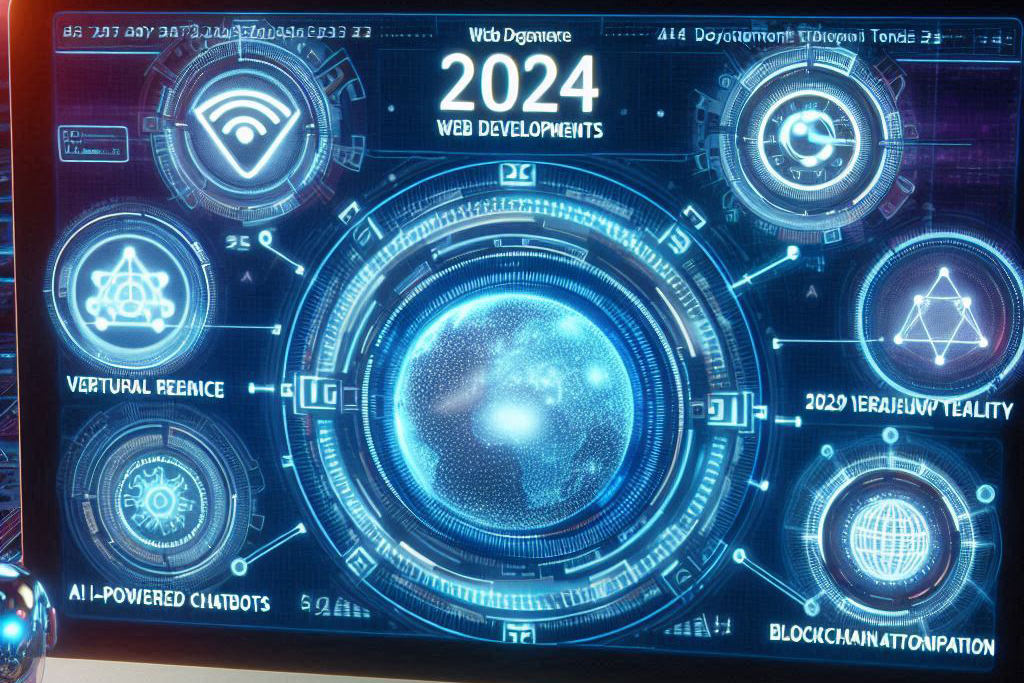
Top Web Development Trends to Watch in 2024
Stay ahead in web development by exploring the top trends of 2024, from Progressive Web Apps and AI integration to serverless architecture and motion UI.
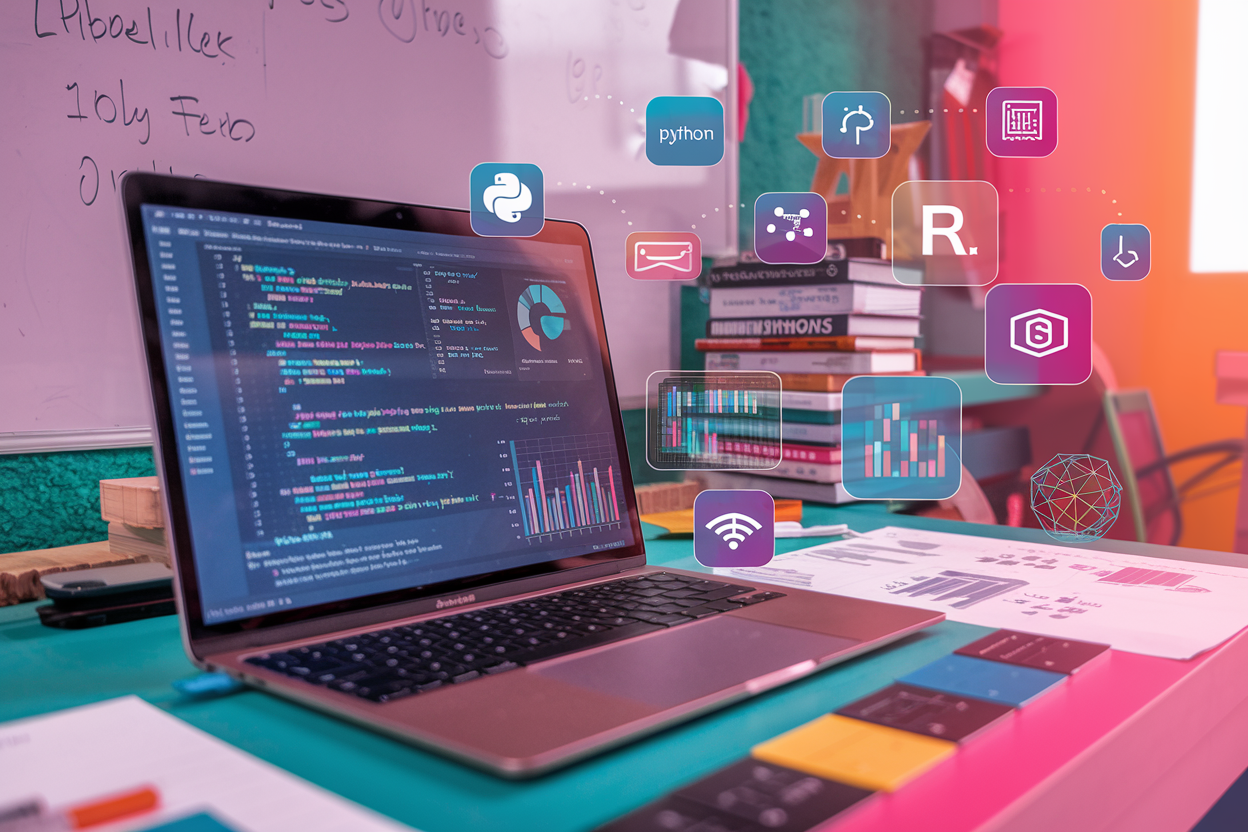
Getting Started with Machine Learning: Tools and Resources for Beginners
Discover essential tools and resources to kickstart your journey into machine learning, including programming languages, libraries, and educational platforms.