The Four Layers of Dfinity Consensus: A Breakdown for Developers
By Kainat Chaudhary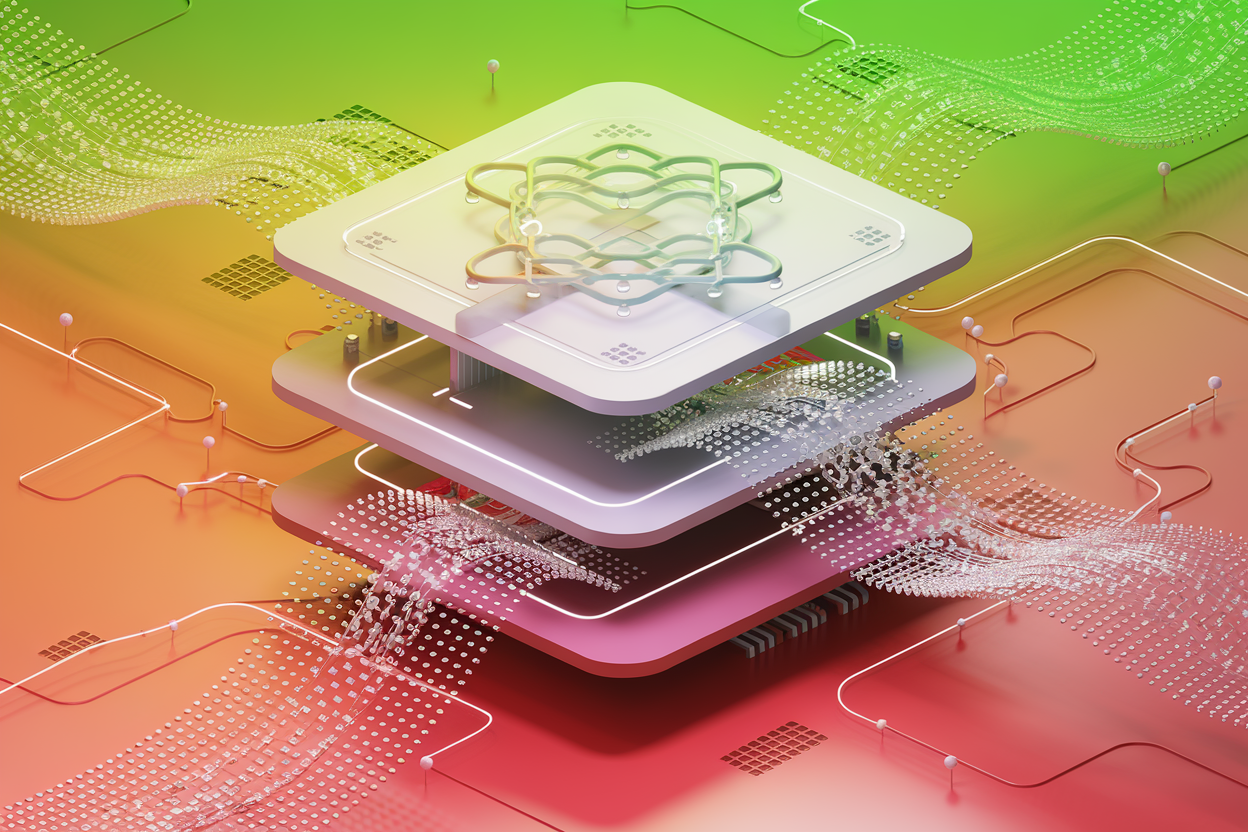
Understanding the Architectural Marvel of Dfinity Consensus
Hello, brilliant developers! Today we're diving deep into the fascinating architecture of Dfinity's consensus mechanism. This remarkable system is structured in four distinct layers, each playing a crucial role in maintaining the Internet Computer's high performance and security standards.
Layer 1: The Random Beacon Layer
The foundation of Dfinity's consensus starts with the Random Beacon layer. This layer generates unbiased, verifiable random values using threshold signatures and distributed key generation. The randomness produced here drives the entire consensus mechanism, ensuring fair participant selection and preventing manipulation.
// Example of Random Beacon interaction
class RandomBeacon {
async generateRandomness(epoch: number): Promise<Bytes> {
const shares = await collectThresholdShares(epoch);
return deriveRandomness(aggregateShares(shares));
}
}
Layer 2: The Notary Layer
- Block validation and verification
- Threshold signatures on block proposals
- Prevention of equivocation
- Network synchronization management
- Block height confirmation
- Notarisation certificate generation
The Notary layer acts as the authentication mechanism for block proposals. It employs a committee of nodes selected by the Random Beacon to validate and sign blocks. This layer ensures that only valid blocks proceed to the next stage, implementing a robust defense against malicious proposals.
Layer 3: The Blockchain Layer
The Blockchain layer manages the actual chain construction and block production. It implements sophisticated fork choice rules and ensures proper block sequencing. This layer is particularly noteworthy for its ability to maintain high throughput while ensuring consistency.
// Simplified block production process
interface BlockProduction {
async createBlock(height: number, parentHash: Hash): Block {
const transactions = await mempool.getValidatedTxs();
const randomness = await randomBeacon.getCurrentValue();
return assembleBlock(height, parentHash, transactions, randomness);
}
}
Layer 4: The Registry Layer
The Registry layer maintains the network's configuration state and manages subnet membership. It handles crucial aspects like node registration, subnet formation, and protocol parameters. This layer ensures the entire system remains adaptable and upgradeable.
The beauty of Dfinity's four-layer architecture lies in its perfect balance of security, scalability, and adaptability - each layer complementing the others in a harmonious dance of distributed consensus.
Cross-Layer Interactions
- Layer 1 → Layer 2: Random committee selection
- Layer 2 → Layer 3: Block validation and propagation
- Layer 3 → Layer 4: Configuration updates and subnet management
- Layer 4 → Layer 1: Network parameter updates for randomness generation
Performance Characteristics
The four-layer architecture achieves remarkable performance metrics: sub-second finality, high throughput (thousands of transactions per second), and excellent scalability through subnet partitioning. Each layer is optimised for minimal latency while maintaining robust security guarantees.
Developer Implications
For developers building on the Internet Computer, understanding these layers is crucial for optimising application performance. Each layer provides specific APIs and interfaces that can be leveraged for different purposes, from accessing randomness to managing subnet deployments.
// Example of developer interaction with consensus layers
interface ConsensusInterface {
async getRandomness(): Promise<Bytes>;
async submitTransaction(tx: Transaction): Promise<Receipt>;
async querySubnetConfig(): Promise<SubnetConfig>;
async monitorBlockProgress(): Promise<BlockHeight>;
}
Future Developments and Scalability
The modular nature of this four-layer architecture allows for continuous improvement and scaling. Future developments include enhanced cross-subnet communication, improved throughput mechanisms, and advanced security features, all while maintaining backward compatibility.
Conclusion
Dfinity's four-layer consensus mechanism represents a masterclass in distributed systems design. Its elegant architecture provides the perfect foundation for the next generation of decentralised applications, offering developers a powerful and flexible platform for innovation.
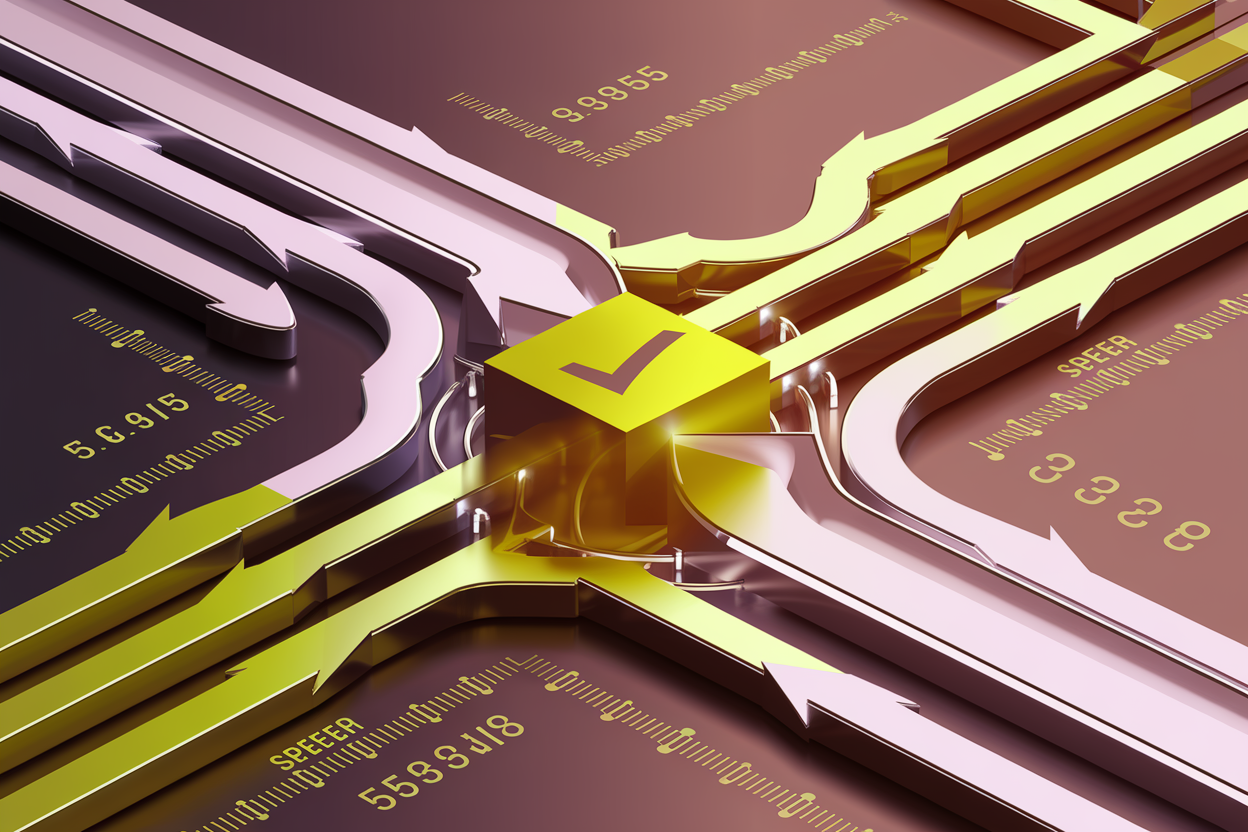
Achieving Near-Instant Finality: Dfinity's Approach to Blockchain Transactions
Explore how Dfinity's Internet Computer achieves remarkable sub-second transaction finality through innovative consensus mechanisms and sophisticated block propagation techniques
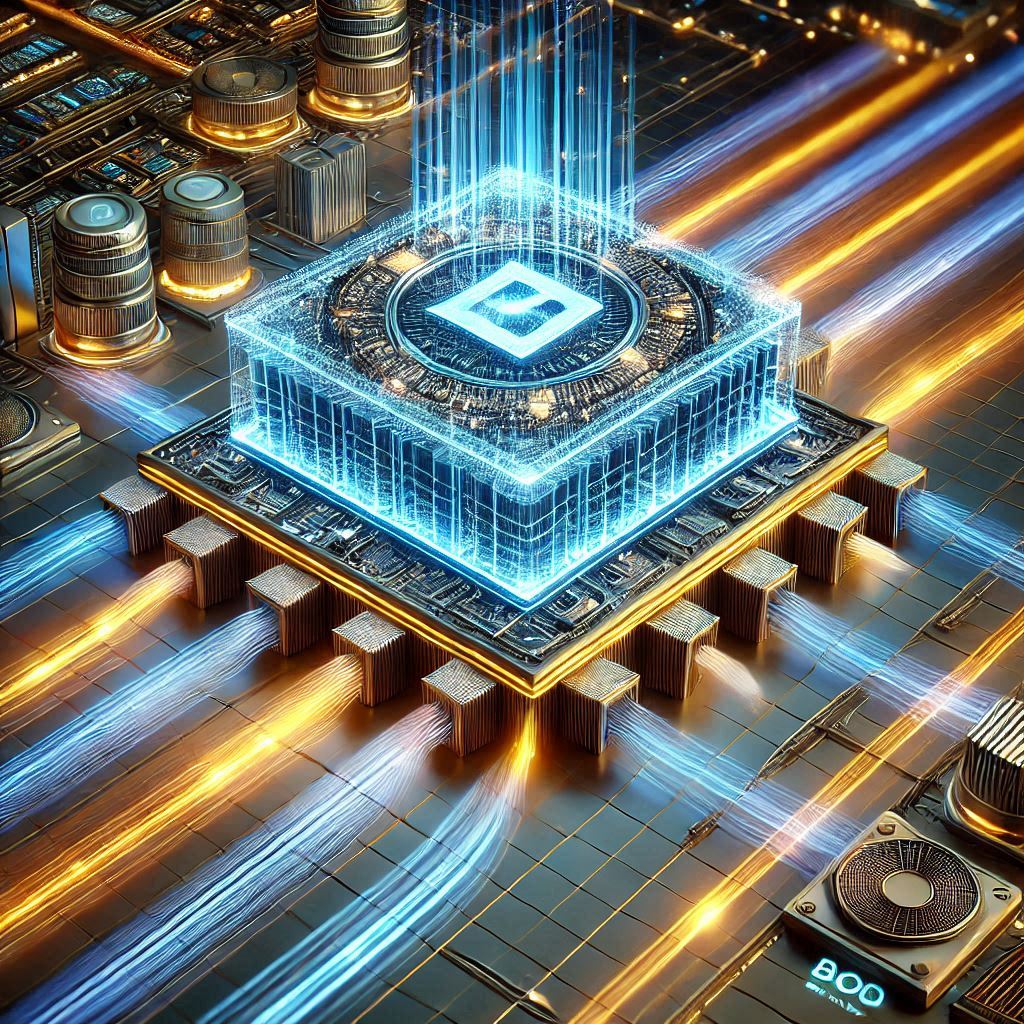
Dfinity's Blockchain Architecture: Balancing Speed, Security, and Decentralization
Discover how Dfinity's revolutionary blockchain architecture achieves the perfect equilibrium between high-speed performance, robust security, and true decentralization in the Internet Computer protocol
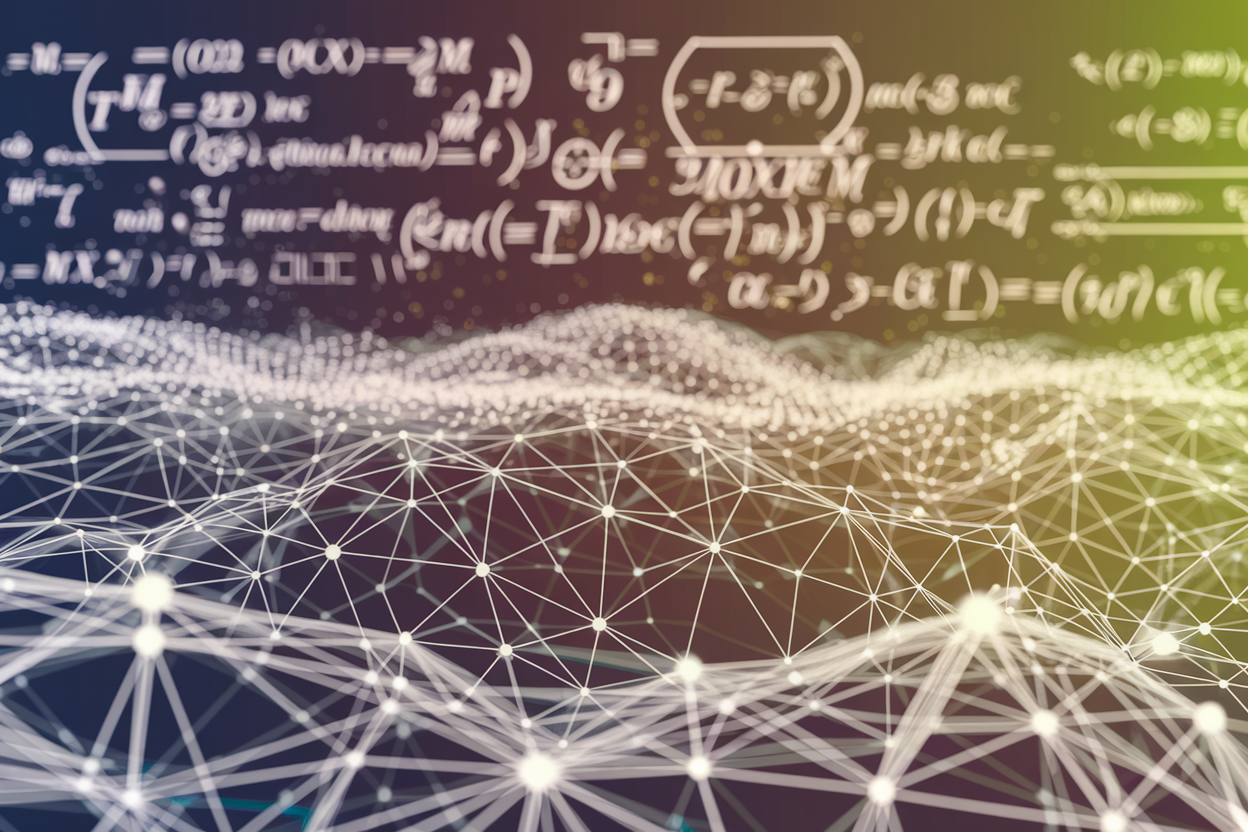
Threshold Relay and Scalability: Dfinity's Solution to Network Growth
Discover how Dfinity's innovative Threshold Relay mechanism enables unprecedented network scalability while maintaining security and performance in the Internet Computer protocol
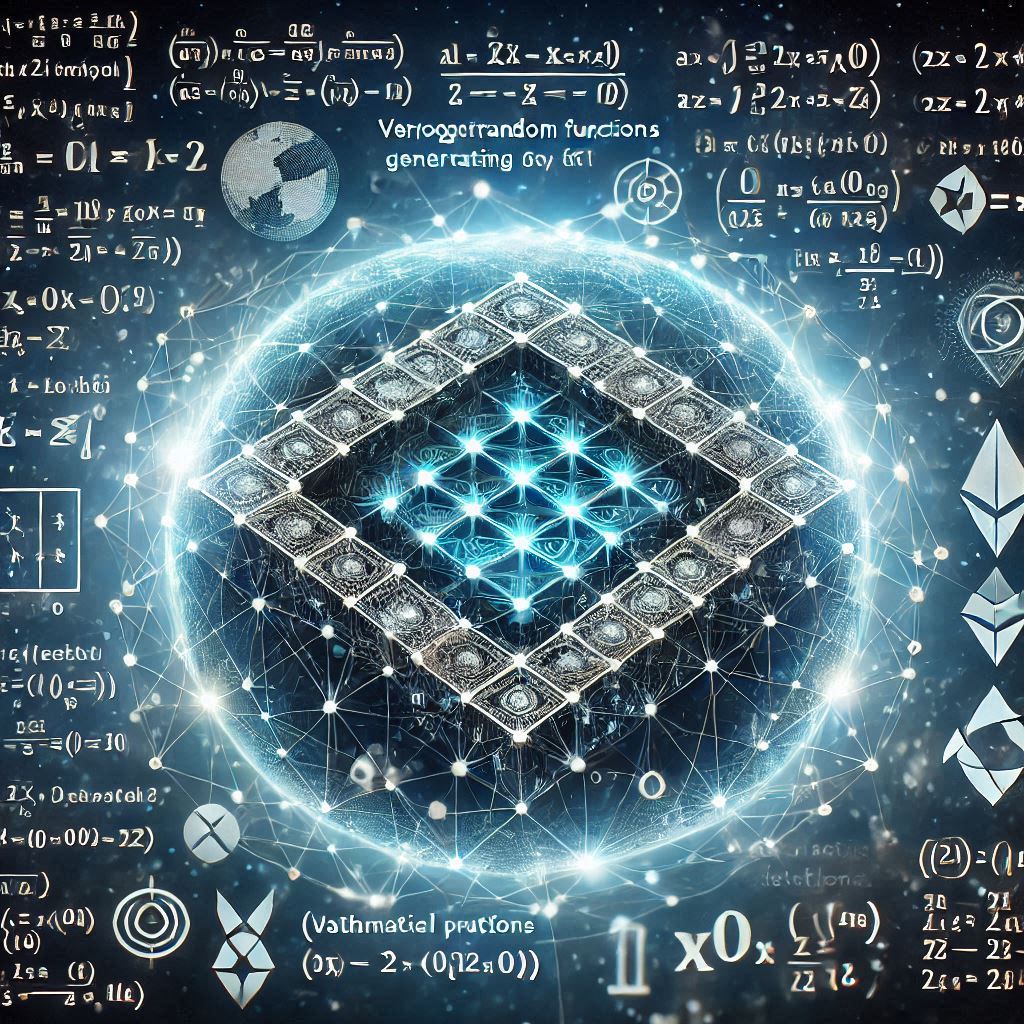
The Role of VRF in Dfinity's Blockchain and Smart Contract Ecosystem
Explore how Dfinity leverages Verifiable Random Functions (VRF) to create a secure, transparent, and efficient foundation for smart contracts and decentralised applications on the Internet Computer